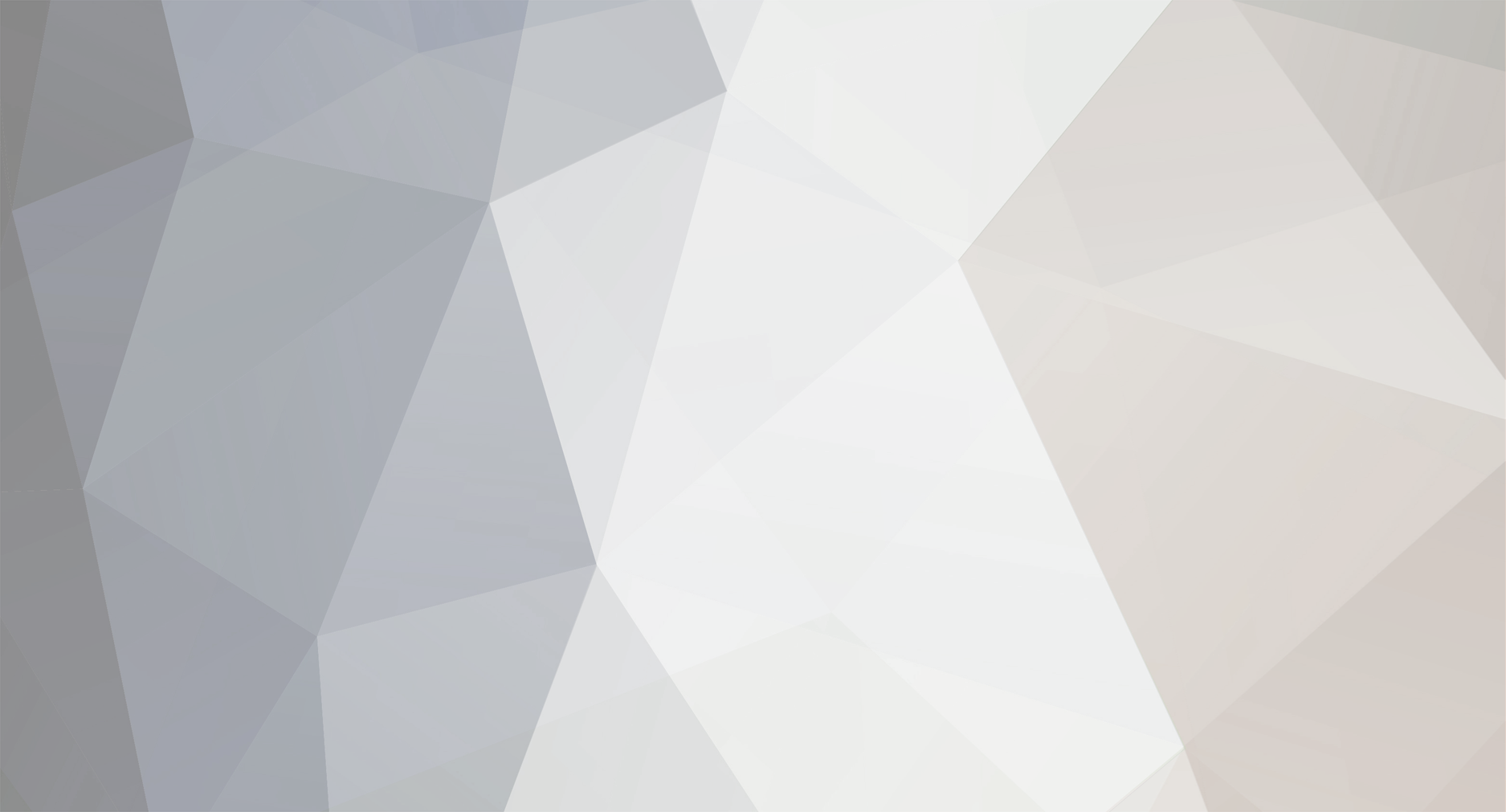
Dirk98
-
Posts
154 -
Joined
-
Last visited
-
Days Won
1
Content Type
Profiles
Forums
Events
Gallery
Downloads
Posts posted by Dirk98
-
-
I can't find this Control in FSUIPC list, is there one?
Thanks,
Dirk.
-
Any idea how to add the sound of turning knobs? All button pull / pushes tick ok.
Thanks,
Dirk.
-
Here you go, Dirk:
-- VS/FPA switch button
function switchHDGTRK (joynum, button, downup)
ipc.writeLvar( "L:AB_AP_HDGTRK", 1 - ipc.readLvar("L:AB_AP_HDGTRK") )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
-- VS/FPA Inc
function incFPAorVS ( joynum, button, downup )
if ipc.readLvar("L:AB_AP_HDGTRK") == 1 then -- 1 = TRK/FPA
local lFPA = ipc.readLvar( "L:AB_AP_FPA_Select2" ) + 0.1
if lFPA > 9.9 then
lFPA = 9.9
end
ipc.writeLvar( "L:AB_AP_FPA_Select2", lFPA )
else -- 0 = HDG/SPD so set VS
local lVS = ipc.readLvar( "L:AB_AP_VS_Select2" ) + 1
if lVS > 60 then
lVS = 60
end
ipc.writeLvar( "L:AB_AP_VS_Select2", lVS )
end
end
-- VS/FPA Dec
function decFPAorVS ( joynum, button, downup )
if ipc.readLvar("L:AB_AP_HDGTRK") == 1 then -- 1 = TRK/FPA
local lFPA = ipc.readLvar( "L:AB_AP_FPA_Select2" ) - 0.1
if lFPA < -9.9 then
lFPA = -9.9
end
ipc.writeLvar( "L:AB_AP_FPA_Select2", lFPA )
else -- 0 = HDG/SPD so set VS
local lVS = ipc.readLvar( "L:AB_AP_VS_Select2" ) - 1
if lVS < -60 then
lVS = -60
end
ipc.writeLvar( "L:AB_AP_VS_Select2", lVS )
end
end
-- VS/FPA Level
function levFPAorVS ( joynum, button, downup )
if ipc.readLvar("L:AB_AP_HDGTRK") == 1 then
ipc.writeLvar( "L:AB_AP_FPA_Select2", 0 )
else
ipc.writeLvar( "L:AB_AP_VS_Select2", 0 )
end
end
-- register the buttons of your joystick triggering the functions
-- replace joyletter by the actual used letter/number, also replace buttonX by the actual value
event.button("joyletter", button1, 1, "incFPAorVS")
event.button("joyletter", button2, 1, "decFPAorVS")
event.button("joyletter", button3, 1, "switchHDGTRK")
event.button("joyletter", button4, 1, "levFPAorV")[/CODE][color=#666600]Cheers,[/color]
[color=#666600]Dirk [/color] :D
-
I can't figure out why most of the script works ok, except for MACH Minus and MACH Minus Fast. The positivie part works both in SPD and MACH modes just fine:
-- SPD/MACH switch button
function switchSPDMACH (joynum, button, downup)
ipc.writeLvar( "L:AB_AP_SPDMACH", 1 - ipc.readLvar("L:AB_AP_SPDMACH") )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
-- SPD/MACH Inc
function incSPDorMACH ( joynum, button, downup )
if ipc.readLvar("L:AB_AP_SPDMACH") == 1 then
local lMACH = ipc.readLvar( "L:AB_AP_Mach_Select" ) + 0.01
if lMACH > 1.0 then
lMACH = 1.0
end
ipc.writeLvar( "L:AB_AP_Mach_Select", lMACH )
else
local lSPD = ipc.readLvar( "L:AB_AP_SPEED_Select" ) + 1
if lSPD > 340 then
lSPD = 340
end
ipc.writeLvar( "L:AB_AP_SPEED_Select", lSPD )
end
end
-- SPD/MACH IncFast
function incFastSPDorMACH ( joynum, button, downup )
if ipc.readLvar("L:AB_AP_SPDMACH") == 1 then
local lMACH = ipc.readLvar( "L:AB_AP_Mach_Select" ) + 0.1
if lMACH > 1.0 then
lMACH = 1.0
end
ipc.writeLvar( "L:AB_AP_Mach_Select", lMACH )
else
local lSPD = ipc.readLvar( "L:AB_AP_SPEED_Select" ) + 10
if lSPD > 340 then
lSPD = 340
end
ipc.writeLvar( "L:AB_AP_SPEED_Select", lSPD )
end
end
-- SPD/MACH Dec
function decSPDorMACH ( joynum, button, downup )
if ipc.readLvar("L:AB_AP_SMPDMACH") == 1 then
local lMACH = ipc.readLvar( "L:AB_AP_Mach_Select" ) - 0.01
if lMACH < 0 then
lMACH = 0
end
ipc.writeLvar( "L:AB_AP_Mach_Select", lMACH )
else
local lSPD = ipc.readLvar( "L:AB_AP_SPEED_Select" ) - 1
if lSPD < 0 then
lSPD = 0
end
ipc.writeLvar( "L:AB_AP_SPEED_Select", lSPD )
end
end
-- SPD/MACH DecFast
function decFastSPDorMACH ( joynum, button, downup )
if ipc.readLvar("L:AB_AP_SMPDMACH") == 1 then
local lMACH = ipc.readLvar( "L:AB_AP_Mach_Select" ) - 0.1
if lMACH < 0 then
lMACH = 0
end
ipc.writeLvar( "L:AB_AP_Mach_Select", lMACH )
else
local lSPD = ipc.readLvar( "L:AB_AP_SPEED_Select" ) - 10
if lSPD < 0 then
lSPD = 0
end
ipc.writeLvar( "L:AB_AP_SPEED_Select", lSPD )
end
end
event.button(149, 3, 1, "switchSPDMACH")
event.button(149, 22, 1, "incSPDorMACH")
event.button(149, 23, 1, "incFastSPDorMACH")
event.button(149, 21, 1, "decSPDorMACH")
event.button(149, 20, 1, "decFastSPDorMACH")[/CODE]Any idea?
Much thanks,
Dirk.
-
Is it possible to add some switching script to reset VS to 0 as well "outside" of AXE code?
Thanks,
Dirk.
Yes, it is! With the tip from Joshua Che. on aerosoft boards (ipc.writeLvar( "L:AB_AP_VS_Select2", 0 ) I produced my own little script (or should I call it "hack"?) :
-- VS/FPA Level code
function levFPAorVS ( joynum, button, downup )
if ipc.readLvar("L:AB_AP_HDGTRK") == 1 then
ipc.writeLvar( "L:AB_AP_FPA_Select2", 0 )
else
ipc.writeLvar( "L:AB_AP_VS_Select2", 0 )
end
end
event.button(175, 5, 1, "levFPAorVS")
[/CODE]See, Reinhard, your kind help and scripts do magic on me already, I feel like I can script something too, lol.
Thank you very much again,
Dirk.
-
well thefile here is not working whit AXE as they chagesed some names so it have to be redone and i have no plans of doeing that as i have stoped helping aerosoft as i got diserpointed whit this release as for me it´s even bigger problems then the privius version when it comes to how sensetive it is and crash the sim even whit out using the hardware so sorry.. i know other have the variables and perhaps you could ask them and get a awenser..
Understood. Just in case, KAPTEJNLN, here you'll find all the new names (follow the link to aerosoft SDK http://forum.aerosof...-sdk-variables/ ) and improved scripting concept for AXE (compared to what had been done for AX):
http://forum.simflig...-into-one-file/
Cheers,
Dirk.
-
Just in case somebody needs current AXE LVars, the latest list is available here:
http://forum.aerosoft.com/index.php?/topic/64255-airbus-x-extended-sdk-variables/
Cheers,
Dirk.
-
I'll post soon my LUA for AXE, that basically can be used with any HID device, all interested please praise Reinhard (and Pete of course).
Question tor Reinhard:
I posted about this issue with AB_AP_VSlevel on aerosoft/axe/sdk forum. I'm not sure though I was right claiming it was an error or an omission of the current AXE SDK:
"AP_AP_VSlevel" actually resets FPA but not V/S to "0". It does not affect V/SPlease revise or explain how it should be handled.
You can easily check this out with this lua:
Quote
if ipcPARAM == 52 thenLVarSet = "L:AB_AP_VSlevel"LVarGet = ipc.readLvar(LVarSet)ipc.writeLvar(LVarSet, 1)endTry to change VS and use the above function, you'll see that nothing happens. Then switch HDGVS to TRKFPA, change FPA to some +/- and run the above again -> you'll see FPA will reset to +0.0.
I haven't found "AP_AP_FPAlevel" in SDK, so the above may well be a wrongly assigned function, with VS reset logic missing at all.
PS: I've just noticed in HDGVS mode the knob actually moves when you push VS knob with "L:AB_AP_VSlevel", but doesn't reset. In TRKFPA it also moves and resets FPA.
See, AB_AP_VSlevel resets only TRK/FPA to "0", when FCU is in TRK/FPA mode. It does nothing for HDG/VS mode though. I currently use this code:
-- VS/FPA level code
function levelVSlevel (joynum, button, downup)
ipc.writeLvar( "L:AB_AP_VSlevel", 1 - ipc.readLvar("L:AB_AP_VSlevel") )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
event.button(175, 5, 1, "levelVSlevel")[/CODE]Is it possible to add some switching script to reset VS to 0 as well "outside" of AXE code?
Thanks,
Dirk.
-
The HDG knob turning code works perfect without "end if". You made my day, Reinhard, thank you very much.
I'm working on the VS/FPA knob and button script now, there are some parts missing yet.
Dirk.
-
Haha! This code now increases VS by 100 or FPA by 0.1 each time I press the assigned button, depending on which state the VS/FPA button is in (not in the code yet, I do it manually). I think I can start building up on it.
-- VS/FPA Toggle code
function incFPAorVS ( joynum, button, downup )
if ipc.readLvar("L:AB_AP_HDGTRK") == 1 then -- 1 = TRK/FPA
local lFPA = ipc.readLvar( "L:AB_AP_FPA_Select2" ) + 0.1
if lFPA > 9.9 then
lFPA = 9.9
end
ipc.writeLvar( "L:AB_AP_FPA_Select2", lFPA )
else -- 0 = HDG/SPD so set VS
local lVS = ipc.readLvar( "L:AB_AP_VS_Select2" ) + 1
if lVS > 60 then
lVS = 60
end
ipc.writeLvar( "L:AB_AP_VS_Select2", lVS )
end
end
event.button(175, 4, 1, "incFPAorVS")[/CODE]Will check the HDG inc/dec code without "end if" now.
Thanks!
Dirk.
-
Hi,
I found it: Replace the "end if" statements by a simple "end".
That should fix it.
Rgds
Reinhard
Ooops, in which one of the two luas? :D
Thanks,
Dirk.
-
let's try something like this:
function incdecHDG ( pDiff ) -- increment or decrement HDG by pDiff
local lHDG = ipc.readLvar( "L:AB_AP_HDG_Select" ) + pDiff
if lHDG > 359 then
lHDG = lHDG - 360
end if
if lHDG < 0 then
lHDG = lHDG + 360
end if
ipc.writeLvar( "L:AB_AP_HDG_Select", lHDG )
end
function incHDG ( joynum, button, downup )
incdecHDG ( 1 )
end
function decHDG ( joynum, button, downup )
incdecHDG ( -1 )
end
function incHDGFast ( joynum, button, downup )
incdecHDG ( 10 )
end
function decHDGFast ( joynum, button, downup )
incdecHDG ( -10 )
end
-- register the buttons of your joystick triggering the functions
-- replace joynum by the actual used number, also replace button by the actual button number
event.button(joynum, button1, 1, "incHDG")
event.button(joynum, button2, 1, "decHDG")
event.button(joynum, button3, 1, "incHDGFast")
event.button(joynum, button4, 1, "decHDGFast")
[/CODE]Unfortunately this one doesn't work neither:
Here's my current lua:
[CODE]
-- toggle FD button
function toggleFD (joynum, button, downup)
ipc.writeLvar( "L:AB_MPL_FD", 1 - ipc.readLvar("L:AB_MPL_FD") )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
-- toggle ATHR button
function toggleILS (joynum, button, downup)
ipc.writeLvar( "L:AB_MPL_ILS", 1 - ipc.readLvar("L:AB_MPL_ILS") )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
-- HDG mode selection managed
function selectHDGmode (joynum, button, downup)
ipc.writeLvar( "L:AB_AP_HDGmode", 1 )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
-- HDG Knob
function incdecHDG ( pDiff ) -- increment or decrement HDG by pDiff
local lHDG = ipc.readLvar( "L:AB_AP_HDG_Select" ) + pDiff
if lHDG > 359 then
lHDG = lHDG - 360
end if
if lHDG < 0 then
lHDG = lHDG + 360
end if
ipc.writeLvar( "L:AB_AP_HDG_Select", lHDG )
end
function incHDG ( joynum, button, downup )
incdecHDG ( 1 )
end
function decHDG ( joynum, button, downup )
incdecHDG ( -1 )
end
function incHDGFast ( joynum, button, downup )
incdecHDG ( 10 )
end
function decHDGFast ( joynum, button, downup )
incdecHDG ( -10 )
end
event.button(175, 0, 1, "toggleFD")
event.button(175, 1, 1, "toggleILS")
event.button(149, 1, 1, "selectHDGmode")
event.button(149, 18, 1, "incHDG")
event.button(149, 17, 1, "decHDG")
event.button(149, 19, 1, "incHDGFast")
event.button(149, 16, 1, "decHDGFast")
[/CODE]None of the functions work when I add the HDG knob turning part.
Thanks,
Dirk.
-
Let's try something like this:
function incFPAorVS ( joynum, button, downup )
if ipc.readLvar("L:AB_AP_HDGTRK") == 1 then -- 1 = TRK/FPA
local lFPA = ipc.readLvar( "L:AB_AP_FPA_Select2" ) + 0.1
if lFPA > 9.9 then
lFPA = 9.9
end if
ipc.writeLvar( "L:AB_AP_FPA_Select2", lFPA )
else -- 0 = HDG/SPD so set VS
local lVS = ipc.readLvar( "L:AB_AP_VS_Select2" ) + 1
if lVS > 60 then
lVS = 60
end if
ipc.writeLvar( "L:AB_AP_VS_Select2", lVS )
end
end
-- register the buttons of your joystick triggering other functions
-- replace joynum by the actual used number, also replace button by the actual button number
event.button(joynum, button, 1, "incFPAorVS")
[/CODE]Not sure if it works
No, unfortunately it does not. The current lua looks as follows and[u][b] none of the functions work[/b][/u]:
[CODE]
-- toggle FD button
function toggleFD (joynum, button, downup)
ipc.writeLvar( "L:AB_MPL_FD", 1 - ipc.readLvar("L:AB_MPL_FD") )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
-- toggle ATHR button
function toggleILS (joynum, button, downup)
ipc.writeLvar( "L:AB_MPL_ILS", 1 - ipc.readLvar("L:AB_MPL_ILS") )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
-- HDG mode selection managed
function selectHDGmode (joynum, button, downup)
ipc.writeLvar( "L:AB_AP_HDGmode", 1 )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
-- VS/FPA Toggle code
function incFPAorVS ( joynum, button, downup )
if ipc.readLvar("L:AB_AP_HDGTRK") == 1 then -- 1 = TRK/FPA
local lFPA = ipc.readLvar( "L:AB_AP_FPA_Select2" ) + 0.1
if lFPA > 9.9 then
lFPA = 9.9
end if
ipc.writeLvar( "L:AB_AP_FPA_Select2", lFPA )
else -- 0 = HDG/SPD so set VS
local lVS = ipc.readLvar( "L:AB_AP_VS_Select2" ) + 1
if lVS > 60 then
lVS = 60
end if
ipc.writeLvar( "L:AB_AP_VS_Select2", lVS )
end
end
event.button(175, 4, 1, "incFPAorVS")
event.button(175, 0, 1, "toggleFD")
event.button(175, 1, 1, "toggleILS")
event.button(149, 1, 1, "selectHDGmode")[/CODE]However when I change back to:
[CODE]
-- toggle FD button
function toggleFD (joynum, button, downup)
ipc.writeLvar( "L:AB_MPL_FD", 1 - ipc.readLvar("L:AB_MPL_FD") )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
-- toggle ATHR button
function toggleILS (joynum, button, downup)
ipc.writeLvar( "L:AB_MPL_ILS", 1 - ipc.readLvar("L:AB_MPL_ILS") )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
-- HDG mode selection managed
function selectHDGmode (joynum, button, downup)
ipc.writeLvar( "L:AB_AP_HDGmode", 1 )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
event.button(175, 0, 1, "toggleFD")
event.button(175, 1, 1, "toggleILS")
event.button(149, 1, 1, "selectHDGmode")[/CODE]the above functions work fine again.
Thanks,
Dirk.
PS: Also, in this script for VS/FPA switching I don't see event buttons for VS incr/decr and FPA incr/decr, even though you added ipc.writeLvar( "L:AB_AP_FPA_Select2", lFPA ) and ipc.writeLvar( "L:AB_AP_VS_Select2", lVS ) functionality (I mean turning the VS/FPA knob) if I got it right.
For your reference the previous 2 LUAs for turning VS +/- looked like this:
-- VS Dec
LVarSet = "L:AB_AP_VS_Select2"
LVarGet = ipc.readLvar(LVarSet)
ipc.writeLvar(LVarSet, LVarGet-1)
-- VS Inc
LVarSet = "L:AB_AP_VS_Select2"
LVarGet = ipc.readLvar(LVarSet)
ipc.writeLvar(LVarSet, LVarGet+1)
otherwise they were combined in one big LUA as follows:
-- V/S inc
if ipcPARAM == 53 then
LVarSet = "L:AB_AP_VS_Select2"
LVarGet = ipc.readLvar(LVarSet)
ipc.writeLvar(LVarSet, LVarGet+1)
end
-- V/S dec
if ipcPARAM == 54 then
LVarSet = "L:AB_AP_VS_Select2"
LVarGet = ipc.readLvar(LVarSet)
ipc.writeLvar(LVarSet, LVarGet-1)
end
-- 56 FPA inc
if ipcPARAM == 56 then
LVarSet = "L:AB_AP_FPA_Select2"
LVarGet = ipc.readLvar(LVarSet)
ipc.writeLvar(LVarSet, LVarGet+0.1)
end
-- 57 FPA dec
if ipcPARAM == 57 then
LVarSet = "L:AB_AP_FPA_Select2"
LVarGet = ipc.readLvar(LVarSet)
ipc.writeLvar(LVarSet, LVarGet-0.1)
end
Thanks.
-
Woo-hooo, Reinhard! You are the King! (lua king) Thank you very much for your time spent on this. Can't wait to work on your script, dig hard into it and try out on the hardware. I have a dozen+ of GF panels, VRinsight MS Panel, (VRI FCU 2 Airbus is in the pipe) and many more that I don't use, lol. My interest atm mainly revolves around GF (MCP, RP48, GF166A VRP, GF-LGT II) and some others. Guys with GoFlight panels, if you've found this, ask me!
I'll post my results for the latest scripts.
Thanks again,
Dirk.
-
I've added 'Button On' case for Managed Heading mode selection to add to the "combined" event-driven lua:
-- toggle FD button
function toggleFD (joynum, button, downup)
ipc.writeLvar( "L:AB_MPL_FD", 1 - ipc.readLvar("L:AB_MPL_FD") )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
-- toggle ATHR button
function toggleILS (joynum, button, downup)
ipc.writeLvar( "L:AB_MPL_ILS", 1 - ipc.readLvar("L:AB_MPL_ILS") )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
-- HDG mode selection managed
function selectHDGmode (joynum, button, downup)
ipc.writeLvar( "L:AB_AP_HDGmode", 1 )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
-- register the buttons of your joystick triggering the functions
-- replace joyletter by the actual used letter/number, also replace buttonX by the actual value
event.button(175, 0, 1, "toggleFD")
event.button(175, 1, 1, "toggleILS")
event.button(149, 1, 1, "selectHDGmode")
[/CODE]Another couple of examples more from kind Lua gurus like Reinhard and I believe everyone will be able to map their HID devices to AXE functions with its SDK http://forum.aerosof...attach_id=38337. For me it's just a matter of seeing similarities and patterns in the code without understanding it, unfortunately. Lua manual and Lua Library are really beyond my depth, or too much time to study.
As I see it there are 2 more templates needed:
[CODE]
-- Heading inc fast
if ipcPARAM == 25 then
LVarSet = "L:AB_AP_HDG_Select"
LVarGet = ipc.readLvar(LVarSet)
ipc.writeLvar(LVarSet, LVarGet+10)
end[/CODE]and
[CODE]
-- 55 HDGVS/TRKFPA button toggle
if ipcPARAM == 55 then
LVarSet = "L:AB_AP_HDGTRK"
LVarGet = ipc.readLvar(LVarSet)
val = 1
if ipc.readLvar(LVarSet) == 1 then
val = 0
end
ipc.writeLvar(LVarSet, val)
ipc.writeLvar("L:SmallOverheadPushButtons", 1)
end[/CODE]I'll repeat, that Reinhard (aua668) kindly suggested another concept of scripting of the "combined" lua file for the access to AXE's internal variables (in this particular case), other than the one previously made by guenseli (in AX Lua script v1.2) and others that I saw. The lua based on Reinhard's method will work better than the parts that I quoted above, when you start mapping your HID devices, as
with every starting of a LUA script, all potential current running instances of the same script are killed immediately. So imagine you must for example wait in one of your script until the panel is reacting, before you trigger the next action. And if the user presses another key during the previous script is running, you might get unwanted results.
.... the event driven version is much better. Because the several events can be fired quite quickly without disturbing each other. And by utilizing the visibility of variables you can define global things for all functions.
I think I've learnt a lot already without delving into programming. Just another couple of examples is needed.
Thanks!
Dirk.
-
Hi,
You can keep AutoAssignLetters at 0 and manually assign them. For example Y for the Yoke etc.
The problems will come, when you unplug the USB and plug it to a different port. Then a different number will be assigned. But the letter will stay. Think about hundred of modules, where you have to change the number.
There are a lot of people, who learned this by pain. So it would be better to use the letters. It's all in the documentation.
Rgds
Reinhard
Yes, I've known about this problem since my first usb hubs 10+ yuears ago, therefore I've always had all usb connections fixed, photoed and written up in the notes, which takes a lot of time, of course, but still works. Now, assigning the letters in fsuipc.ini sounds much easier, thank you for the tip.
Dirk.
-
Reinhard, I badly need just one more piece of your code for control of the rotary, to substitue the following:
-- Heading dec
if ipcPARAM == 24 then
LVarSet = "L:AB_AP_HDG_Select"
LVarGet = ipc.readLvar(LVarSet)
ipc.writeLvar(LVarSet, LVarGet-1)
end
If I get another example of your method for the above, I'll have everything to start building the complete lua for all my input devices.
Thanks,
Dirk.
-
When there is no Toggle function, like when you turn HDG knob, what comes in place of toggleXXX?
Much thanks,
Dirk.
-
Cool, it works. I see I don't need to assign any buttons in FSUIPC GUI all is done in the script. In my example:
event.button(135, 0, 1, "toggleFD")
where 135 is the number given by FSUIPC to one of my GF panels, and 0 is button #1 on it.
Thanks!
Dirk.
-
I forgot one important thing: FSUIPC does not assign letters to my GoFlight panels, so I can use only joynumber for GoFlight. Do you know if "joyletter" in your code can be substituted by "joynumber" or smth similar?
Thanks,
Sabre10
-
Reinhard, thanks, I'll try it now. If it works I'll be able to build up more on this example and some hacking.
I know what you mean by using letters instead of numbers for the joysticks. At some point I used AutoAssignLetters=1 in FSUIPC.ini to name my joysticks by letters. But I did not understand the benefit, so I rolled back to Numbers. I have no obvious problems with numbering the joystics, are there any benefits with letters?
Thanks,
Dirk.
-
Hi,
If you combine a lot of such functions in one LUA file, which you then trigger by several buttons just by changing the paramater, you will run into a problem, if you have some more time consuming statements included in several branches. Why? You will find in the documentation, that with every starting of a LUA script, all potential current running instances of the same script are killed immediately. So imagine you must for example wait in one of your script until the panel is reacting, before you trigger the next action. And if the user presses another key during the previous script is running, you might get unwanted results.
When I started to implement some LUA scripts for buttons I first came also to your solution. But believe me, the event driven version is much better. Because the several events can be fired quite quickly without disturbing each other. And by utilizing the visibility of variables you can define global things for all functions.
Rgds
Reinhard
Reinhard, thank you very much. I think I understand what you're saying regarding the event driven version. Good methods of doing things are always very important, of course. So I'll play with your code to make it work with a concrete hardware button or a knob, then I'll be able to switch completely to your version. I'm still missing how this part should look in a concrecte code:
"joynum, button, downup".
Could you give me a concrete example please, maybe from one of your lua's.
Much thanks,
Dirk.
-
Reinhard, maybe you can figure this out:
In AXE SDK among others there are 3 specific LVars for V/S - FPA knob, that are:
AB_AP_VS_Select2
AB_AP_HDGTRK
AB_AP_FPA_Select2
HDGTRK button switches mode between VS_Select2 and FPA_Select2 modes. I'm not sure if this can somehow be merged so that one V/S hardware wheel (or knob) could understand and control both VS or FPA, depending on the mode changed by HDGTRK button. What I mean is that now I can only map my hardware VS wheel either to VS_Select2 or FPA_Select2. Obviously pressing HDGTRK button immediately loses control of the currently mapped function (VS_Select2 or FPA_Select2 depending on the script used). So, how to make the script explain and hand over control of the next function (VS or FPA code) to my hardware wheel each time HDGTRK is pressed?
Thanks.
Dirk.
-
-- toggle FD button
function toggleFD (joynum, button, downup)
ipc.writeLvar( "L:AB_MPL_FD", 1 - ipc.readLvar("L:AB_MPL_FD") )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
-- toggle ATHR button
function toggleATHR (joynum, button, downup)
ipc.writeLvar( "L:AB_AP_ATHR", 1 - ipc.readLvar("L:AB_AP_ATHR") )
ipc.writeLvar( "L:SmallOverheadPushButtons", 1 )
end
-- register the buttons of your joystick triggering the functions
-- replace joyletter by the actual used letter/number, also replace buttonX by the actual value
event.button("joyletter", button1, 1, "toggleFD")
event.button("joyletter", button2, 1, "toggleATHR")
[/CODE]Rgds
Reinhard
Very interesting. I ended up for the same with this code:
[CODE]
-- F/D button toggle
if ipcPARAM == 62 then
LVarSet = "L:AB_MPL_FD"
val = 0
if ipc.readLvar(LVarSet) == 0 then
val = 1
end
ipc.writeLvar(LVarSet, val)
ipc.writeLvar("L:SmallOverheadPushButtons", 1)
end
-- ATHR button toggle
if ipcPARAM == 9 then
LVarSet = "L:AB_AP_ATHR"
val = 0
if ipc.readLvar(LVarSet) == 0 then
val = 1
end
ipc.writeLvar(LVarSet, val)
ipc.writeLvar("L:SmallOverheadPushButtons", 1)
end
[/CODE]So in FSUIPC interface I just have to select the corresponding lua item and add parameters 62 and 9 where necessary.
I used script v1.2 by guenseli, I understood how it works and then I added and modified more code based on the latest AXE SDK by Aerosoft, for example its V/S logic.
However I'm interested in your method as well. "joynum, button" look clear, I think I know what to put in those fields, for example: J#3.B#12" But how do I edit "downup" field? (I'm talking about editing the code, not selectiing items in FSUIPC interface).
Thanks,
Sabre.
How to zap AI?
in FSUIPC Support Pete Dowson Modules
Posted
"AI zapper" would be easier to find :P
Thanks, Pete!
Dirk.