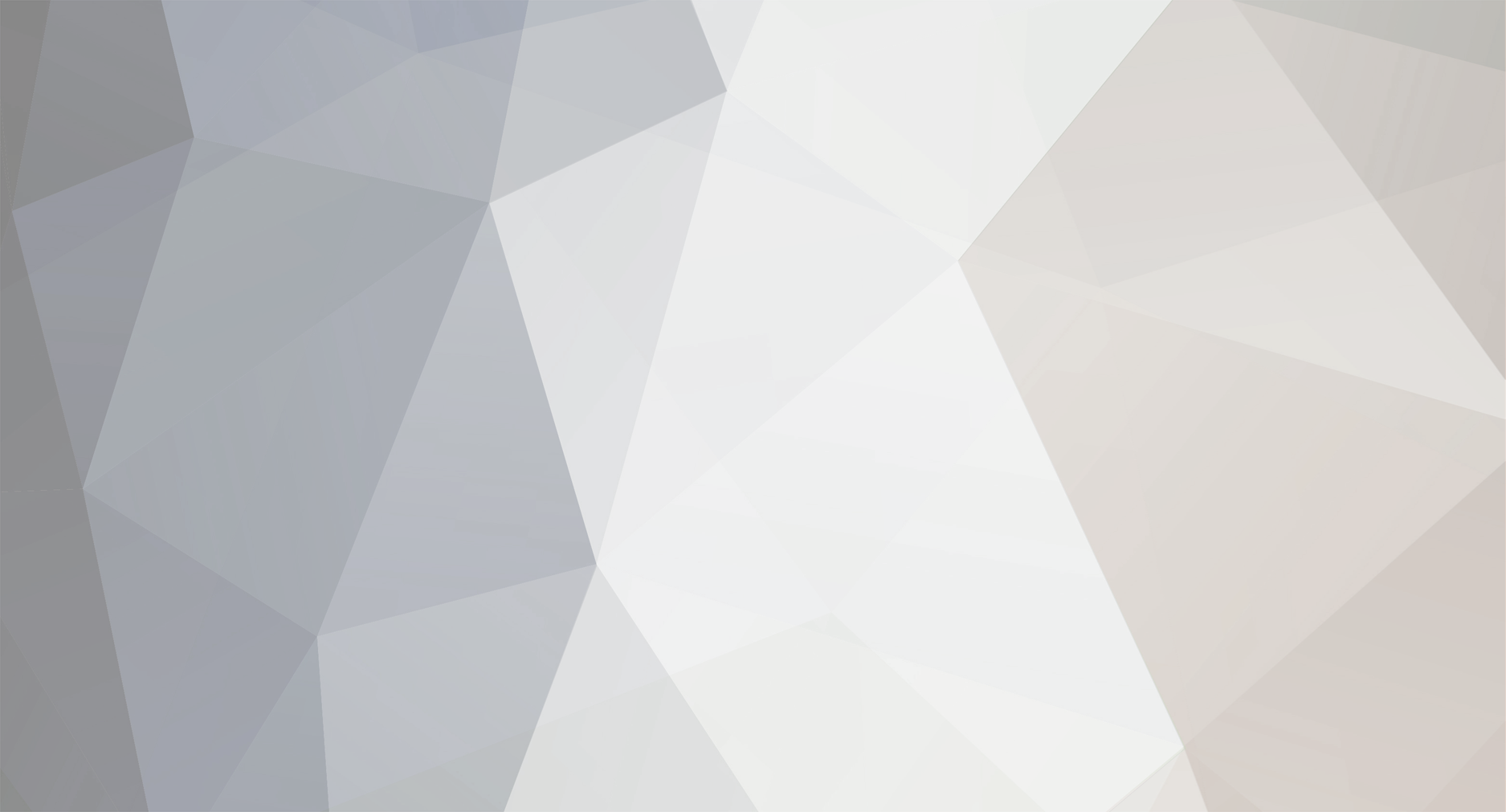
Paul Henty
Members-
Posts
1,724 -
Joined
-
Days Won
76
Content Type
Profiles
Forums
Events
Gallery
Downloads
Everything posted by Paul Henty
-
Visual Basic 6, reading Lat and Long
Paul Henty replied to superdudes's topic in FSUIPC Support Pete Dowson Modules
The code at the top of the following thread shows how to read the lon and lat in VB6. It uses a currency type which is actually a 64bit integer type in VB6. http://forums.simflight.com/viewtopic.php?f=54&t=72968&start=0&st=0&sk=t&sd=a Paul -
Strange value for 0C48 and 0C49
Paul Henty replied to javiercuellar's topic in FSUIPC Support Pete Dowson Modules
Oh dear. How embarrassing. My DLL doesn't support signed bytes! I'll fix this tomorrow and PM you a new version. Paul :oops: -
Strange value for 0C48 and 0C49
Paul Henty replied to javiercuellar's topic in FSUIPC Support Pete Dowson Modules
Hi Javier, If you declare the offset as a signed byte you won't need to do any conversions. The base type for Signed bytes in .NET is System.SByte. The c# alias for this is 'sbyte', for VB it's 'SByte'. Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
Hi javiercuellar, Each Process() call you make has an impact on performance because of the overheads involved in getting the data from FSUIPC. The amount of data you read in each process is not that significant in terms of speed. So getting 30 offsets in one process() is always going to be faster than making 2 process() calls of 15 offsets each. The grouping is good if you have groups of offsets that need to be updated at different rates, or updated conditionally like your example. In the 1.3 version of the DLL you need to call each group in a separate Process() call, so updating 3 groups would need 3 process() calls. This was a slight drawback in terms of performance. I've since added a feature (for the poster above you) that allows you to process multiple groups in one process call. The DLL bundles them into a single FSUIPC call. I'll PM you the beta 1.4 version in a few minutes so you can try it out. If anyone else wants this feature, PM me and I'll send you the beta also. Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
Hi, Firstly, calling Process() without a group doesn't process all the groups. It only processes offsets that are not assigned to any group. Calling 4 Process()'s is not very efficient and will probably take about 4 times longer than calling the same number of offsets in one Process(). At the moment the DLL does not allow you to process multiple groups in 1 Process() so you've no choice but to call Process() 4 times. I can add another overload to Process() that will take an array of groups. This will then bundle all the offsets in the given groups into 1 process call. I think this would be a very useful feature. I'll send you a beta version via PM for you to test when I've implemented it. Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
Hi, I can't see anything obviously wrong with your code. You can easily tell if my DLL (or your code) is causing the problem by looking at FSInterogate. If FSInterogate is reporting 1s as well then it's very unlikely the problem lies with your or my code. But surely all those aircraft have autopilots installed? Are you sure you've tried it with a plane without an autopilot? Maybe a glider (sailplane)? Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
I read it as he wanted to always round down. Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
I think you've got the parenthesis wrong there. You want to 'floor' the result of the division. You are dividing after you've done the 'floor' function. Try this: dmespdval = Math.Floor(vor1dmespd.Value / 10) Paul -
Help with FSUIPC SDK C# Altitude and Long/Lat
Paul Henty replied to vendetta's topic in FSUIPC Support Pete Dowson Modules
The altitude is marked in the SDK documentation as being 8 bytes. The same goes for the Lon and Lat. You must read these offsets into a variable large enough (8 bytes). An int in c# (on a 32 bit OS) is 4 bytes. You need a Long. long dwResult = -1; Obviously you can't then use the same dwResult variable for other offsets that are different lengths. You should really be defining specific variables to hold each bit of data you get back from FSUIPC, and typing them according to the specific FSUIPC offset. If you're not too far into development you might want to look at my FSUIPC Client DLL for .NET. It's far easier to use and more suited to .NET programming patterns than the old C# SDK that you are currently using. http://forums.simflight.com/viewtopic.php?f=54&t=53255 It also has good documentation explaining how to access different types of offsets and whhich C# (or VB) data types you need to use. Paul -
FSUIPC.bas and Microsoft Visual Studio 2008
Paul Henty replied to Gus172's topic in FSUIPC Support Pete Dowson Modules
I'm pretty sure that .BAS file will be for Visual Basic version 6. Visual Studio 2008 uses a different version of Visual Basic called VB.NET. It's a different language (although the syntax is very similar). That's why you're getting the errors. If you want to wirte FSUIPC apps in VB.NET using Visual Studio 2008 then go to this thread and download my .NET Client DLL. http://forums.simflight.com/viewtopic.php?f=54&t=53255 It has extensive documentation and example applications to help you on your way. Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
Can't see anything obviously wrong. As Pete says, there is no data being requested from FSUIPC. I can only imagine the Timer_Tick() isn't actually getting called. Try putting a breakpoint on one of the lines in Timer_Tick() to make sure it's being run. If it's not getting run then: Go to the form design view At the bottom, click on the Timer control. Go to the properties pane and put it into Event view by pressing the events button at the top of the pane. Make sure the Tick event has "Timer1_Tick()" as its value. I suspect this is blank at the moment. If this isn't the problem, and you are sure the Timer_Tick() sub is getting called, then let me know and I'll think about it some more. Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
Does the included sample VB.NET application work for you? If not there maybe something wrong with your FSUIPC install. If my app works then I'd need to see some code to see why yours isn't working. You can also use the FSUIPC logging features to see what values are being read from FSUIPC (if any). From the document "FSUIPC for Programmers.pdf" (FS9) or "FSUIPC4 Offsets status.pdf" (FSX) found in the FSUIPC SDK. Download it here: http://www.schiratti.com/dowson.html Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
Hi Pete and Roger, Sorry I've not chimed in on this - I've been in hospital for a couple of weeks after being thrown off a horse. Anyway - It looks like you've sorted it out between you - thanks Pete. I'm back on-line now so if you need any more help just ask... Regards, Paul -
FSUIPC: Reading Latitude from FS2004 with VB
Paul Henty replied to WN-Dotzigen's topic in FSUIPC Support Pete Dowson Modules
Sorry Werner, For some reason I thought you were using VB.NET. Anyway, here is a thread that shows how to read the Longitude and Latitude using VB6. The code in the first post apparently works. The poster's problem was elsewhere. http://forums.simflight.com/viewtopic.php?f=54&t=72968&start=0&st=0&sk=t&sd=a I hope this helps. Paul -
FSUIPC: Reading Latitude from FS2004 with VB
Paul Henty replied to WN-Dotzigen's topic in FSUIPC Support Pete Dowson Modules
Hi Werner, Looking at your code you don't seem to be doing the maths to get the Lattitude into the correct value. In the documentation is says: This tells you how to get the value out of that offset. You load it into a 64 bit integer (Long in VB.NET on a 32bit OS). Then you have to multiply the Long by 90.0/(10001750.0 * 65536.0 * 65536.0) to get the units right. The result of this calculation should be put into a Double. If you haven't gone too far with the old VB.NET SDK that you are using, I suggest you take a look at my FSUIPC Client DLL for .NET. It comes with extensive documentation and an example project in VB.NET. It is much easer to use than the SDK you are currently using. For example, if you use my DLL this is all the code you need to get the Lattitude and Longitude: Private latFS As Offset(Of Long) = New FSUIPC.Offset(Of Long)(&H560) Private lonFS As Offset(Of Long) = New FSUIPC.Offset(Of Long)(&H568) ' Convert Lat and Lon from FS Units into degrees (as a double). FSUIPCConnection.Process() Dim lat As Double = latFS.Value * 90D / (10001750D * 65536D * 65536D) Dim lon As Double = lonFS.Value * 360D / (65536D * 65536D * 65536D * 65536D) You can find it in the sticky at the top of this forum: http://forums.simflight.com/viewtopic.php?f=54&t=53255 Paul -
Firstly, I made a small error in the above post, which I have now corrected. Pete's document says the data in the level offset is a percentage but it's actually a fraction. I've corrected the above post by scaling the data in the level offset by 100. Anyway - here is some code which will read the data from the two offsets I mentioned and calculate the remaining fuel in US Gallons for the Left tank: This code uses three text boxes to display the data. They are called: txtLeftTankCapacity txtLeftTankLevelPercent txtLeftTankLevelGallons You need to declare the two offsets: Dim leftTankCapacity As Offset(Of Integer) = New Offset(Of Integer)(&HB80) Dim leftTankLevel As Offset(Of Integer) = New Offset(Of Integer)(&HB7C) Here is a method (fired from a button) that will read the data and work out the amount of fuel left. The data is displayed in the text boxes. Private Sub Button5_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button5.Click FSUIPCConnection.Process() ' 1. Display the capacity of the left tank in a text box (number of US Gallons when full) Me.txtLeftTankCapacity.Text = leftTankCapacity.Value.ToString() ' 2. Get the level of the left tank as a percentage (i.e. how full it is) Dim dblLeftTankLevelPercentage As Double = leftTankLevel.Value / 128D / 65535D * 100D ' 3. Display this in a text box Me.txtLeftTankLevelPercent.Text = dblLeftTankLevelPercentage.ToString("f2") & "%" ' 4. Work out how many gallons are left Dim dblLeftTankLevelGallons As Double = leftTankCapacity.Value * dblLeftTankLevelPercentage / 100 ' Or, if you can also do it like this: 'Dim dblLeftTankLevelGallons As Double = leftTankCapacity.Value * (leftTankLevel.Value / 128D / 65535D) ' 5. Display the amount left in the left tank in gallons Me.txtLeftTankLevelGallons.Text = dblLeftTankLevelGallons.ToString("f2") End Sub Regards, Paul
-
The offset before it (0B7C) tell you how full the tank is as a percentage. So if the tank holds 2500 and there is 1500 left, this offset will give you back 60%. If this offset says 25% then the tank only has 625 US gallons left. Offset 0B7C is an Integer (4 bytes). The documentation says: "% * 128 * 65536" which means it's a percentage but it's been multiplied by 128 and then 65536 before being stored here. To get the percentage back you need to divide the integer in this offset by these numbers: Fuel left (%) = Offset value / 128 / 65536 * 100 You can then work out the amount of fuel by using the capacity: Fuel left (us gallons) = Capacity * Fuel left (%) / 100 Or you can do the whole lot in one go: Fuel left (us gallons) = Capacity offset * (fuel left offset / 128 / 65536) Paul
-
Problem writing AI Traffic in FSUIPC4
Paul Henty replied to Paul Henty's topic in FSUIPC Support Pete Dowson Modules
This all looks fine now. Thanks again, Paul -
Nicholas , I ran your code here. It looks OK to me. I assume we're still talking about the fuel here. Your 'label26' is being regularly updated with the value of offset 0x090C - the amount of fuel used by Engine 1 in pounds. These looked sensible to me (I'm no expert on fuel use). As I increased the throttle this value increased at a faster rate as I would expect. When I shut down to engine this offset stopped changing. When you say it "doesn't work", what do you mean? Are you getting no values? The wrong values? If so, what are they? Also, do you realise this offset only works on FSX? It won't work on earlier versions like FS9. Paul
-
Problem writing AI Traffic in FSUIPC4
Paul Henty replied to Paul Henty's topic in FSUIPC Support Pete Dowson Modules
Hi Pete, Thanks for that, just tested 4.535 and it looks fine. There is just a small difference: The injected AI planes aren't being cleared after a few seconds of no updates. In FSUIPC3 they get deleted from the tables if you don't supply FSUIPC with regular updates. In FSUIPC4 they are still being reported by TrafficLook long after my test app closed down. Regards, Paul -
Hi, I looks like you're using my .NET Client DLL. In the ZIP there are some comprehensive documents that explain how to use the offsets. This includes a very helpful table showing you what VB type to use depending on the length and type of the Offset. Also the sample VB program shows exactly how to use read and write offsets. Please do read the documentation and help that I've already provided. That's not quite correct. The hex number is in VB is 90C not H90C. The H bit is not part of the number - it just tells VB that the number following is in Hex and not decimal. When you see a C hex number like 0x090C then in VB you just take off the 0x and add &H. So 0x090C becomes &H090C. VB may remove the leading 0 because it's redundent giving &H90C. As Pete said offset 0x090C is a 4 Byte (32-Bit) floating point offset. My documentation clearly states that this should be declared as 'Single' in VB.NET. Also you need a Process() call to actually read the data. This is also explained in documentation and the sample code. So your code should look something more like this. Dim Fuel As Offset(Of Single) = New FSUIPC.Offset(Of Single)(&H90C) Public Sub MySub() FSUIPCConnection.Process() Dim fuelleft As Single = (Fuel.Value) Label26.Text = fuelleft.ToString("f1") End Sub Paul
-
Problem writing AI Traffic in FSUIPC4
Paul Henty replied to Paul Henty's topic in FSUIPC Support Pete Dowson Modules
Hi Pete, Here's the WideClient log with the 2 AI Plane writes to 0x1f80: ********* WideClient Log [version 6.78] Class=FS98MAIN ********* Date (dmy): 18/09/09, Time 13:02:01.746: Client name is PJHLAPTOP 109 Timing Thread Started 141 SendReq Thread Started 468 Trying TCP/IP host "FSVisuals" port 8002 ... 468Okay, IP Address = 192.168.0.1 61839 Trying TCP/IP host "FSVisuals" port 8002 ... 61839Okay, IP Address = 192.168.0.1 123600 Trying TCP/IP host "FSVisuals" port 8002 ... 123600Okay, IP Address = 192.168.0.1 184861 Trying TCP/IP host "FSVisuals" port 8002 ... 184861Okay, IP Address = 192.168.0.1 206140 Sending computer name and requesting base data ... 206140 Button Thread Started 282393 New Client Application: "TestApp" (Id=4492) 282393 0 ReadLocal: Offset=3304, Size=0004 00 00 34 45 282393 0 ReadLocal: Offset=3308, Size=0004 08 00 DE FA 287666 Write: Offset=1F80, Size=0028 01 00 00 00 90 B1 44 42 38 AF EE BF CD 50 FA 45 7C 49 64 00 03 00 50 41 55 4C 31 00 00 00 00 00 00 00 00 00 00 8C 45 23 287666 Write: Offset=1F80, Size=0028 02 00 00 00 A1 C2 44 42 16 8D EC BF 66 86 7C 44 E4 4A 65 00 03 00 50 41 55 4C 32 00 00 00 00 00 00 00 00 00 00 8C 45 24 299054 Timing Thread Terminated 299054 Button Thread Terminated 299054 SendReq Thread Terminated 299054 ****** End of session performance summary ****** 299054 Total time connected = 93 seconds 299054 Reception maximum: 31 frames/sec, 846 bytes/sec 299054 Reception average whilst connected: 24 frames/sec, 621 bytes/sec 299054 Transmission maximum: 1 frames/sec, 79 bytes/sec 299054 Transmission average whilst connected: 0 frames/sec, 22 bytes/sec 299054 Max receive buffer = 595, Max send depth = 1, Send frames lost = 0 299054 **************** Individual client application activity **************** 299054 Client 4492 requests: 2 (Ave 0/sec), Data: 144 bytes (1/sec), Average 72 bytes/Process 299054 ********* Log file closed (Buffers: MaxUsed 2, Alloc 2244 Freed 2244 Refused 0) ********* There's no hurry on my part - I'm just adding a feature into my .NET client dll to make it easier for .net programmers to read/write AI traffic. Thanks, Paul -
Hi Pete, There seems to be a problem writing AI traffic in FSUIPC 4 (currently installed version 4.534) using offset 0x1F80. The FSX Status document says it's OK but it's doing some weird stuff. If I run the code on my FS9 setup it's OK. Here's a pic of TrafficLook with my 2 injected planes at the top - Paul1 and Paul2: If switch the wideclient to my FSX machine and run the same code I get this: G-TEXR was one of the AI generated by FSX. After this happens FSUIPC4 also stops responding to any IPC requests. Can you have a look at this please? Thanks, Paul
-
There's nothing in my DLL that will add any delay or jerkyness to the data. The exchange of data between the DLL and FSUIPC follows exactly the C SDK and is mostly done with unmanaged code. I think Pete is right about the need for interpolation code. Many years ago I did some work on an external panel that I wrote in DirectX. It too was visibly 'stepping' until I added some smoothing code to it. The problem you've got is that the values from FS only come at a fixed interval which is generally not small enough to make a gauge look really smooth. You need to update the gauge state more often than you get the data back. That way you can fill in the gaps between the data points making your gauge look smooth. Paul
-
Terms for redistributing SDK API code
Paul Henty replied to jkelloggs's topic in FSUIPC Support Pete Dowson Modules
Mark posted in this forum a few weeks ago. See the last post of this thread... http://forums.simflight.com/viewtopic.php?f=54&t=77011 You may be able to contact him via the private message (PM) or email button. Paul