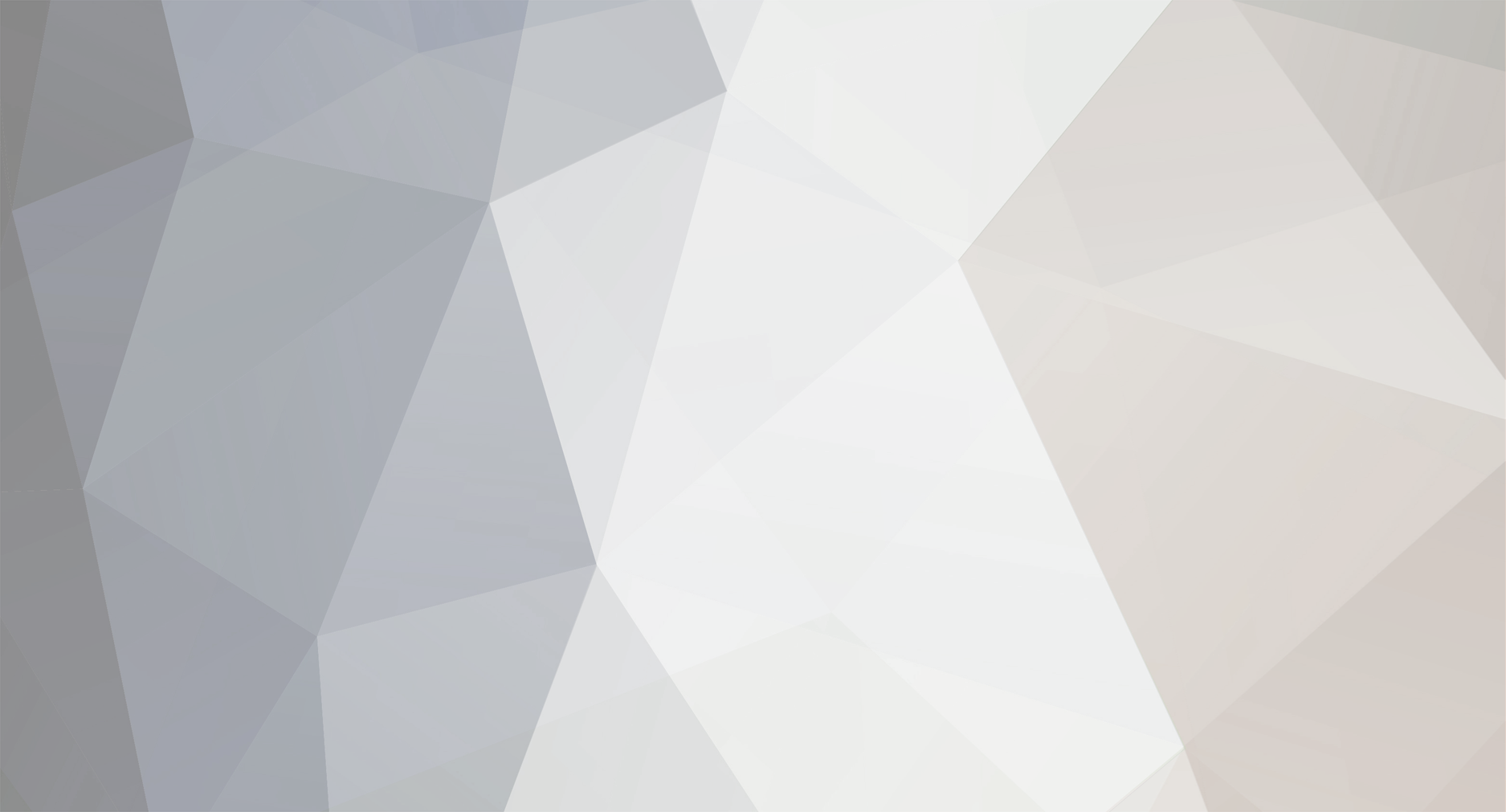
Paul Henty
-
Posts
1,652 -
Joined
-
Days Won
74
Content Type
Profiles
Forums
Events
Gallery
Downloads
Posts posted by Paul Henty
-
-
Paul, and if such offset, which considers the distance traveled in the simulator?
George
There is no such offset. You would need to keep track of this yourself. Every few seconds you should get the current position (lat/lon) and measure the distance from the previous position. Then add this to a total. I suggest adding another timer to the form and doing this every 10 seconds. The more often you track the position the more accurate the distance will be.
You can use the FsLonLatPoint class to measure the distance between two points in nm or km. E.g.
distanceFlown += currentPoint.DistanceFromInNauticalMiles(previousPoint)
Paul
-
Sorry about that. There was a bug with the write only offsets.
Fix attached.
Paul
-
Can you just do two quick tests for me? When you application is running can you please test the values of:
FSUIPCConnection.FlightSimVersionConnected to make sure it's coming back as 'Prepar3d'.
FSUIPCConnection.DLLVersion to make sure it's 2.6.5124. (This will show what dll was actually loaded, rather than checking the version number on the disk).
Thanks,
Paul
-
Here's the fix. Let me know how it goes. Two zips: one targets the .NET 2 framework, the other .NET 4 Client Profile.
EDIT: Bugs in this upload. See good attachments further down.
Paul
-
George,
1. Give me please a sample code, how to do it!
I really don't have the time to do this. This would be long and complicated code. All I can do is describe the process. The UserGuide shows you how the fsLonLatPoint class is used. I don't have MySQL so I cannot write the code to access the database.
2. How to add in DB coordinates of airport, in one field record the latitude and longitude once or each in its field?
You need two fields, one for Longitude and one for Latitude so you can filter on them.
What used offset this, and how to write it correctly?
Even92LN gave you sample code. This just shows you how to do it. It's not code you can just paste into your application. If you cannot see from that code how to make it work then you need to learn programming or c# first. I have suggested this many times.
This forum is for helping people use my DLL. I cannot write your application for you one feature at a time, sorry. I just don't have the time. This isn't my Job, it's just a hobby. I will answer questions about my DLL, but not about how to write your application.
Paul
-
or it must be coded in de DLL that is only fills those vaules when it is FSX
Good guess. Just checked the code and that's exactly the problem. Easy enough to fix though. I'll make a fixed version of the DLL for you over the weekend.
Paul
-
Hi Peter,
I've just reinstalled my laptop so my FSX/FSUIPC stuff isn't up an running yet. I'll get it installed at the weekend and test this for you - only on FSX though as I don't have P3D. At least if it works on FSX we can rule out a problem with the DLL.
Paul
-
MySQL I know well, so what exactly should the database from the airport:ICAO Code, IATA Code and Coordinates?George
Yes that's all you need to find the current airport.
You would do something like this:
1. Find current player position
2. Work out the Lon, Lat coordinates of a box 5 NM around the player
3. Select the airports from the database that have coordinates within this box
You can use the FsLatLonPoint class in my DLL to help with getting the coordinates of the test box. See the UserGuide.pdf for more details on this class.
If you get more than 1 airport back you can measure the distance to each one and find the closest. You can use FsLatLonPoint for this also.
Paul
-
MySQL Database for this good?
Yes that would be the easiest and fastest way if you know SQL.
Most people use text files, for example those produced by MakeRunways.exe by Pete Dowson. Text files are slower but people use them because they do not know how to use SQL databases.
Paul
-
Hi Jens,
The requirement for third-party keys was removed from FSUIPC a long long time ago. As your tests have shown your software will work on unregistered FSUIPC without any problem.
However, if your software is commercial then you should speak to Pete about using FSUIPC.
Paul
-
Well, and how then to define airport of departure that removed the ICAO code?
FSUIPC cannot tell you what airport you are at or departed from.
The easiest way to get this information is to provide a text box for the user to enter the ICAO code manually.
The much more difficult way is to have a database of all the airports with their longitude and latitude. You then take the player's Lon and Lat from FSUIPC and use it to work out which airport they are at.
Paul
-
And this Grund flag will not work on taxi?
It works all of the time. So when you're taxiing it will be 1. That's why you need to test it going from 0 to 1, not just being 1. So if it's 1 you only save your data if the previous value was 0.
Paul
-
Sorry but I don't know MySQL. Try looking for a MySQL tutorial or forum.
Paul
-
if (FSUIPCConnection.Open == true) { this.checksim.Text = CLIENT_MSG6_RU; } else { this.checksim.Text = CLIENT_MSG7_RU; }
That's it right?
No. That is not what is written in the example application. You need to open the example application and look at the code in there. Search for Open() and Process() and look at the try/catch blocks. The catch blocks catch the errors when FS is not loaded.
Paul
-
How to check whether the simulator is running or not?
You can only check this by trying the Open() or a Process(). If the simulator is not running these methods will throw an exception which you must catch.
The sample application shows this. Look for the Open() and Process() calls and you will see the Try, Catch blocks that detect if the simulator is running.
Paul
-
All done, it works, I'm not yet found one offset, what version of the simulator has been launched!
FSUIPCConnection.FlightSimVersionConnected.ToString()
Paul
-
I convert correctly?
Yes, that looks good to me.
As for the headlights, I did not understand, it is impossible to deduce (On, Off)!
This is as easy as I can make it:
1. At the top of your form with the other 'using' statements, add this:
using System.Collections;
2. Declare the offset as follows:
private Offset<BitArray> lights = new Offset<BitArray>(0x0D0C, 2);
3. Access each light as follows. This example is for strobe lights (bit 4). For other light numbers look up offset 0D0C in the "FSUIPC4 Offset Status.pdf".
// Strobe lights (Bit 4) if (lights.Value[4]) { this.lblStrobeLights.Text = "Strobes ON"; } else { this.lblStrobeLights.Text = "Strobes OFF"; }
Paul
-
1. What offset use for lights (landing, taxi, navigation, strobes for Read)?
0D0C. The sample application that I supply with the DLL shows reading (and writing) of lights.
2.What is the difference in the offset value "int", "Long", "short", "double", "string"?
These are c# types and hold different types of data. For example 'int' holds an Integer 32 bits wide. 'String' holds text. If you don't know what different types are you really need to learn some basic c#. Here is a web page that explains the types:
http://msdn.microsoft.com/en-us/library/ya5y69ds.aspx
If you want to know which c# type to use with each offset you need to read the UserGuide.pdf. Page 7 has a table showing which types to use depending on the size of the offset and what kind of data is stored in the offset. You need to also need to read the information about each offset in "FSUIPC4 Offset Status.pdf"
3.Whether correctly I convert ground speed?
Knots is correct.
To convert metres/second to Km/h you need to multiply by 3.6:
double gsKMH = ((double)airspeed_gs.Value / 65536d * 3.6d);
4.And how convert Zero fuel weight, empty weight and payload?
The easiest way to get this data is to use the PayloadServices in the DLL.
At the top of your form (where you declare the offsets), declare a payload services object called 'ps'.
private PayloadServices ps;
After you Open() the connection to FSUIPC, assign this object:
FSUIPCConnection.Open(); ps = FSUIPCConnection.PayloadServices;
Then when you want to get the payload data you can use the code like this:
ps.RefreshData(); //Empty weight in lbs & kgs double fw_ew_lbs = ps.AircraftWeightLbs - ps.FuelWeightLbs - ps.PayloadWeightLbs; double fw_ew_kgs = ps.AircraftWeightKgs - ps.FuelWeightKgs - ps.PayloadWeightKgs; this.emw1.Text = fw_ew_lbs.ToString("F0") + " (Lbs)" + " / " + fw_ew_kgs.ToString("F0") + " (Kgs)"; //---------------------------------------------------------------------------------// //Zero fuel weight in lbs & kgs double fw_zfw_lbs = ps.AircraftZeroFuelWeightLbs; double fw_zfw_kgs = ps.AircraftZeroFuelWeightKgs; this.zfw1.Text = fw_zfw_lbs.ToString("F0") + " (Lbs)" + " / " + fw_zfw_kgs.ToString("F0") + " (Kgs)"; //---------------------------------------------------------------------------------// //Weight payload in lbs & kgs double fw_pl_lbs = ps.PayloadWeightLbs; double fw_pl_kgs = ps.PayloadWeightKgs; this.payload1.Text = fw_pl_lbs.ToString("F0") + " (Lbs)" + " / " + fw_pl_kgs.ToString("F0") + " (Kgs)";
Paul
-
I have a problem, flaps are displayed correctly, but the slats are not, either 0 or 1 degree!
I also get 0 or 1 on the default Boeing 737 and 747. But on the default A320 I get more sensible values. There is nothing wrong with your code. These are just the values that FSUIPC/FSX gives us. Try on different aircraft. If you think they are wrong then ask Pete about these values in the main support forum.
And another question!Why do we need file: FSUIPCClient.XMLThis file holds the text for the Intellisense. This appears when you are programming inside Visual Studio. It gives information about the method calls and parameters for my DLL. You only need this while coding. You do not need to give this file to users.
Paul
-
Hi Even,
The only way to know this is to catch the errors produced when the FSUIPC Process() call fails.
If you are using my DLL the sample program that comes with it shows how to do this. Look at the code on the tick event for Timer1. You'll see a try/catch block where it catches the FSUIPCException from the Process() call which means FSX/9 has unloaded or crashed. It then closes the connection, tells the user, and lights a button so the user can try reconnecting.
If you're not using my DLL then it will depend on the programming language you are using. You would also be in the wrong sub-forum :-) so I recommend you post again in the main support forum (above this one) and include the language you are using.
Paul
-
1. How to deduce the state of the gear (Up, Down)?
Use offset 0BEC. This is for the nose gear. It is possible to get values for all three wheels if you want. Or just use one of them like my example:
Declare the offset as follows:
private Offset<int> gearPositionNose = new Offset<int>(0x0BEC);
Test the value to determine gear up (0), down (16383) or in transit (a value in between).
if (gearPositionNose.Value == 0) { this.lblGear.Text = "Gear Up"; } else if (gearPositionNose.Value == 16383) { this.lblGear.Text = "Gear Down"; } else { this.lblGear.Text = "Gear In Transit"; }
2.How to deduce the state of the wing (degrees slats and flaps)?
Below is an example for left wing inboard flaps using offset 30F0. You can do the same for other flaps and slats at offsets 30F0 to 30FE. Not all of these offsets will have values. It depends on the aircraft.
Declare the offset as follows:
private Offset<short> leftInboardFlap = new Offset<short>(0x30F0);
Calculate the flap angle and display:
double flapsDegrees = (double)leftInboardFlap.Value / 256d; this.lblFlaps.Text = flapsDegrees.ToString("F0");
For details of the other offsets I have mentioned, please look in the FSUIPC4 Offsets Status.pdf.
Paul
-
The ground speed in offset 02B4 is in metres/second. To convert to knots you need to multiply by 1.943844492:
double airpeedKnotsGround = (double)airspeed_gs.Value / (65536d * 1.943844492); this.label18.Text = airpeedKnotsGround.ToString("F0") + " Knots";
You have not shown me the offset declaration. You always need to show it. This offset should be declared as an 'int' like this:
Offset<int> airspeed_gs = new Offset<int>(0x02B4);
Paul
-
1
-
-
Your code works well for me.
If you are not seeing the Longitude and Latitude updating then make sure you have your events linked properly:
On the visual form designer, click on the timer1. In the properties grid, click on the lightning button to see the list of events. There is only one called Tick. Make sure it has 'timer1_Tick' next to it. If it's blank select timer1_Tick from the dropdown box.
Do the same for the buttons. Look for an event called 'click' and make sure the correct _Click event is selected from the dropdown box.
I notice that you are calculating things like Airpeed, but not displaying them. You need to create a label or textbox in which to display this information. Then you need to assign the calculated values to these labels or textboxes. For example to display the airspeed, create a label on the form designer called lblAirspeed. Then add a line of code after you calculate the airspeed to display the value:
double airpeedKnots = ((double)airspeed.Value / 128d); this.lblAirspeed.Text = airpeedKnots.ToString("F0") + " Kts";
The ToString() method converts the numeric value into a string so it can be displayed as text. The F0 rounds the value to 0 decimal places. For 2 decimal places you would use ToString("F2").
These are very very basic C# programming concepts. You should learn C# before you attempt something more complicated like getting data from FSUIPC. I really cannot teach C# here.
Paul
-
1
-
-
I can't see much wrong with the code except that you don't call displayCurrentPosition() anywhere so it's never run.
You need to call this from a button press, or a timer if you want regular updates.
Paul
Write programm on C#
in FSUIPC Client DLL for .NET
Posted
Hi George,
Offset 0020 is the height of the ground (above sea level) directly under the aircraft. So to get the altitude of the aircraft above the ground you subtract this from the altitude of the aircraft:
Paul.