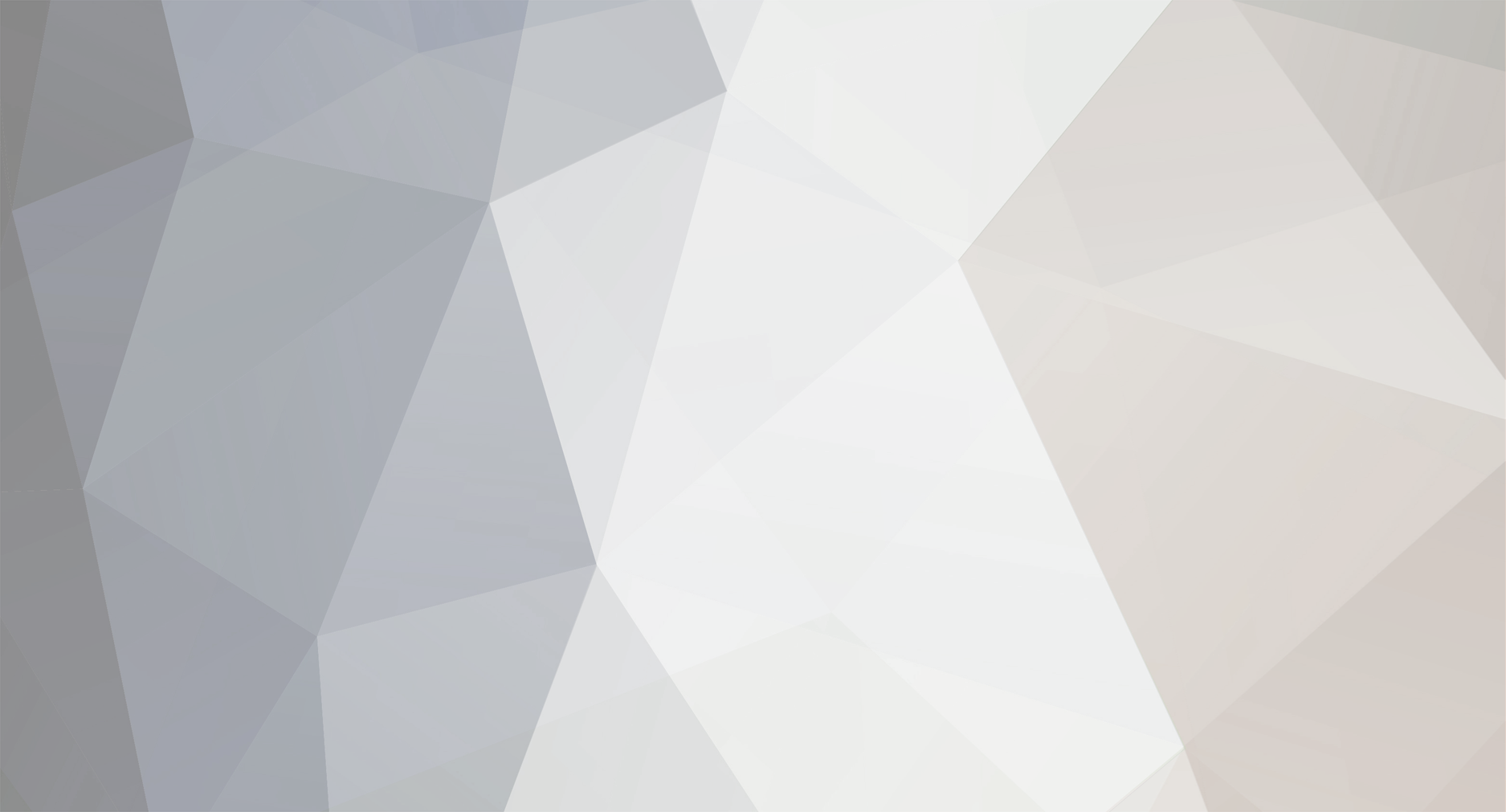
eiekland
-
Posts
1 -
Joined
-
Last visited
By using this site, you agree to our Terms of Use. Guidelines Privacy Policy We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.
FSUIPC TCAS?
in FSUIPC Support Pete Dowson Modules
Posted
Hi,
If you use VB.NET use the FSUIPC SDK and the .NET example.
Then add the following lines.
I'm sure it's cleaner way's to do it, but this was what I could come up with right now.
/Richard