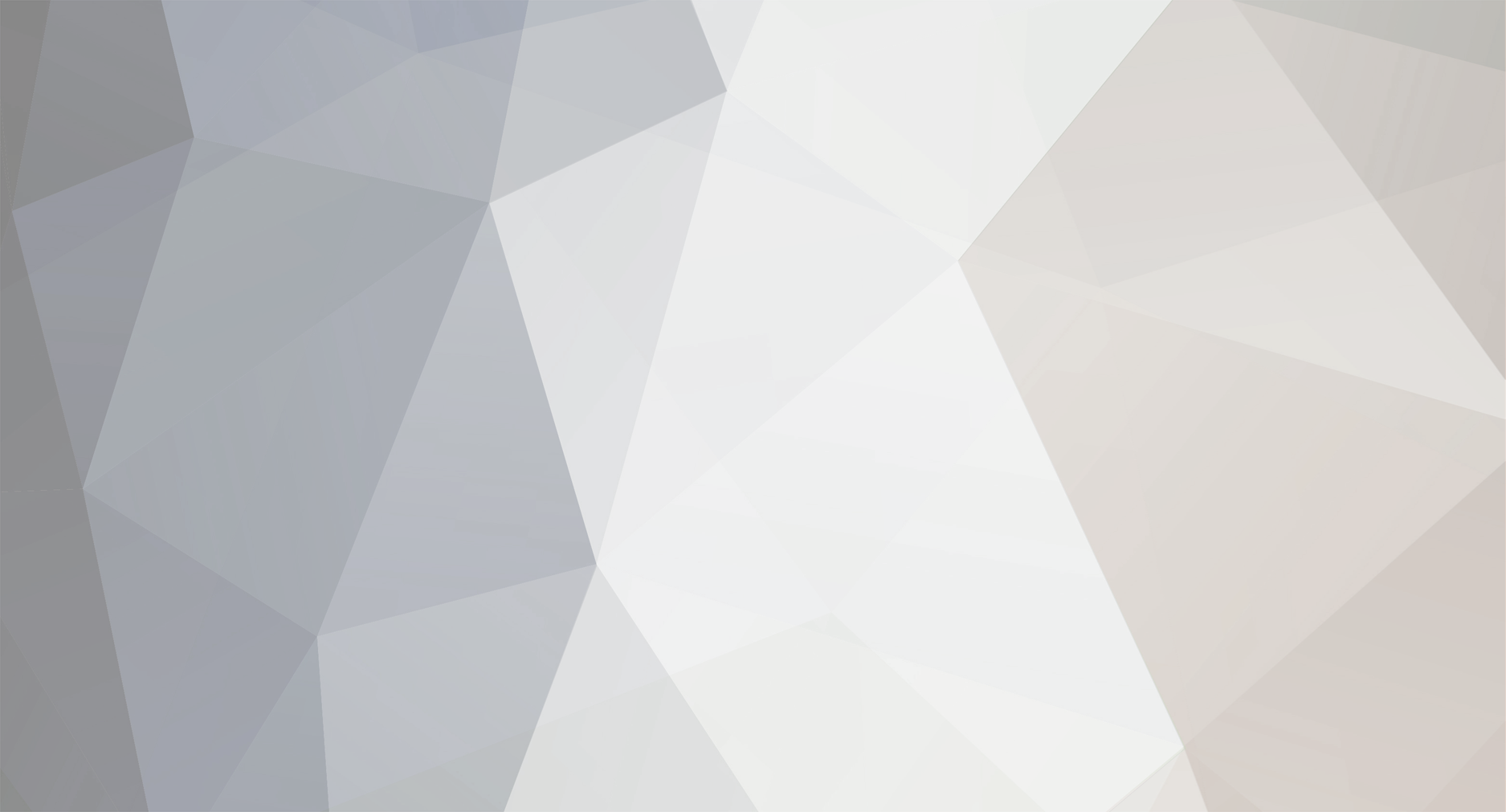
Paul Henty
Members-
Posts
1,724 -
Joined
-
Days Won
77
Content Type
Profiles
Forums
Events
Gallery
Downloads
Everything posted by Paul Henty
-
I got MSFS for a month so I can test this properly here. Sadly I can't reproduce the access violation at all. I've tried with my test code and your exact code from three posts up and it runs flawlessly. I did a few 30-minute flights with no problems. I've tried a few different aircraft but I can't see any CRJ so I guess that's in a better version of MSFS or it's third-party. So it could be something with so with the CRJ. Do you also get access violations with the built-in aircraft? Paul
-
Everything looks okay to me. I suggest using FSUIPC to log the A000 offset. This will take my DLL and your C# application out of the equation. In FSUIPC7 select Log->Offsets and log A000 as S32 (signed 32bit) a the MSFS Title bar: With your Lvar to offset set up in the ini file. you should see the value of A000 appear on the title bar. If it's 0 there then the problem is with your FSUIPC setup. You can go back to John with this logging result and without any C# code. If you can see a good value there then it's something in the C# side of things and we can continue here. Sorry this is frustrating for you, getting passed back and forth from me to John, but hopefully this strategy will make it clear where any problems are and you can go to the right person. Once you can see valid values in the offset with the FSUIPC7 logging then you can bring the C# part back into play. Paul
-
Ah so the CRJ isn't resetting the LVar itself. That's a pain, so yes you'll need to do this yourself. The delay requirement is likely to be down to a timer somewhere. Maybe the CRJ code checking the LVAR value, maybe FSUIPC checking the offset value. If you change it to 0 and then back to 1 too quickly (between another component's timer tick) then it won't notice the change. That would be my guess. There is also a whole chain of communication from my DLL to FSUIPC to the WASM module to SimConnect to the CRJ code so there are a number of places it could get missed if you change values too quickly. Paul
-
Yes, normally offset values are read from the sim when you call Process(). They only write if the value has changed. So setting the value to 1 when you have previously set the value to 1 won't trigger the write. Your code would be fine if the LVar took an explicit value for each state (which is usually the case), but since it's toggling with a 1 you'll need to use a write-only offset. These write on every process() even if their value has not changed. Because of this it's advisable to put them in their own group so you can control when they are written. var offset = new Offset<byte>("ToggleHeading", 0xA000, true); // write only Then your code will only need this: offset.Value = (byte)1; FSUIPCConnection.Process("ToggleHeading"); This uses a special offset that instructs FSUIPC to send the LVAR value. It's write only and clears itself internally after each request. So every time you call this method the Lvar gets written regardless of the previous value. Paul
-
I can't test this myself as I don't own MSFS. Your c# code looks fine. I don't think the problem is there. I notice you're using a profile called crj. Are you sure you've set this up properly in FSUIPC? Are you sure it's being used (active)? You could try it without using profiles - just change the section to be global: [LvarOffsets] 1=L:ASCRJ_FCP_HDG=UB0xA000 If that works then your crj profile isn't set up properly or isn't being activated. If it still doesn't work then John is really the person to help you on this. If you show him your full fsuipc.ini file he might be able to spot the problem. It's working via all the other code methods so the problem will be somewhere in your FSUIPC configuration. Paul
-
@kingm56 @activex Version 3.2.9 BETA is now on NuGet. (Tick 'Include Prerelease' to see it). This might fix the access violation problems. I've changed the way the incoming strings are handles from the C dll. It was technically wrong before so I'm hoping this will fix the problems. Also there are new overloads for Init() so you don't need to pass a window handle. The DLL will handle this internally. Paul
-
It could be, but since the error was from the WAPI dll I said to ask you. There are 2 users experiencing this exception intermittently but I can't reproduce it. I was hoping you might be able to shed some light on it with a line number of something. I'll take another look at my code and see if there's anything I've missed. Paul
-
I don't think it actually uses it to access your window. I think it's just a unique ID that the WASM module uses to keep the requests from multiple clients separated. You can probably just create an IntPtr from a random Int32 value and pass that instead. Yes, if the random pointer works I could add a constructor without the handle parameter and make a random ID inside the dll. SD is signed double word which in C# would be a normal 4 byte 'int'. So check your A000 offset is declared as 'int'. If you don't mean to be working with a c# int you'll need to change SD to the appropriate size: c# -> size in ini file sbyte -> SB byte -> UB short -> SW ushort -> UW int -> SD uint -> UD double -> do not specify a size. Paul
-
Sorry for the broken links - things are still in development and are getting moved around. I'll amend the post. The latest is 0.5.2. Here is the link: http://www.fsuipc.com/download/FSUIPC-WASMv0.5.2.zip If your browser blocks this direct link, goto: http://www.fsuipc.com/ and search for 'WASM'. That's the .lib file, not a dll. Please use the DLL from the zip file above. To use the dll to your project in Visual Studio: In Visual Studio you can add this DLL to your project as a normal project item and set the property "Copy to Output Directory" to "Copy if Newer". Yes, FSUIPC7 now supports this legacy method of reading and writing LVARS. It was designed back in the days of FSX and is provided now so that old applications using FSUIPC will now work on MSFS. You can use this feature if you like (it's a bit easier) but it's significantly slower than using the new MSFSVariableServices. Each LVAR read/write takes up a whole IPC exchange to itself. If you're accessing 10 variables this might be okay. If you want to read 30 or more in real time, this method is going to be too slow. Paul
-
Detect Current Airport or Closest Airport
Paul Henty replied to Alp's topic in FSUIPC Client DLL for .NET
That's true. FSUIPC cannot tell you which airport you are at, or are near to. Paul -
Detect Current Airport or Closest Airport
Paul Henty replied to Alp's topic in FSUIPC Client DLL for .NET
If you're using my Client DLL for .NET languages you can use the AirportsDatabase service for this. It reads the files generated by Pete Dowson's MakeRunways program. For details and examples, please look in the Example Code application available on my Website: http://fsuipc.paulhenty.com/#downloads Go through the examples under the section called "Airports/Runways Database" to see how to use the database. Example "DB003 Finding Airports In Rage" shows you how to get the nearest airport to the player. You can use this to find out if the player is at an airport and which one it is. Paul -
No, this works fine in the .NET client DLL. Are you using the UpdateExtendedPlaneIndentifiers() method like I told you in the other thread you posted? Have you tried the AI Traffic Examples in the "Example Code" application? You can download it from my website here: http://fsuipc.paulhenty.com/#downloads Run this and go to "AI003 Getting Extended Information" under "AI Traffic". If it works in the example app, please see the code in the form for how to get the extended information. If it doesn't work in the example app then it's a problem with FSUIPC7. Please report this to John in the FSUIPC7/MSFS sub forum. Paul
-
How do I know, the sim has exited?
Paul Henty replied to Karli D's topic in FSUIPC Client DLL for .NET
I recommend asking John for this in the FSUIPC7/MSFS forum. He's unlikely to see this thread. https://forum.simflight.com/forum/183-fsuipc7-msfs/ Paul -
How do I know, the sim has exited?
Paul Henty replied to Karli D's topic in FSUIPC Client DLL for .NET
Versions prior to FSUIPC7 all ran inside the Flight Sim process, so FSUIPC could never be left running on its own, so this was never a problem. I don't know if John has added an offset to FSUIPC7 to know if the Flight Sim connection has been lost. I can't see anything on the spreadsheet. It might be a good feature to request. For now, you could try something like reading offset 0x0230 (Double) which is a timestamp in seconds. If this doesn't change for a while then you know the connection to MSFS has been lost. Paul -
These are extended identifiers. You need to enable them using the AITrafficServices.UpdateExtendedPlaneIndentifiers() method. The way FSUIPC works, it can take a while to get the extended information which is why you need to opt in. The info is cached so the delay is only noticeable when you first update the AI Traffic and when new planes come in to range. Paul
-
possible threading issues with pmdg cdu data refresh
Paul Henty replied to Jason Fayre's topic in FSUIPC Client DLL for .NET
No, the fix was all within the dll. Paul -
possible threading issues with pmdg cdu data refresh
Paul Henty replied to Jason Fayre's topic in FSUIPC Client DLL for .NET
Hi Jason, The CDU class was using the same offset group between multiple instances. I've changed this so each instance will now have its own offset group. This should fix the problem. Version 3.2.8 is now on NuGet. Paul -
MSFS is still under development so many functions are not yet exposed for developers to use. If you have questions about MSFS specifically, it's best to ask John Dowson in the FSUIPC7/MSFS sub-forum. https://forum.simflight.com/forum/183-fsuipc7-msfs/ Paul
-
That error means there is a mismatch somewhere between 64 and 32 bit. Is it compiled to x64 only, or it is "Any CPU"?. If it's "Any" then your DLL will run in 32 bit if the exe or process it's running in is 32 bit. When the host application is running, check the Windows Task Manager. If it's running as 32 bit it will add this to the end of the name. Paul
-
The other thing to check is that you're compiling your application as a 64bit application (targeting x64). Or, if your compiling for "Any CPU" make sure you've unchecked "prefer 32-bit". Since WAPID.DLL is 64 bit your application will also need to be running as a 64bit application. You'll find these in the project properties under the 'Build' tab. Paul
-
No, I can't see any way of doing that. Paul