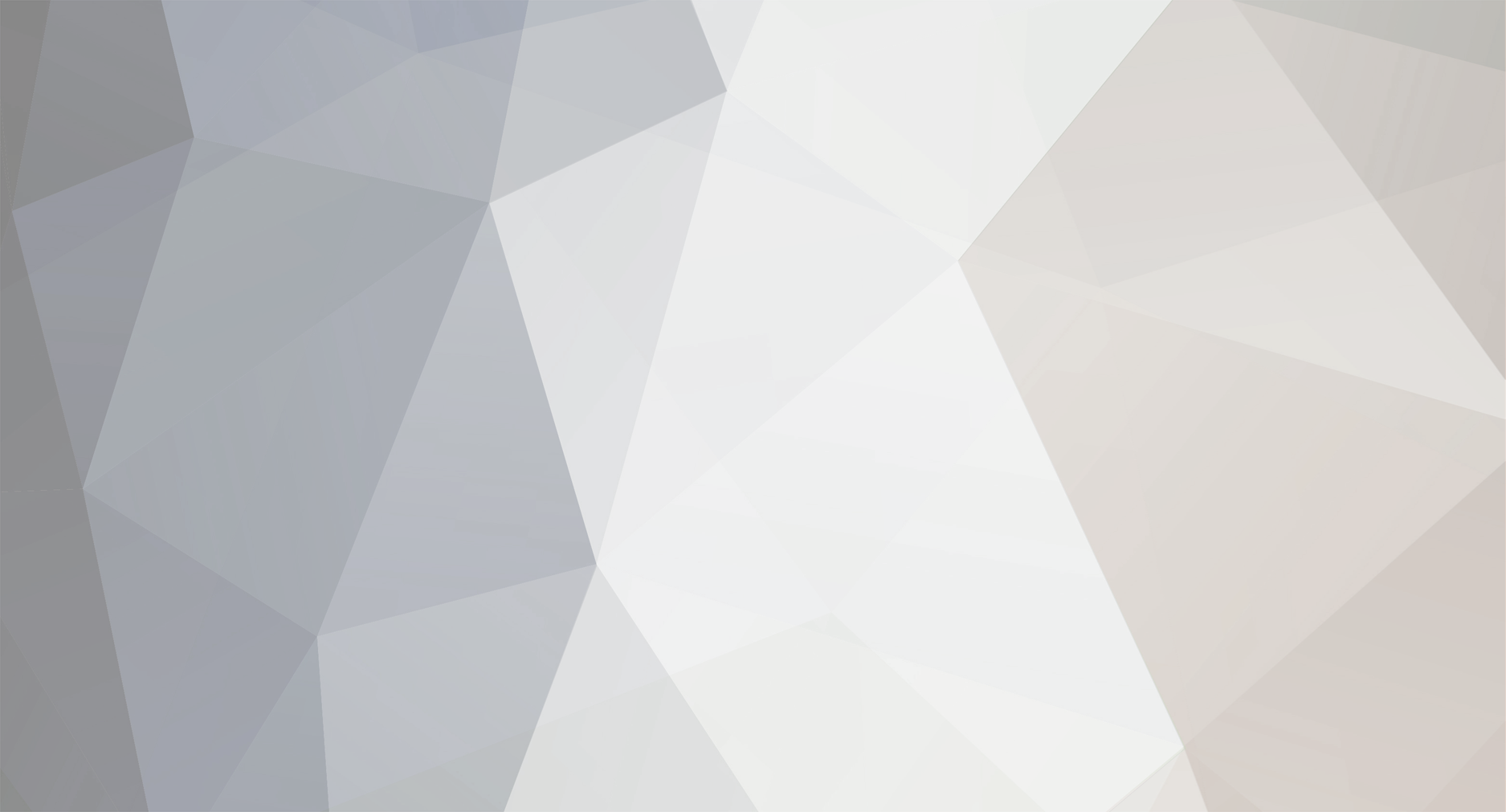
Paul Henty
-
Posts
1,652 -
Joined
-
Days Won
74
Content Type
Profiles
Forums
Events
Gallery
Downloads
Posts posted by Paul Henty
-
-
Got it, thanks. So it sounds like the WASM ini should be set to off when using my dll. Is that the default?
Paul
-
Quote
It is still very weird. In Debug mode it runs fine. In release mode with Visual Studio open it runs fine. Standalone I get within a short period of time (after a couple of minutes or so) this error.
You're probably running with the debugger attached in both cases. (F5). Try running the release version without the debugger (ctrl-F5). That should be the same behaviour as running outside of VS.
If it is (i,.e. it crashes when running via ctrl-f5) then it's very likely a timing issue. Your app is running slower with the VS debugger attached.
For John:
QuotePresumably the VS.LVARUpdateFrequency in the MSFSVarServices C# code sets the WAPI parameter LvarUpdateFrequency
That's correct.
QuoteIf the lvars are being updated by the WASM itself, you should set the frequency in the client to be 0. Alternatively, if the client is requesting the lvar updates, you should set the update frequency in the WASM to be Off.
I'm a bit confused about this bit. My DLL relies only on the call back registered with fsuipcw_registerLvarUpdateCallbackById to know when LVars have changed. (Parameter -1 for all Lvars).
In this case, which setting is relevant: the wasm ini frequency or client frequency?
Paul
-
[ERROR]: Error setting Client Data lvar value 295=58.039216
This error is being generated by @John Dowson's WASMIF module. Looking at his source code it's generated when a SimConnect call fails when trying to write the LVar value.
John might have some ideas about why this could happen.
Quote. The program works fine when running in Visual Studio debug mode. But when it runs in release without Visual Studio, I will get an error after a while like this:
The main difference would be the speed your application runs at. It will be much faster in release. Maybe the writes are too fast and SimConnect is getting overwhelmed. It would depend on how you're controlling the timing though.
Most of the .NET timers only tick as fast as the code running on the Tick event can complete. It could be that in debug mode the timer is running slower than your timer interval setting. But in release the timer tick code runs faster and the timer ticks AT the timer interval setting.
Paul
-
Quote
I do not understand the x2 and [2] in the offset description.
It just means that there are two of these offsets, one for each switch.
So for the CRS switch the first one is at 6520, the second will be 2 bytes later (Short is two bytes) at 6522.
For your flight director led, the first one is at 6538, the second one will be 1 byte later at 6539.
You might find it easier to use the provided 737 Offsets class as all this is handled for you. You also won't need to code the offset declarations.
To get the information you want you would use this code:
1. Create an instance of the PMDG 737 Offsets class at the form level:
Dim PMDG737 As PMDG_737_NGX_Offsets = New PMDG_737_NGX_Offsets()
2. Refresh the data (like calling Process()) and read the offsets you want. All offsets are named like the offsets pdf.
PMDG737.RefreshData() label1.Text = PMDG737.MCP_Course(0).Value label2.Text = PMDG737.MCP_Course(1).Value radioButton1.Checked = (PMDG737.MCP_annunFD(0).Value = 1) radioButton2.Checked = (PMDG737.MCP_annunFD(1).Value = 1)
Note that if there is only one switch or display, you don't need the array accessor at the end. e.g. for the MCP heading it's just:
lblHeading.Text = PMDG737.MCP_Heading.Value.ToString()
Paul
-
1
-
-
You need to use both. There is not enough information in 0x3124 alone. The platform type at 0x3308 will tell you how to interpret the version code at 0x3124.
In short:
If it's P3D or MSFS you divide the version code by 10. (e.g. 21 = V2.1).
if it's FSX then version code 1= RTM, 2 = SP1, 3 = SP2 and 4= Acceleration (all non-steam editions). Anything over 100 means Steam Edition and you subtract 100 to get the specific build number. (e.g. 109 = Build 9 = Last stable release. 112 = Build 12 = Latest Beta).
Paul
-
Quote
however I have one user that is getting a value of 112 which I think is the value range for Microsoft Flight Simulator 2020 (110 and up?).
The 0x3124 offset is the specific version number of the flight sim. This means it has different sets of values depending on which flight sim you are connected to. FSX has one range of values. P3D has a different set of values etc. Some values may overlap between sims.
You can only know which flight sim you are connected to with offset 0x3308, not with 0x3124.
For FSX, the value 112 in offset 0x3124 means build 12 of the Steam Edition (Version 10.0.63003.0), which is the latest beta version. The last stable release for FSX-SE was 9 (109), which was probably the latest version when the documentation was written, and the version you get if you don't opt into betas on Steam.
Paul
-
First, create some constant definition for the PMDG Mouse Click codes: (Put these at the class or form level).
Const MOUSE_FLAG_RIGHTSINGLE As UInteger = &H80000000UI Const MOUSE_FLAG_MIDDLESINGLE As UInteger = &H40000000UI Const MOUSE_FLAG_LEFTSINGLE As UInteger = &H20000000UI Const MOUSE_FLAG_RIGHTDOUBLE As UInteger = &H10000000UI Const MOUSE_FLAG_MIDDLEDOUBLE As UInteger = &H8000000UI Const MOUSE_FLAG_LEFTDOUBLE As UInteger = &H4000000UI
Events are also known as 'Controls'. My dll uses the term Control instead of Event.
Use the FSUIPCConnection.SendControlToFS() method to send the control/event ID. There is an Enum called PMDG_737_NGX_Control that you can use instead of calculating the event IDs.
You also need to send a parameter value. For PMDG controls, this is one of the mouse codes above.
For example, this will simulate a single left mouse click on the left Flight Director switch:
FSUIPCConnection.SendControlToFS(PMDG_737_NGX_Control.EVT_MCP_FD_SWITCH_L, MOUSE_FLAG_LEFTSINGLE)
The control will be sent immediately. There is no need to call Process().
Paul
-
10 hours ago, cncandi said:
But I need a documentation for using LVars in C#. Maybe WASM Client si available in C# as well?
Yes, you can use the MSFSVariableServices class in my DLL. Make sure you have version 3.2.19.
The main documentation for this class is a separate example project. You can download it here:
http://fsuipc.paulhenty.com/#downloads
Also, the first post in the following thread has an overview of this class, requirements and how to use it:
QuoteOr is there a Offset List for FBW as well?
I don't think so, people seem to be using LVars and HVars for this aircraft.
Paul
-
Probably you have not enabled the second CDU broadcast in the ini file: (In bold below).
QuoteTo enable the data communication output from the PMDG aircraft, you will need to open the file
737NGX_Options.ini (located in the FSX folder PMDG\PMDG 737 NGX, and add the following lines
to the end of the file:
[SDK]
EnableDataBroadcast=1For CDU screen data you also need one or both of these lines:
EnableCDUBroadcast.0=1
EnableCDUBroadcast.1=1Which enable the contents of the corresponding CDU screen to be sent to FSUIPC.
If you have that line but it still doesn't work, let me know and I'll investigate more. Also let me know which flight sim you are using.
Paul
-
Version 3.2.19 is now on NuGet.
Details of the changes to MSFSVariableServices are here:
https://forum.simflight.com/topic/94374-breaking-changes-for-msfsvariableservices-in-version-3219/
Paul
-
Version 3.2.19
Unfortunately, I've had to introduces some breaking changes to MSFSVariableServices.
This had to be done as the old way was wrong and was (understandably) causing confusion for users working with this class for the first time.
The breaking changes are:
1. The MSFSVariableServices is now a static class.
Until now, you needed to make an instance of the class: e.g.
MSFSVariableServices vs = new MSFSVariableServices();
This implies that more than one can be created and that you can dispose of this class. This is not the case because of how the FSUIPC_WAPID.DLL works.
Now you directly access the class using it's name. e.g.
MSFSVariableServices.Start();
You can no longer create instances.
If you don't like using the full name you can create an alias at the top of your code file:
C#
using VS = FSUIPC.MSFSVariableServices;
VB.NET
Imports VS = FSUIPC.MSFSVariableServices
This will let you just use 'VS':
VS.Open();
2. You can no longer access an LVar or HVar based on it's ID.You can only use the variable name now. The ID was arbitrary and had nothing to do with MSFS. I can't imagine the ID was useful at all so it's been removed to simplify things.
Bug fix:MSFSVariableServices.CreateLVar() now accepts a double instead of an integer.
Improvement:Any FsLVar/FSHVar objects you keep a reference to will persist between Reload() and Stop()/Start() calls. This means any ValueChanged events you have registered will still be valid. In the previous version, new FsLVar/FsHVar objects were created by the DLL meaning your exiting references and events stopped working.
The example code applications in C# and VB.NET have been updated (V1.2) to use the new version of the DLL:http://fsuipc.paulhenty.com/#downloads
Paul
-
I will be making changes to MSFSVariableServces to prevent both these problems.
Paul
-
I don't have MSFS here so I'm not sure how useful your project will be. I can certainly have a look and see if I can see anything obvious. But I won't be able to run and debug it.
I still recommend the three steps in my last post:
- The debug/trace level log will likely show the problem.
- My example code should work and will show you if the DLL works okay.
- John's client program will show if the problem is in your MSFS/FSUIPC install is okay.
Paul
-
I've been looking into this in more detail.
When I made MSFSVariableServices a normal class, this was a design mistake by me. It should have been a static class. The FSUIPC_WAPID.dll that I use is written in a singleton pattern, and only one copy of that DLL can be loaded by my dll.
So it doesn't make sense to dispose the MSFSVariableServices once you create it. Setting the reference to null means that the .NET garbage collector will dispose of it for you.
I will change this to a static class in the future, but for now you should not dispose it or lose the reference to it by setting it to null.
QuoteAfter calling Stop() and Start(), the IsRunning getter returns false consistently...
This is a separate issue from the above. Here are some things you can try to find the problem:
1. Turn the logging level up (e.g. debug/trace) and see what the log says when the connection fails.
2. Try stopping and starting with my example code application for MSFSVaraibleServices: (You might need to update the FSUIPC_WAPID.dll and my DLL via nuget).
http://fsuipc.paulhenty.com/#downloads
If this works then something is different in your code. You can compare them.
3. Try stopping and starting with John's client program in the FSUIPC-WASMv0.5.6 package (\WASMClient\WASMClient.exe).
If this doesn't work either then there is problem with John's code, or your setup.
Paul
-
Hi,
First, can you please check that you're using all the latest versions:
My DLL: 3.2.17 (Update on NuGet)
FSUIPC: 7.3.1 (http://fsuipc.com)
FSUIPC_WAPID.DLL from the 0.5.6 version of the "FSUIPC WASM Module" package. (http://fsuipc.com)
If you're still getting the error:
Are you disposing of the MSFSVariableServices object, or setting the reference to null when this happens?
I think when you call stop() the WASM module will try to send back a log message to say it's shut down. If the MSFSVariableServices class no longer exists it'll throw this exception. The best thing is to keep the instance alive once you've created it. Calling Stop() is enough to shutdown the connection.
If none of that helps, perhaps you can post your code where you call Stop() and also where you create the MSFSVariableServices.
Paul
-
If you're using Simconnect and MSFS there is a SimConnect variable called CAMERA_STATE and one called CAMERA_VIEW_TYPE_AND_INDEX which looks like it will do what you want. You can make a request to John to add these as offsets.
https://docs.flightsimulator.com/html/Programming_Tools/SimVars/Camera_Variables.htm
I can't see anything like this in the P3D reference though.
Quotewhat I found is SimConnect_RequestCameraRelative6DOF that can provide X,Y,Z values, I could base on those values to detect if I'm inside the plane, what do you think ?
It depends if all views use the same 'eye-point' or not. The coordinates returned from this function are relative to the eye-point. So if the external view eye-point is different to the cockpit eye-point then you won't be able to do this. If they are the same you would then need to know the rough geometry of the plane to know if you were inside or out.
Paul
-
You can try offset 0x8320 (1 byte). The documentation suggests this might not be reliable. I don't know if this works in FSUIPC7.
That would be the only way using FSUIPC.
Paul
-
If you want to run everything on the NET core 3.1 runtime then you'll need a specific version of my dll for that. Your app might work with the existing dll, but your users will need the correct .NET runtime installed. I suspect it will use the 4.6 Framework version, so they'll need that as well as your core 3.1 runtime.
I'll have a look tomorrow to see if it's possible for the dll to target core 3.1. I believe it includes Winforms so it might be okay. I know I couldn't do Core 2 as there was no winforms. I don't think I tried 3.
Paul
-
NAudio is free (MIT licence) and you can extend it to play ogg files with the additional NAudio.Vorbis library. Both are available on NuGet.
https://github.com/naudio/NAudio
https://github.com/naudio/Vorbis
Paul
-
FSUIPC can play sounds within the sim via offset 0x4200, but it's limited to .WAV files only.
If you want to try this, I can give you some example code.
The only other alternative is to have your application play the sounds using the System.Media.SoundPlayer class.
This supports all types of sound files, but of course will play on the computer that your application is running on.https://stackoverflow.com/questions/3502311/how-to-play-a-sound-in-c-net
Paul
-
Hi Matthias,
For MS2020: I'm not sure that FSUIPC7 supports writing to these offsets at the moment. In the FSUIPC7 offsets status spreadsheet (offsetStatus-v0.27.ods) the payload offsets (starting at 0x1400) are marked as okay for reading but unknown/untested for writing. If you are testing with a third-party aircraft try one of the built-in aircraft. If you still have no luck you can ask John about this in the MSFS/FSUIPC7 sub-forum.
For XPlane: XPUIPC is only a partial emulation of FSUIPC. Some offsets are not supported. It sounds like the payload offsets haven't been implemented. You'll need to check with the developers if they are still supporting XPUIPC.
An alternative to XPUIPC is to use the 'XPlaneConnector' library (also on NuGet). It works differently to FSUIPC but does allow access to all the XPlane data. It works well but it's not well supported. If you need to read/write strings you'll need to download the source and compile it yourself as the NuGet package is out-of-date and doesn't support strings at the moment.
https://github.com/MaxFerretti/XPlaneConnector
Paul
-
Hi Matt,
Some things to check:
1. Your joystick/button IDs should be the same a FSUIPC displays on it's "Buttons and Switches" tab when you press the joystick button.
2.The buttons presses are only detected when the Flight Sim window has focus. It won't work if FSUIPC7 or your application are in focus.
3. Can you please let me know the values of offsets 0x2910 and 0x290C running under FSUIPC7, but *without* using any of the User Input features: (Both are 4-byte ints)
I would test everything here myself but I don't have MSFS.
Those offsets should tell me if there is a problem with FSUIPC7.
If none of this helps I can prepare a version of the DLL with debug logging that should pin the problem down.
Thanks,
Paul
-
Quote
Any plans to update your dll to take advantage of said offset?
There nothing for me to do; user input services is already in the DLL and works fine in FSUIPC4,5 and 6.
To check if it works in FSUIPC7, please try the UserInput examples in my ExampleCode solution on the website: (Please update my DLL to the latest version as these solutions tend to restore a previous version).
http://fsuipc.paulhenty.com/#downloads
If those examples do not work with FSUIPC7 then let me know and I'll speak with John to see where the problem is. Let me know if there are any exceptions thrown.
If they do work then compare the example code with your code.
When you test your code, make sure you're not ignoring any Exceptions with blank Catch() blocks. Any exceptions thrown by the DLL could point to what the problem is.
Paul
-
You can combine the modifier keys with the bit-wise 'or' operator (| in C#) like this:
FSUIPCConnection.SendKeyToFS(Keys.F1, SendModifierKeys.Control | SendModifierKeys.Shift, null);
Paul
FSUIPCConnection.SendKeyToFS - Question
in FSUIPC Client DLL for .NET
Posted
Hi Peter,
You can do this by converting the string into the 'Keys' enum using Enum.Parse().
(I think when you wrote Keys.1 you mean Keys.D1).
For this to work, the string will need to be exactly the same as the Enum value text.
Paul