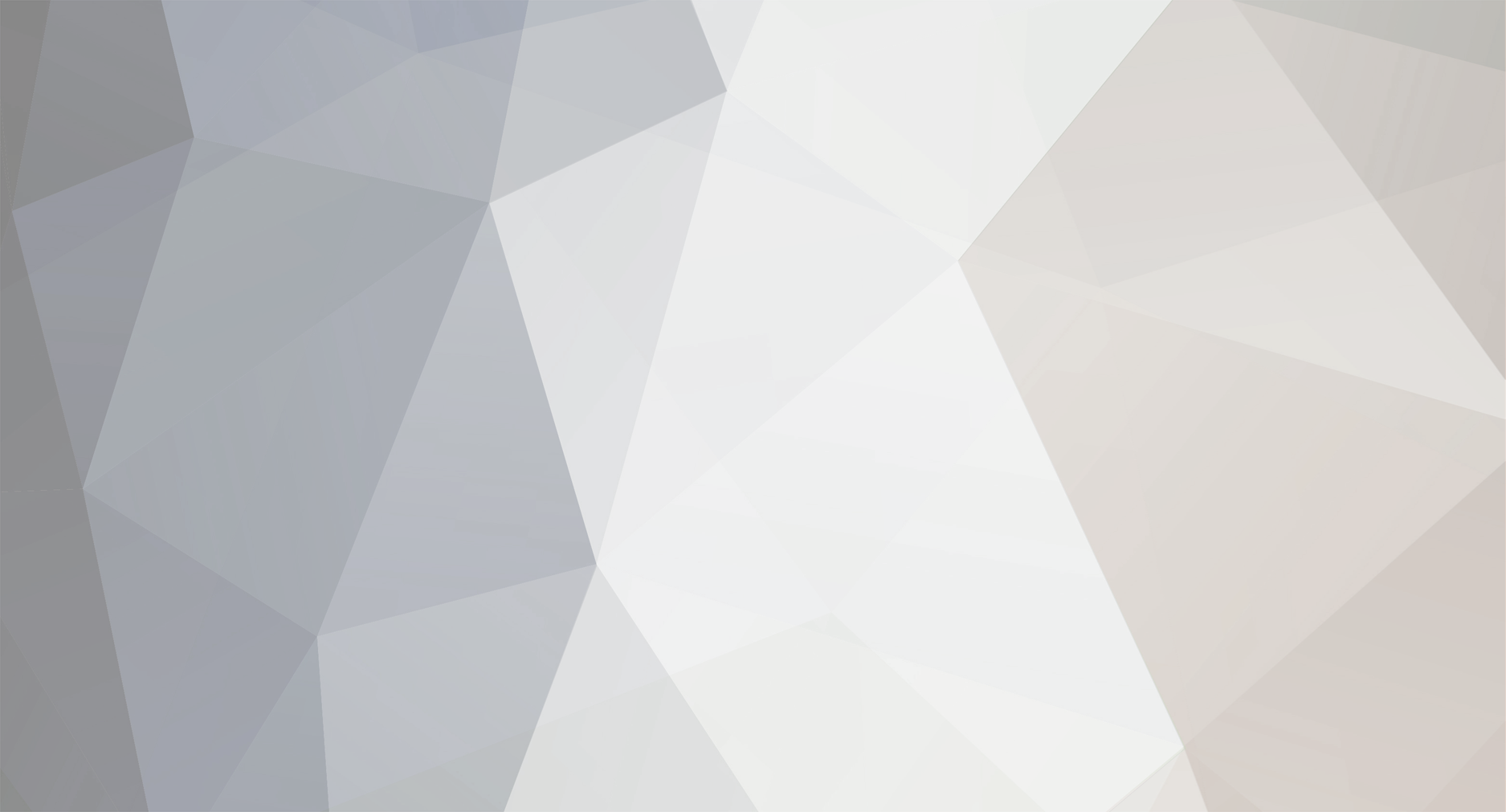
Paul Henty
-
Posts
1,657 -
Joined
-
Days Won
74
Content Type
Profiles
Forums
Events
Gallery
Downloads
Posts posted by Paul Henty
-
-
Hi Andy,
The only thing that looks out of place to me is in the processTimer.Tick() function:
At the start you have processTimer.start() and right at the end you have processTimer.Stop(). That's last line will mean the timer only ticks once and then stops. I've added comments below with !!!.
// Start processing data. #region processTimer.Tick += (s, e) => { processTimer.Start(); // !!! Not required - it's already running. try { FSUIPCConnection.Process(); var counter = activityCounter.Value; } catch (FSUIPCException ex) { /* * Since the connection to MSFS failed, stop the process timer and restart * the connection timer. We don't want to continue processing if * the connection is closed. We also don't want the connection timer running at * the same time as the process timer. It could cause connection problems, and needlessly fill the logs with endless loops of * errors. */ processTimer.Stop(); connectionTimer.Start(); logger.Warn($"Connection to MSFS closed: ({ex.FSUIPCErrorCode} - {ex.Message})."); } processTimer.Stop(); // !!! This will stop the timer after the first tick. };
Paul
-
There are no features in John's WAPI dll that are specifically for BVars, so the C# client doesn't have them either.
You might be able to trigger them with Calculator Code which can be sent via MSFSVariableServices.ExecuteCalculatorCode().
I can't tell you the syntax for this as I don't know calculator code, but you can probably find it elsewhere or ask John in the main MSFS (FSUIPC7) support forum.
Paul
-
Yes, no problem, I've now added .net 8 support starting from version 3.3,10 - available now on Nuget.
Paul
-
FSUIPC uses the Win32 API to communicate with the client applications. It's therefore 100% reliant on the internals of the Windows operating system, so that dependency cannot be removed.
While you can install XPUIPC into XPlane running on a Mac or Linux, this will only allow communication with their XPWideClient software on separate (Windows) machine. This communication will be using their own protocol based on TCP/IP. There is no way the FSUIPC protocol can be used on MacOS or Linux.
Paul
-
Yes, you can get the latest version on NuGet - it's called FSUIPCClientDLL. It targets all versions up to .NET7.
Full details are on the website:
Paul
-
Quote
After getting to the welcome screen, it still reported 0, even though IsOpen reported true.
IsOpen is true because it's connected to FSUIPC7. The counter is probably doesn't update until the player has loaded an aircraft. It should start counting up when you start a flight.
QuoteNow, when I close MSFS, the display in my app still shows 219/connected for the current status.
This seems correct then. If it's sticking at 219 then there is no activity. The ValueChanged property on 0x337E will be false. So your app knows that MSFS has been unloaded. The activity counter might also stop when the user has ended the flight and is back in the menus.
Paul
-
Quote
. However, closing MSFS while the app is open still results in the connected message staying on scree
You need to be checking the ValueChanged property of offset 0x337E. If you close MSFS and this offset is still changing (counting up), you'll need to ask John Dowson in the FSUIPC7 support forum if he has any other ideas for detecting if MSFS is unloaded.
Paul
-
Hi Andy,
The IsOpen property refers only to the FSUIPC connection, not the flight sim. Prior to FSUIPC7 this was effectively the same thing as FSUIPC ran inside the flight sim.
WideClient.exe and FSUIPC7 run outside of the flight sim, so the connection will be open if they are running, even if the flight sim is not.
If you want to know if the flight sim is open you can try polling offset 0x337E (activity counter) to make sure it's changing. See the notes in the documentation as there are times it might not update (e.g. loading new aircraft).
Paul
-
These module are only for reading and writing LVars and HVars with the MSFS flight sim. They are not for any other sim (e.g. P3D) and cannot be used for reading/writing offsets.
If you're using MSFS and the aircraft you are using provides LVars for the data you need (e.g. flaps position) you could rewrite your app to use LVARs. You will need to use the MSFSVariableServices class in my DLL.
There is more info and an example project here:
http://fsuipc.paulhenty.com/#downloads
The example project will let you read LVars so you can check that you can get all the data you need before you start coding.
(Note that the WASM module is installed with FSUIPC7. The FSUIPC_WAPID.dll needs to be downloaded and placed in your project. It should be set to be copied to the output folder when the project builds.
Paul
-
1 hour ago, Metall4You said:
How can I establish a FSUIPC connection without WideClient?
The only way without WideClient is to run the app on the same PC as the simulator.
Paul
-
Hi Ramon,
It doesn't look like focus is the problem then.
I afraid I can't suggest anything else. I can't see any reason that your program reading some data would stop your CDU working.
Quoteshould I switch to the FSUIPC client version?
I'm not sure what you mean by this. If you're running your software on a different machine that the flight sim then you have to use WideClient. If you want it to talk directly to FSUIPC you need to run it on the flight sim machine. You don't need to make any change your software to do this. It will automatically detect either FSUIPC or WideClient.
Paul
-
Hi Ramon
I not sure what the problem could be. There should be no problem having multiple software programs using the same Wideclient connection.
I don't know how your CDU and the software works. Does the software need to have focus to work? If the CDU hardware is just seen as a keyboard then it probably needs to be the focused application. Having your own software in focus would stop it working.
If that's not the problem, you'll need to think about what your software is doing that could be interfering with the CDU software, or the communication with the CDU hardware. E.g. if the CDUs are on a COM port, is your software also using COM ports? The same one?
I may have some more ideas if you can let me know more about what your software does and how the CDUs are connected and communicate with Prosim.
Paul
-
The top 4 errors are because Windows has flagged the files as coming from the internet. If you right click them in the File Explorer and select properties you'll find an option to unblock them. It might be better to unblock the ZIP file and then unzip it again.
The 6 warning should resolve when you build the project for the first time and the NuGet components get automatically downloaded and installed.
Paul
-
Hi David,
You should just choose the update option here and let VS re-target to the 4.8 framework. It will work fine.
The example projects target 4.6.2 because that is the earliest version that Microsoft still support at the moment.
Paul
-
Hi Jason.
You'll need to ask John Dowson about this in the MSFS (FSUIPC7) support forum.
Paul
-
Hi,
You are getting the warnings because my DLL depends on the Win32 API and the WinForms library . Those are not included in the .NET Standard 2.0 specification.
It's telling you that if you use my library, your application will no longer be .NET Standard 2.0 compatible. e.g. It won't run on Linux.
Running it on a Windows .NET 8 machine will be fine because that platform does include the Win32 API and the WinForms library.
Since my DLL cannot work on any platform except Windows I cannot make it target a cross-platform specification like .NET Standard 2.0. Even the .NET versions I do target have to be the specific 'windows' versions. e.g. net6.0-windows not net6.0.
If you must use Standard 2.0, the only thing you can do is tell VS to suppress the warning, but your app will not really be compatible with Standard 2.0.
Paul
-
Hi Kees,
This forum is for .NET programming; your questions seems to be about Lua so this isn't really the best place to get help with that.
However, you'll probably get better performance by using the lua event system for LVars:
event.Lvar("lvarname", interval, "function-name")
This will call the specified function when the value of the LVar changes. That function can then set the value in the offset. I think this will reduce the processing from the current situation where you are reading the writing every LVAR every 100ms.
I don't really know lua that well, so If you want more information about this, please ask John Downson in the main FSUIPC forum (not any of the sub-forums) at the link below.
https://forum.simflight.com/forum/30-fsuipc-support-pete-dowson-modules/
Paul
-
My DLL has no way to access SimConnect variables.
I know FSUIPC7 has a way to map a simconnect variable to an unused offset, but I'm not sure if this works with a whole structure, or if it works with earlier versions of FSUIPC.
@John Dowson will be able to tell you if it's possible to access this simconnect data via offsets. If he doesn't see this and reply here, please ask him directly in the main FSUIPC support forum.
Paul
-
Hi Robert,
I don't know anything about the P3D shared cockpit feature, but it sounds like these particular variables are not getting shared between the copies of P3D. I guess P3D can't share everything due to bandwidth, especially if you're using third-party aircraft.
QuoteWould I have to store each value in a seperate offset then have a client app to read it and process the LVARs on the individual computers?
FSUIPC itself doesn't do any cockpit sharing so offset values will also not be shared unless they are backed by P3D data that is shared. So writing to spare offsets on one copy of P3D will not be seen on the other.
I don't think there's an easy way to do this. You'll need to come up with a way to pass the data between the two PCs yourself.
The obvious way is probably to use a TCP link between your application running on the the server and the client(s) and pass the LVAR data between them. .NET has a TcpClient and TcpListener class. Your application can then exchange the LVAR data over the TCP link and update the local copies of the LVARs using FSUIPC.
There might be some .net libraries that make data exchange easier than writing your own networking code, but I've never looked into this.
Paul
-
Hi,
I think you've posted in the wrong sub-forum. This is for questions about programming FSUIPC applications in .NET languages.
I recommend reposting in the main FSUIPC support forum. Follow this link, scroll down a bit and find the [Start New Topic] button.
https://forum.simflight.com/forum/30-fsuipc-support-pete-dowson-modules/
Paul
-
The NAV offsets are stored in hexadecimal, not decimal like the COM offsets.
To convert the value, you must convert it to a string using hexadecimal format. Then you need to add the 1 at the front and the decimal point in the middle...
string nav1String = nav1offset.Value.ToString("X4"); // Convert to hex - exactly 4 digits nav1String = "1" + nav1String.Substring(0, 2) + "." + nav1String.Substring(2, 2);
In your example you got the value 4176. In hexadecimal this is 1050. Which represents the frequency 110.50
Paul
-
1
-
-
Great! Glad it's all sorted. It was baffling me for sure. I hadn't considered you were using the untyped Offset class.
I've reproduced the error here and I've made a small change to the DLL so that it gives a much better exception than "index out of range":
FSUIPC.FSUIPCException: 'FSUIPC Error #999: FSUIPC_ERR_WRITE_OVERFLOW. Offset 66C0 is declared with length of 9 Bytes. The data you are trying to write is different from this. (8 bytes)'
Version 3.3.9 is now on NuGet.
Paul
-
That line number doesn't really help unfortunately. Can you make sure FSUIPCConnection.DLLVersion is reporting 3.3.8?
Also some of this info might help:
1. are you using any named offset groups anywhere in your application? Or are you just using the blank default group?
2. is there any multi-threading in your application? Or is everything run on the main UI thread?
Paul
-
Okay - here's the file. Copy it to your application folder (along side the fsuipcClient.DLL).
When you get the stack trace it should give you the line number in my Process() method where it's failing.
Paul
FSUIPC is connected, but can't get values from offsets
in FSUIPC Client DLL for .NET
Posted
I ran your code here and it works fine. I deleted the parts specific to your application that I don't have (mainly in the 'catch' statements).
I was able to use it to display the player lon/lat on a WPF window.
Whatever the problem on your side, it's not this class (unless it's the parts I had to delete). Maybe start with the code below and work your way up to see when it breaks.
This works for me:
Paul