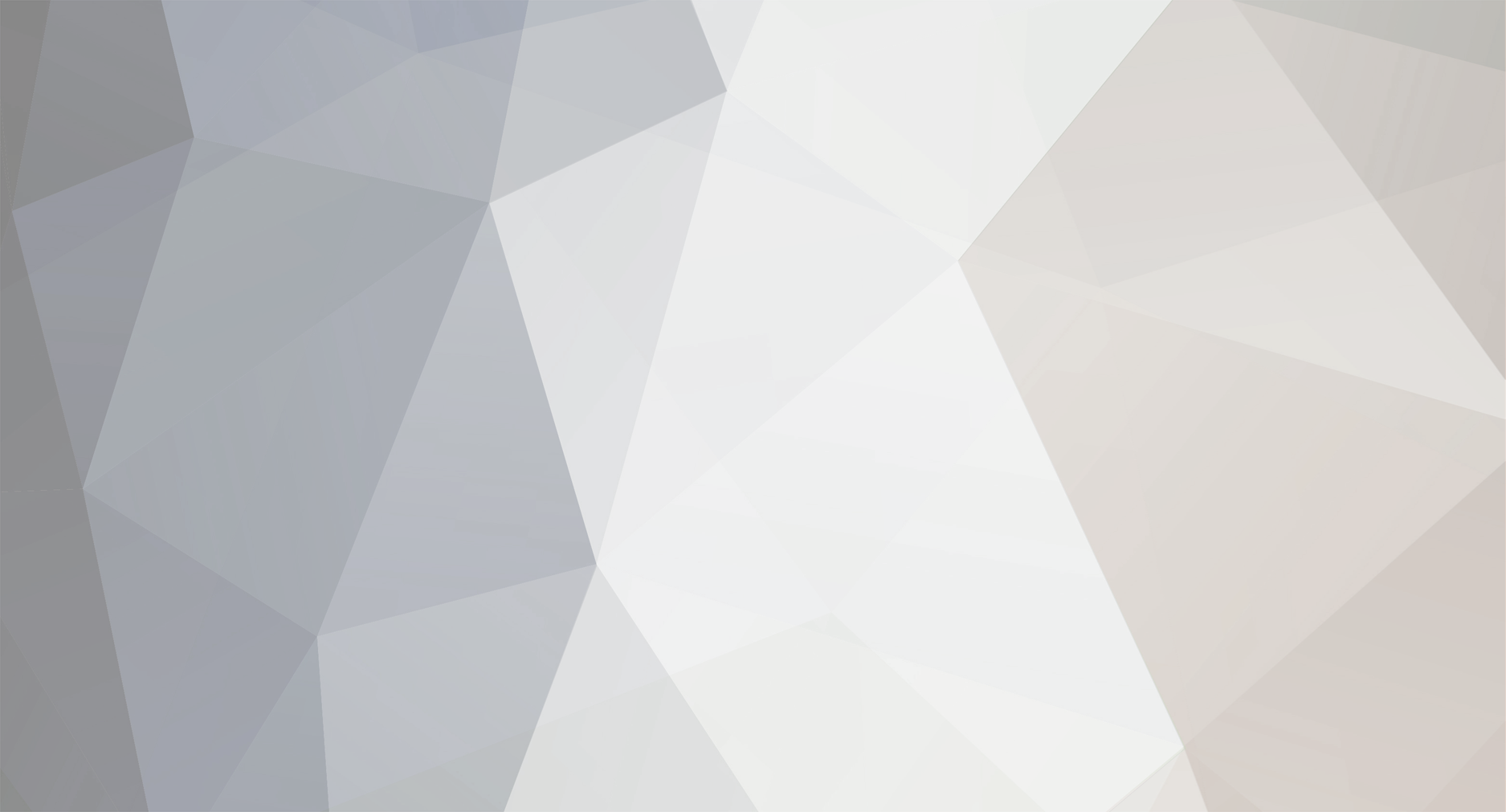
Paul Henty
-
Posts
1,652 -
Joined
-
Days Won
74
Content Type
Profiles
Forums
Events
Gallery
Downloads
Posts posted by Paul Henty
-
-
I don't understand Russian so I wouldn't be able to read it.
Paul
-
Welcome George,
1. Download the DLL Package from the sticky thread at the top of this sub forum:
http://forum.simflight.com/topic/74848-fsuipc-client-dll-for-net-version-24/
2. In the Docs folder open "UserGuide.pdf" and read the sections called 'Introduction' and 'Getting Started'
3. Load the Sample C# Project in Visual Studio. Run it and see what it does (It does quite a lot of what you want). Study the code to see how it works.
You will need to refer to the document called "FSUIPC4 Offsets Status.pdf" which gives details of all the information that you can get from FSUIPC. This is found under your main FSX folder in a sub folder called "\Modules\FSUIPC Documents".
If you have any questions about using the DLL, ask here.
If you have questions about FSUIPC in general please ask Pete in the main forum.
Unfortunately I cannot teach C# or programming here so if you need to learn to program you'll have to find some other resources for that.
Paul
-
1
-
-
Hi Even,
Offset 0x0366 is only 2 bytes. You're copying the value into dwResult which is 4 bytes (int). This means only the lower 2 bytes are getting updated by the C# SDK you are using so you've got the bad values.
The best solution here is to use the correct length integer (2 bytes called 'short' in C#) to receive the value. I think the following code will work but this isn't my SDK so I'm not very familiar with it.
public short getIsOnGround()
{
bool result = false;short onGround = 0;
int dwResult = -1;
int token = -1;
result = fsuipc.FSUIPC_Read(0x0366, 2, ref token, ref dwResult);
result = fsuipc.FSUIPC_Process(ref dwResult);
result = fsuipc.FSUIPC_Get(ref token, ref onGround);
return onGround;
} -
Hi Erik,
There are no offsets to tell you the starting location. In any case the distance between the starting point and the current aircraft location isn't really going to be the distance flown because not many routes are in a straight line.
You'll need to have a button in your application which the the user presses when they are at the starting location. When this button is pressed you read the current position of the aircraft and store it in a variable as the 'previous location'.
Then at regular intervals you get the current position again, work out the distance from the 'previous location' and add it to the total distance flown. Then you copy the current location into the 'previous location' ready for the next time.
The more frequently you sample the position the more accurate your total distance will be, and the more frequently you can update your display of the distance so far. I would suggest once every 30 seconds would be good enough but you could make it more or less frequent according to your preferences. You should add another timer to your form to do this calculation at your chosen interval.
Paul
-
Hi Erik,
There isn't one. You're program needs to track this during the flight. At regular intervals you need to calculate the distance between the current position and the previous position and add this to a total distance variable.
The DLL can handle the trigonometry for you if you use the FsLatLonPoint class. This takes in an FsLongitude and FsLatitude to specify a position on the Earth. You can use one of the DistanceFrom...() methods to calculate the distance between two positions.
The FsLongitude and FsLatitude classes take in the raw 8-byte value from the fsuipc offset so you don't need to worry about converting this to degrees.
For more details and examples, see the UserGuide.pdf:
"Reading and Displaying Longitudes and Latitudes" on Page 15 and
"Distances and Bearings between Two Points" on Page 17Paul
-
Hi Matthias,
All you need to do is convert the string into an integer that you can pass to the offset constructor.
Int32 has a parse Method that will convert a string in hex into an integer. You need to remove the '0x' though.
Here's the code:
string _Offset = "0x4433"; int intOffset = Int32.Parse(_Offset.Substring(2), System.Globalization.NumberStyles.HexNumber); _FsuipcOffset = new Offset<short>(intOffset);
When you've finished with the offset, make sure you call Disconnect() before you make a new one. If you don't, the DLL will still have a copy of it and will still read it when you call Process();
_FsuipcOffset.Disconnect();
Paul
-
Might they be 12-pin and 20-pin ribbon cables?No they're not. Sorry.
Paul
-
Installed FSUIPC Version 4.60 for FSX.
Hi,
Pete is away for a few days.
The earliest supported version of FSUIPC4 is version 4.91 which is available here:
http://www.schiratti.com/dowson.html
You might find that this fixes your problems.
Paul
-
You just need to declare the offset as a signed byte which is sbyte in c# or SByte in VB.
e.g. Offset<sbyte>
Paul
-
1
-
-
In my application I need to know these three things I haven't quite figured out.... Precondition being that the NAV1 radio is tuned to a VOR station and the a/c is within the signal reach of the VOR.
1. How do I obtain the radial of that station the a/c is currently in?
2. How to I calculate the bearing TO the VOR station based on the current position?
3. How do I know the radial FROM the VOR station the a/c is currently in?
A brief search of the Offset Status pdf turned up the following offsets:
1. 0C50
2. 0C56
3. Isn't this the same as 1?
For your prerequisites the ID of the currently tuned VOR can be read from offset 3000. I'm pretty sure this will need the pilot to be in range for this to appear. Otherwise you can check the distance with offset 0300.
All these offsets are for NAV1 but there are also equivalents for NAV2.
Paul
-
Maybe there is something wrong with my data type?
Hi,
I don't know much about C(++) but it looks like you've declared Value as a 64bit integer and then you're casting it to a 64bit Float (double). This won't work because the cast just makes a new double with the same value as the integer; it doesn't change the data type of the variable (i.e. how the bits are interpreted).
You need to declare 'Value' as a double, then the bits returned from FSUIPC will be interpreted in the correct way.
Paul
-
1
-
-
Thanks for your help Pete, It's working now.
Paul
-
Hi Pete,
I'm adding weather handling to my .NET DLL but I'm having a problem with writing metar strings.
To test this I'm reading the current metar, changing the winds and visibility and writing it back.
This is the write process I am doing:
1. Read the timestamp from C824
2. Write the Command to C800, the ICAO to C808 and the Metar string to B000 in one process call.
3. Read the timestamp again until it's changed (which it does).
The weather does change - the altimeter changes indicating a change in pressure, but the new weather is nothing like the metar string I sent; more like it clears the weather. So I suspect the above process may be wrong. I've tried without writing the command to C800 but then nothing happens at all.
Here's the weather log - My metar write is at 114365 (that's coming through as written by my dll)... At the same timestamp another metar string appears which is completely different. This is more like the weather that actually gets set.
********* FSUIPC4, Version 4.909 by Pete Dowson ********* Running inside FSX on Windows 7 Module base=0D0D0000 User Name="Paul Henty" User Addr="" FSUIPC4 Key is provided WideFS7 Key is provided 250 System time = 01/08/2013 00:19:49 266 FLT UNC path = "\\PJHLAPTOP\Users\Paul\Documents\Flight Simulator X Files\" 312 Trying to connect to SimConnect Acc/SP2 Oct07 ... 344 FS UNC path = "C:\Program Files (x86)\Microsoft Games\Microsoft Flight Simulator X\" 905 LogOptions=00000000 00000001 905 SIM1 Frictions access gained 905 Wind smoothing fix is fully installed 905 G3D.DLL fix attempt installed ok 905 SimConnect_Open succeeded: waiting to check version okay 905 Trying to use SimConnect Acc/SP2 Oct07 5164 Running in "Microsoft Flight Simulator X", Version: 10.0.61472.0 (SimConnect: 10.0.61259.0) 5164 Initialising SimConnect data requests now 5164 FSUIPC Menu entry added 5258 \\PJHLAPTOP\Users\Paul\Documents\Flight Simulator X Files\EGJJ.FLT 5258 C:\Program Files (x86)\Microsoft Games\Microsoft Flight Simulator X\SimObjects\Airplanes\C172\Cessna172SP.AIR 14680 System time = 01/08/2013 00:20:03, Simulator time = 00:19:55 (22:19Z) 14680 Aircraft="Cessna Skyhawk 172SP Paint1" 15679 Starting everything now ... 17005 Advanced Weather Interface Enabled 105707 Sim stopped: average frame rate for last 76 secs = 204.6 fps 111307 LogOptions changed, now 00000000 00000003 111557 Weather Read request (At Aircrft) to area 4: Lat=49.21, Lon=-2.21, Alt=85.6, Req=2 111557 Weather Received (type 4 request, Interpolated): "????&A0 011145Z 00000KT&D0NG 100KM&B-448&D3048 2CU057&CU001FNMN000N 6CI393&CI001FNMN000N 14/04 Q1013 " 111557 WX Received in (0 mSecs), WX request type 4, Lat=49.2070, Lon=-2.2066, Alt=85.6m 113897 Weather Read request (Nr Station) to area 5: Lat=49.21, Lon=-2.21, Alt=0.0, Req=1 113897 Weather Received (type 5 request, Nearest): "EGJJ&A84 191859Z 00000KT&D0NG 27019KT&A1917NG 27024KT&A5917NG 100KM&B-532&D3048 2CU054&CU001FNMN000N 6CI391&CI001FNMN000N 15/05 Q1013 @@@ 66 15 270 19 | 197 15 270 24 | " 113897 WX Received in (0 mSecs), WX request type 5, Lat=49.2070, Lon=-2.2066, Alt=0.0m 113897 >Change: Pressure=1013.0 mb 113897 >Change: Surface wind: to alt=276ft, dir=0T, vel=0.0, gust=0.0, turb=0, shear=0, var=0.0 113897 >Change: Wind layer 1: to alt=19690ft, dir=0T, vel=0.0, gust=0.0, turb=0, shear=0, var=0.0 113897 >Change: Wind layer 2: to alt=22690ft, dir=270T, vel=24.0, gust=0.0, turb=0, shear=0, var=0.0 113897 >Change: Visibility[0]: range=62.1sm (100005m), from=-1460ft, to=8530ft 113897 >Change: Cloud[0]: type=9, from 5700ft to 9700ft (+/- 0ft), cover=2, turb=0, topshape=0 113897 >Change: Precip=0, base=0ft, rate=0, icing=0 113897 >Change: Cloud[1]: type=1, from 39300ft to 39500ft (+/- 0ft), cover=6, turb=0, topshape=0 113897 >Change: Precip=0, base=0ft, rate=0, icing=0 113897 >Change: Temperature[0]: alt=276ft, Day=14.0 C, NightVar=0.0 C, DewPt=4.0 C 113897 Results: FS98 Pressure=1013.1 mb 113897 Results: FS98 Wind0: ground (275ft) to 0ft AGL, dir 0M, vel 0, gust 0, turb 0 113897 Results: FS98 Wind1: 276ft to 19690ft AMSL, dir=0T, vel 0, gust 0, turb 0 113897 Results: FS98 Wind2: 19690ft to 22690ft AMSL, dir=270T, vel 24, gust 0, turb 0 113897 Results: FS98 Vis: range =62sm, (raw value=6214) 113897 Results: FS98 Cloud1: type=9, from 5700ft to 9700ft (+/- 0ft), cover 2, turb 0, ice 0 113897 Results: FS98 Cloud2: type=1, from 39300ft to 39500ft (+/- 0ft), cover 6, turb 0, ice 0 113897 Results: FS98 Temp0: to 276ft, Day 14.0C, NightVar 0.0C 113897 Results: FS98 CurrTemp at PlaneAlt=279: 14C 114365 Setting Metar: "EGJJ&A84 191859Z 06015KT&D0NG 06015KT&A1917NG 06015KT&A5917NG 50KM&B-532&D3048 2CU054&CU001FNMN000N 6CI391&CI001FNMN000N 15/05 Q1013 @@@ 66 15 270 19 | 197 15 270 24 | " 114365 NW_SETEXACT weather command received, ICAO=EGJJ 114365 >NewSet: **** New Weather being set: ICAO=EGJJ (Dyn=0) 114365 >NewSet: Pressure=0.0, Drift=0.0 114365 >NewSet: Visibility[0]: range=0.0sm (0m), from=0ft, to=0ft 114365 >NewSet: **** End of New Weather details for ICAO=EGJJ 114365 Setting Weather: "EGJJ 011145Z 00000KT 0050&B-84&D1 CLR 15/07 Q0000 " 114427 Weather Mode now = Custom 116221 Weather Read request (Global set) to area 1: ICAO="GLOB", Req=0 116221 Weather Received (type 1 request, (null)): "GLOB&A0 000000Z 00000KT&D985NG 27020KT&A2001NG 27025KT&A6001NG 100KM&B-448&D3048 2CU057&CU001FNMN000N 6CI393&CI001FNMN000N 15/05 Q1013 @@@ 66 15 270 20 | 197 15 270 25 | " 116221 WX Received in (0 mSecs), WX request type 1, ICAO=GLOB 116221 >Change: FS98 Pressure=846.0 mb 116221 >Change: FS98 Wind1: 276ft to 19690ft AMSL, dir=0T, vel 0, gust 0, turb 0 116221 >Change: FS98 Wind2: 19690ft to 22690ft AMSL, dir=270T, vel 24, gust 0, turb 0 116221 >Change: FS98 Vis: range =62sm, (raw value=6214) 116221 >Change: FS98 Cloud1: type=9, from 5700ft to 9700ft (+/- 0ft), cover 2, turb 0, ice 0 116221 >Change: FS98 Cloud2: type=1, from 39300ft to 39500ft (+/- 0ft), cover 6, turb 0, ice 0 116221 >Change: FS98 Temp0: to 276ft, Day 14.0C, NightVar 0.0C 116221 >Change: FS98 Dewpoint Control: disabled 116221 >Change: FS98 Precip Control: disabled 116221 Results: FS98 Pressure=846.0 mb 118561 Weather Read request (Nr Station) to area 5: Lat=49.21, Lon=-2.21, Alt=0.0, Req=1 118561 Weather Received (type 5 request, Nearest): "EGJJ&A84 011145Z 00000KT&D304NG 0050&B-84&D1 CLR 15/07 Q0846 " 118561 WX Received in (0 mSecs), WX request type 5, Lat=49.2070, Lon=-2.2066, Alt=0.0m 123148 LogOptions changed, now 00000000 00000001 137188 System time = 01/08/2013 00:22:06, Simulator time = 13:45:46 (11:45Z) 137188 *** FSUIPC log file being closed Average frame rate for running time of 95 secs = 196.0 fps G3D fix: Passes 1119, Null pointers 0, Bad pointers 0, Separate instances 0 Memory managed: 48 Allocs, 48 Freed ********* FSUIPC Log file closed ***********
I'd be grateful for any pointers...
Thanks,
Paul
-
Welcome to the "FSUIPC Client DLL for .NET" Sub-forum.
Firstly, thanks to Pete Dowson for giving us a corner of his support forum. This will make things much easier than the very long single thread.
The latest download package with introductory information about the DLL is found in the 'sticky' thread at the top of this sub-forum.
This forum is only for support questions regarding the use of my .NET DLL for talking to FSUIPC.
I am not an expert in the use of FSUIPC or what the offsets mean or do. All general FSUIPC questions and queries about offsets should be directed to Pete Dowson in the main FSUIPC support forum (the one above this).
If you are posting a 'how to' question please let me know if you want the answer in VB or C#.
If you want help with a bug or non-working code please post the relevant code AND the offset declarations. I cannot help without these. If you are getting an exception please post the text.
Thanks,
Paul
-
Extra Documentation for the new 2.4 Features - C# (VB.NET Above)
Reading Payload and Fuel data
The payload and fuel data is accessed from a new property on the FSUIPCConnection class called 'PayloadServices'.
It's a good idea to assign this to a local variable to save typing FSUIPCConnection.PayloadServices all the time...
// Get a reference to save so much typing... PayloadServices ps = FSUIPCConnection.PayloadServices;
Whenever you want to get the latest payload and fuel data you just need to call RefreshData() on the PayloadServices object...
// Get the latest data from FSUIPC ps.RefreshData();
Once you have done that there are lots of properties on the PayloadServices class that will give you weight and fuel data for the aircraft overall. For example:
ps.PayloadWeightLbs ps.AircraftWeightLbs ps.FuelWeightLbs ps.FuelCapacityUSGallons
Most are self-explanatory and there is Intellisense on everything.
You also have access to the individual payload stations and fuel tanks. Here is an example of iterating through each payload station.
// Go through each station and get the name and weight foreach (FsPayloadStation payloadStation in ps.PayloadStations) { string name = payloadStation.Name; double weight = payloadStation.WeightLbs; }
You can do the same thing with the FuelTanks collection. This holds a FsFuelTank object for each possible tank. Some might not be in use for the current aircraft though.
To access properties on a specific fuel tank you can use the following syntax: (This gets the current level of the Centre_Main tank in US Gallons. Other properties and tanks are available.)
ps.GetFuelTank(FSFuelTanks.Centre_Main).LevelUSGallons
Changing Payload and Fuel
You can also write new values to individual payload stations or fuel tanks.
To change the value of payload station, just change one of the weight properties for that station (Lbs, Kg etc).
When you've made all the changes you want call WriteChanges() on your PayloadServices object. This will send all the changed values to FSUIPC.Fuel Tanks
Fuel tanks are the same except, in addition to changing the weight, you can also change them by setting a new % full level or a volume in Gallons or Litres.
Note that WriteChanges() send both Payload and Fuel changes in one go.
Here's some code to set all the payload stations to 0
// Get the latest values ps.RefreshData(); // Go through each station and set weight to 0 foreach (FsPayloadStation payloadStation in ps.PayloadStations) { payloadStation.WeightLbs = 0; } // send the changes to FSUIPC ps.WriteChanges();
User Input - Menus
Below is code for a form that puts two menu items on the FS menu and responds to the user selecting these menu items.
This shows you everything you need to do. If you want to put this behind a real form to see it working, you'll need two timers on the form called 'timerPollForInput' and 'timerKeepAlive'. You'll also have to change the namespace and class name to suit your project and wire up the _load event.
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Text; using System.Windows.Forms; using FSUIPC; namespace TestApp { public partial class MenuDemo : Form { UserInputServices ui; public MenuDemo() { InitializeComponent(); } private void MenuDemo_Load(object sender, EventArgs e) { // Open FSUIPC FSUIPCConnection.Open(); // Get a reference to the UserInputServices ui = FSUIPCConnection.UserInputServices; // Add our Menu Items // The first parameter is the key and can be whatever you want. // We'll use this later to see which item was chosen. // The second parameter is the text to display on the menu item. // Putting an & before a letter makes it underlined and becomes the shortcut key. // Set the last parameter to true is you want to FS to pause when the user chooses this item. ui.AddMenuItem("MenuA", "Our Menu Item &A", false); ui.AddMenuItem("MenuB", "Our Menu Item &B", false); // Sink the MenuSelected event so we can respond ui.MenuSelected += new EventHandler<UserInputMenuEventArgs>(ui_MenuSelected); // Start our two timers. // This one keeps FSUIPC from deleting our menu items (every 4 seconds) this.timerKeepAlive.Interval = 4000; this.timerKeepAlive.Tick += new EventHandler(timerKeepAlive_Tick); this.timerKeepAlive.Start(); // This one checks to see if the user has selected a menu item (2 times a second). this.timerPollForInput.Interval = 500; this.timerPollForInput.Tick +=new EventHandler(timerPollForInput_Tick); this.timerPollForInput.Start(); } private void timerPollForInput_Tick(object sender, EventArgs e) { // Check if the user has selected our menu items ui.CheckForInput(); } private void timerKeepAlive_Tick(object sender, EventArgs e) { // Stop FSUIPC from deleting out menu items ui.KeepMenuItemsAlive(); } private void ui_MenuSelected(object sender, UserInputMenuEventArgs e) { // This method is called when the user selects one of our menu items. // Check which one and do stuff switch (e.ID) { case "MenuA": // Do menu A stuff here MessageBox.Show("Menu Item A selected!"); break; case "MenuB": // DO menu B stuf here MessageBox.Show("Menu Item B selected!"); break; } } private void Form2_FormClosing(object sender, FormClosingEventArgs e) { // Remove our menu items from FS ui.RemoveAll(); // Close FSUIPC FSUIPCConnection.Close(); } } }
User Input - Buttons and Keys
Similar to above except you use AddKeyPress() and AddJoystickButtonPress() to register the buttons/keys.
You then sink the KeyPressed or ButtonPressed events to detect the user pressing the button/key.
You still need to call the CheckForInput() regularly but KeepMenuItemsAlive() is obviously not required for buttons/keys. -
Extra Documentation for the new 2.4 Features - VB.NET (C# Below)
Payload and Fuel data
The payload and fuel data is accessed from a new property on the FSUIPCConnection class called 'PayloadServices'.
It's a good idea to assign this to a local variable to save typing FSUIPCConnection.PayloadServices all the time...' Get a reference to save so much typing... Dim ps As PayloadServices = FSUIPCConnection.PayloadServices
Whenever you want to get the latest payload and fuel data you just need to call RefreshData() on the PayloadServices object...
' Get the latest data from FSUIPC ps.RefreshData()
Once you have done that there are lots of properties on the PayloadServices class that will give you weight and fuel data for the aircraft overall. For example:
ps.PayloadWeightLbs ps.AircraftWeightLbs
Most are self-explanatory and there is Intellisense on everything.
You also have access to the individual payload stations and fuel tanks. Here is an example of iterating through each payload station.
' Go through each station and get the name and weight For Each (payloadStation As FsPayloadStation In ps.PayloadStations) Dim name As String = payloadStation.Name Dim weight As Double = payloadStation.WeightLbs Next payloadStation
Changing Payload and Fuel
You can also write new values to individual payload stations or fuel tanks.
To change the value of payload station, just change one of the weight properties for that station (Lbs, Kg etc).
When you've made all the changes you want call WriteChanges() on your PayloadServices object. This will send all the changed values to FSUIPC.Fuel Tanks
Fuel tanks are the same except, in addition to changing the weight, you can also change them by setting a new % full level or a volume in Gallons or Litres.
To access properties on a specific fuel tank you can use the following syntax: (This gets the current level of the Centre_Main tank in US Gallons. Other properties and tanks are available.)
ps.GetFuelTank(FSFuelTanks.Centre_Main).LevelUSGallons
Note that WriteChanges() send both Payload and Fuel changes in one go.
User Input - Menus
1. First, declare a UserInputServices object using the WithEvents modifier
' Declare a UserInputServices object so we can use it in the code Private WithEvents userInput As UserInputServices
2. After opening the FSUIPC connection you need to set the variable above and add your menu items:
' Set our userInput object to the UserInputServices userInput = FSUIPCConnection.UserInputServices ' Add our menu items (2 examples) ' First parameter is the ID we use to identify this menu item ' Second parameter is the Text for the menu item (Max 30 chars) ' use & before a letter to make it the shortcut key ' Third parameter specifies whether or not to pause FS when ' the menu item is selected. userInput.AddMenuItem("Menu1", "Menu Item &One", False) userInput.AddMenuItem("Menu2", "Menu Item &Two (Pauses FS)", True)
Note that if you use a pausing menu item it will be your application's responsibility to unpause FS by writing to offset 0626 at the appropriate time.
3. You need add a new method to handle the MenuSelected event. This will be called when the user selects one of your menu items:
Private Sub menuItemSelected(ByVal sender As Object, ByVal e As UserInputMenuEventArgs) Handles userInput.MenuSelected ' This sub gets called when a menu item is selected ' Use the e object to see which one (Check the ID) Select Case e.ID Case "Menu1" MessageBox.Show("Menu item 1 selected") Case "Menu2" MessageBox.Show("Menu item 2 selected") End Select End Sub
4. You need to call CheckForInput() regularly to check if the user has selected any menu items. If they have the above method above will automatically be called for you. Add this code in one of your timers.
' Check for input. Recommended every 200ms userInput.CheckForInput()
5. FSUIPC will delete your menu items after about 14 seconds. To prevent this you must tell FSUIPC that your application is still running and stills needs the menu items. You must call KeepMenuItemsAlive() regularly. Pete suggests every 8 seconds. So on an 8 second timer call:
userInput.KeepMenuItemsAlive()
6. Before your application exits, call the RemoveAll() method to clean up your menu items.
' Remove our menu items userInput.RemoveAll()
User Input - Buttons and Keys
Similar to above except you use AddKeyPress() and AddJoystickButtonPress() to register the buttons/keys.
You then sink the KeyPressed or ButtonPressed events to detect the user pressing the button/key.
You still need to call the CheckForInput() regularly but KeepMenuItemsAlive() is obviously not required for buttons/keys. -
Full Feature List
PayloadServices
Makes it easy to access the payload stations and fuel tanks on the player's aircraft. The DLL supplies you with a .NET List<> of payload or fuel tank objects. Weights and fuel tank levels are supplied in a number of units (percent full, Lbs, Kg, Slugs, Newtons, Gallons, Litres).
The DLL also supplies calculated data such as Zero Fuel Weight and total aircraft weight.Individual fuel tank levels can be changed using %, Gallons, Litres, Lbs, Kgs, Newtons or Slugs.
Individual Payload station weights can be changed using Lbs, Kgs, Newtons or Slugs.UserInputServices
Makes it easy to use the FSUIPC user input facilities to trap key presses and joystick button presses from inside Flight Sim.
Allows you to add your own menu items to the 'Modules' (<=FS9) or 'Add Ons' (FSX) menu. You can respond to the user selecting these menu items in your own application.Offset Grouping
Make groups of offsets that you can process at different times in your application.
AI Traffic
Make it very easy to access the AI Traffic Information from FSUIPC. AI Planes are returned to you as a strongly-typed List<> of AIPlaneInfo instances. AIPlaneInfo provides all information available from FSUIPC plus lots of extra info (e.g. Distance and Bearing from the player) in a ready-to-use .NET class.
You can also write into the internal FSUIPC AI Traffic Tables. (This does not add AI planes into flight sim, it just adds TCAS targets).Longitude and Latitude Helper Classes.
These understand the raw FS Units provided by FSUIPC (both the 8-Byte and 4-Byte types) so conversion to and from degrees is done for you. Also has a very flexible ToString() method to enable output in a number of human-readable formats.
Classes exist for dealing with Longitude and Latitude Spans (distances). These classes can be used to convert Lon/Lat spans to and from metres or feet. Lon/Lat spans can be calculated between two Lon/Lats.
Another class deals with a Lon/Lat points. This represents a point on the Earth but can also be used to calculate the distance and/or bearing to another point.
Another class represents a Quadrilateral area on the Earth. Its primary function is to test if a lon/lat point is contained within the Quadrilateral. This is useful for testing if you are on a runway. The Quadrilateral can be generated from a set of four Lat/Lon points, or from basic runway information such as that found in the output of MakeRunways.exe by Pete Dowson.Connect to Multiple Instances of WideClient
The DLL is fully capable of talking to multiple instances (Classes) of WideClient on the same machine. This is an advanced feature that enables applications to control multiple copies of FS on the same network.
-
.NET DLL for communicating with FSUIPC
Attached is Version 2.4 of my FSUIPC Client DLL for .NET
Benefits:- Object-Oriented class interface better suited to .NET than the other procedural .NET SDKs.
- Data from FSUIPC is returned directly to your .NET variables. Supported types are:
Byte, Int16, Int32, Int64, UInt16, UInt32, UInt64, Double, Single
String (Unicode/ASCII conversion and termination handled for you)
BitArray (Allows you to easily manage the bit field type offsets)
Array of Bytes (Allows you to read and write raw blocks of data if you need that level of control.)
- Writes are handled just by assigning a new value to the local .NET data variable. The write to FSUIPC will be handled automatically during the next Process().
- Errors are handled by .NET exceptions, not by checking return values.
- The DLL can be use with any .NET language. Documentation and example projects for C# and Visual Basic.NET.
- The library has full internal documentation that will appear on the IntelliSense popup menus in Visual Studio.
- Just as fast as the procedural-style .NET SDKs.
- Thread safe. Can be used in multi-threaded applications, with each thread communicating with FSUIPC, without corrupting the data. (Only one thread can talk to FSUIPC at a time – other threads will block until the connection is free).
The zip file contains the DLL, documentation and an example project in C# and Visual Basic.NET (Visual Studio 2010 format).
Documentation for the 2.4 features is only in the form of example code which is attached below.
Microsoft have free versions of Visual Studio 2012 compilers for VB, C#, C++ here:
http://www.microsoft.com/visualstudio/eng/products/visual-studio-express-for-windows-desktop#product-express-desktopPaul
-
This thread will soon be locked.
All support questions from now on should be new threads in the "FSUIPC Client DLL for .NET" subforum of Pete's FSUIPC forum.
Thanks to Pete for kindly giving us our own sub forum. This should make things more manageable than this very long thread.
-
Hey guys, would it be possible in getting some help on terms of reading the Metar weather in VB, I've tried a few various ways but I cant seem to get it to work at all
Also - Is there a way through FSUIPC to tell what version of flight sim is running? I didn't know if it might be able to differentiate between FS2004 and FSX
Cheers
Dan
Using the New Weather Interface or the Metar read/write is not simple, especially since at the moment the DLL doesn't allow you to read/write structures (You'd need to pack/unpack the structures to/from byte arrays which isn't pretty) . It would take me hours to work this out and I'd rather spend the time adding easy weather handling into the version 3.0 of the DLL. It's been a while since the last official 2.0 release so I'm going to get 3.0 done by the end of August. It's mainly going to be a weather update.
If you're interested in being a beta tester for this let me know on a private message. (Also anyone else reading this).
To tell what version of the flight sim you are connected to, just check the property on FSUIPCConnection called FlightSimVersionConnected. It returns an enum which you can convert to a string using ToString() if you want to print it directly. E.g.
MessageBox.Show(FSUIPCConnection.FlightSimVersionConnected.ToString())
Paul
-
Slightly OT but are you the same Paul that composes/records music as I have seen a few posts/vids elsewhere and he certainly looks like you :razz:
Yes, it's probably me. There is another Paul Henty who does music under the name Lynskey which is not me, but he doesn't tend to use his real name.
Paul
-
Hi Guys, Just wondered with this DLL if there is a way to check 2 things:
1. A way to check If the engines are On or Off
2. A way to check the Metar information within FSX
Hi Dan,
The DLL gives you access to all information provided by the FSUIPC interface. Details can be found by downloading the FSUIPC SDK and looking in the document called "FSUIPC4 Offset Status.pdf".
To answer your specific questions:
1. Yes, but it doesn't look like it's just one offset. I think you'll need to check a few because different types of engine (reciprocating, turbine etc) have this data in different places.
2. Yes, an extended FSX METAR string can be retrieved for an airport or lon/lat location. See offset B800 in the documentation I referred to above.
Paul
-
Hi Ruediger,
My suggestion is to use the grouping facilities so that you process all the user offsets in one go.
First, you'll need to keep a List<> of the user offsets you create for later use, so declare one at the form (or class) level:
Private UserOffsets As List(Of Offset(Of UShort)) = New List(Of Offset(Of UShort))
Next, you'll need a method that will take your array of user offset addresses and create the new offsets (assigning them to their own group).
Private Sub updateUserOffsets(userOffsetAddresses As String()) ' Clear the user offsets list UserOffsets.Clear() ' Delete the user offsets group FSUIPCConnection.DeleteGroup("UserOffsets") ' For each user offset, make a new Offset in the "UserOffsets" group and add it to ' a list (UserOffsets) for later use. For Each OffsetAddress As String In userOffsetAddresses Dim UserOffsetAddress As Integer = Integer.Parse(OffsetAddress, Globalization.NumberStyles.AllowHexSpecifier) Dim User_Offset As Offset(Of UShort) = New FSUIPC.Offset(Of UShort)("UserOffsets", UserOffsetAddress) UserOffsets.Add(User_Offset) Next End Sub
You can call this when your application starts or when the user changes the list of offsets in the application.
When you need to process them, you can just process the entire group at once and then step through each Offset and do whatever you need to with the values:
' Process the user offsets FSUIPCConnection.Process("UserOffsets") ' Now do stuff with the data in the user offsets For Each UserOffset As Offset(Of UShort) In UserOffsets Dim val As UShort = UserOffset.Value ' process the data ' ... Next
Paul
-
I can't create Forums or even Subforums by myself, but I do think at least a Subforum here would be worthy. I can ask SimFlight to set that up if Paul agrees, and transfer this complete thread over when done.
I think a subforum here would be good as this thread is getting a bit long now but whenever you have time, there's certainly no rush.
Thanks,
Paul
Write programm on C#
in FSUIPC Client DLL for .NET
Posted
I can't see anything wrong there. Please show me:
1. Where you declare playerLongitude and playerLatitude
2. What does appear in the text boxes?
You can also try inspecting the value of playerLatitude.Value while the program is running using a breakpoint.
Paul