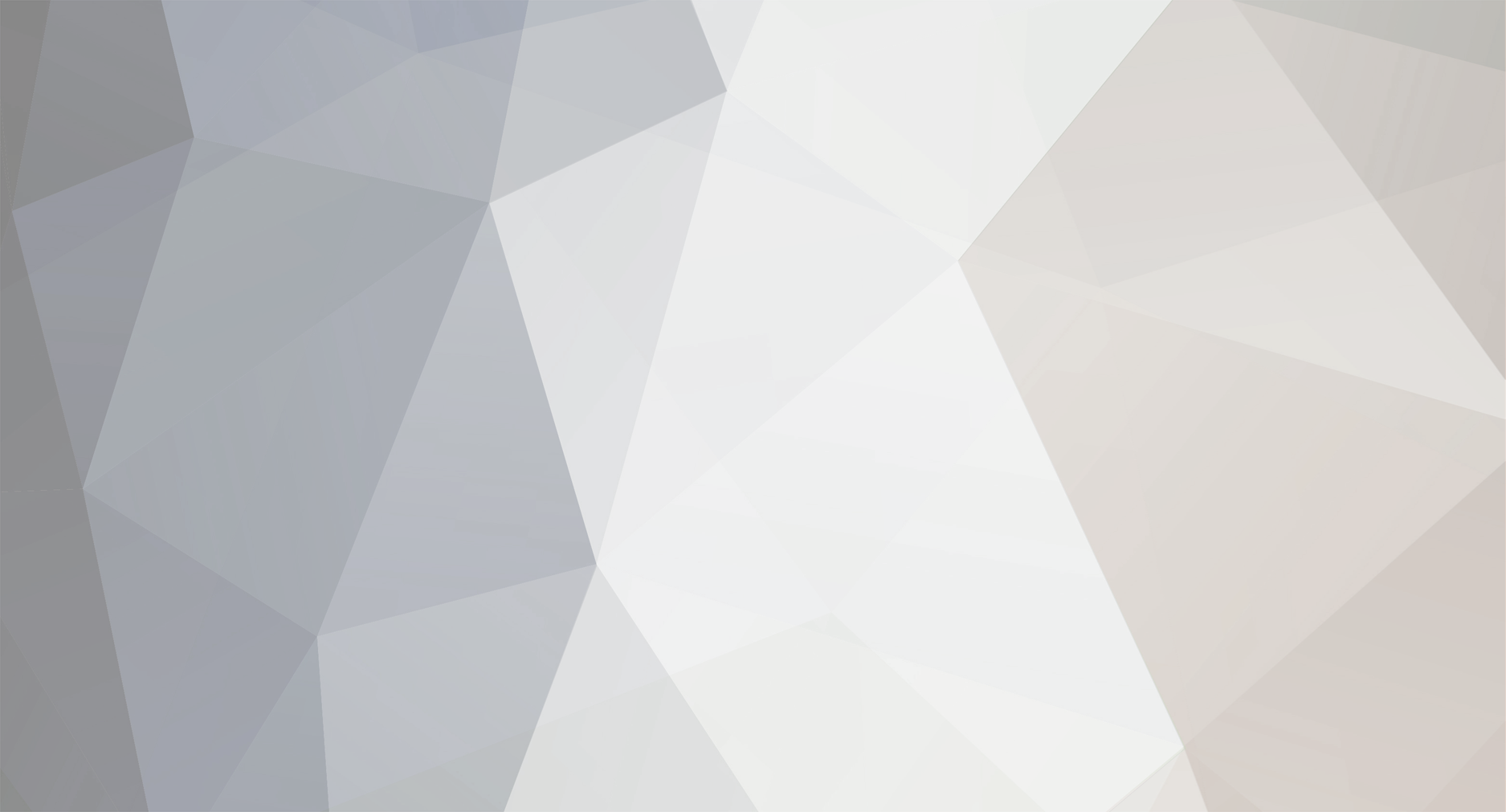
Paul Henty
-
Posts
1,652 -
Joined
-
Days Won
74
Content Type
Profiles
Forums
Events
Gallery
Downloads
Posts posted by Paul Henty
-
-
Can you put an example table in VB .NET with the report of parking brakes? For any time that I put the parking brakes creates a line in the table with a colum: Like Name: Parking Brakes SET.
Hi,
I'm away at the moment so I can't give you complete code but this might point you in the right direction...
I think what you want is to detect when the parking brake changes and write a log entry.
To do this, you need to keep a note of what the last value was, so set up a variable to hold this in addition to the offset:
Dim parkBrakes as Offset(Of UShort) As New Offset(Of UShort)(&HBC8)
Dim lastParkBrakes as Boolean[/CODE]In your main timer you need to check this last state with the current one. If it's different then add your log entry. (I've just put a dummy method here for the log entry).
In the Timer_Tick()
[CODE]
FSUIPCConnection.Process()
Dim currentParkBrakes as Boolean
currentParkBrakes = (parkBrakes.Value > 0)
If currentParkBrakes <> lastParkBrakes Then
lastParkBrakes = currentParkBrakes
Dim Message as String = IIF(currentParkBrakes, "Parking brake ON", "Parking Brake OFF")
WriteLogEntry(Message)
End If[/CODE]This will run every tick waiting for the value to change. When it does, it write the log and waits for the next change.
PD: I´m thinking do it with a rich textbox. I think is easier. How Can I put the dates here? With a while?
To handle the date, it depends how you record the log:
1. For a text box (or rich text box) just get the string value of the current date and time using one of the built-in formats (I suggest using "G"):
[CODE]DateTime.Now.ToString("G")[/CODE]and add this into the message text.
2. For a memory Table just create a column with type DateTime and put the current date and time in:
[CODE]Dim newRow as DataRow = MyLogTable.NewRow()
newRow("EntryDateTime") = DateTime.Now[/CODE]Hope that helps. If you need any more help just ask...
Paul
-
1
-
-
But the code number isn´t all like the PDF. In some offsets the number it is without &H and in other &H is the same than 0. Why?
In Visual Basic hexadecimal numbers are written with &H in front of them. The offsets in the PDF are all given in hexadecimal so that's why the &H is needed.
VB also deletes any leading 0s because they are technically not needed. For example 0570 is the same number as 570. So in VB this appears as &H570 (Even if you type in &H0570, the code editor will delete the first 0).
Just put '&H' in front of the offset given in the PDF. If the code editor deletes the leading 0 don't worry about it.
Paul
-
Just checked, I have v2.3Beta which I downloaded from this thread but in VS.2012 the FSUIPCClient.dll from 2.3 shows runtime v2.0.50727
Yeah that would be for .NET 2.
Attached is 2.3 but compiled for the .Net 4 runtime.
Let me know if this doesn't work and I'll see if I can tweak anything.
Paul
-
Could you tell me for which .NET platform it was compiled AND which System.dll version it is referencing?
The normal one available at the start of this thread targets the .NET 2 framework.
In December 2011 I sent you a DLL (version 2.2) via PM that targets the .NET 4.0 framework. I don't know which one you are using.
I can make you another for .NET 4 if you like (will be 2.3). Looking at my system, the version info for the System.DLL will be:
Version = 4.0.0.0
Runtime Version = v4.0.30319
Specific Version = False
Paul
-
I don't know the answer to this. You'll be better of posting in the main support forum for Pete to answer.
-
It would be nice if the next release of FSUIPC.NET Client came with both an x86 DLL as well as an Any CPU dll.
I'm not sure this is possible. The .NET Client DLL must be x86 or it will not be able to communicate with FSUIPC. The communication process uses windows messages and shared memory.
Because FSUIPC is running inside Flight Sim which is a 32-bit process, my understanding is that only another 32-bit process can send windows messages to it and access the same memory. This makes sense because 32-bit software is running inside a virtual machine on the 64-bit windows so it has it's own separate virtual memory.
I think the only communication possible would be those than can cross machine boundaries like DCOM and TCP/IP etc. But FSUIPC doesn't use these.
Paul
-
I guess you'll need to wait for Pete's return next week then.
In the mean time you could post the contents of your FSUIPC.log file (from your flight sim modules folder) here. It might point you or someone in the right direction and Pete usually asks to see it anyway.
Paul
-
Check the date on your PC is set correctly. A common cause for this problem is that the date on the PC is set earlier than your purchase date.
Paul
-
...
But in textbox is V/S in airborne... it´s always updating, but i want just record v/s at touchdown.. thank you very much.
You've slightly misunderstood about the OnGround flag. You don't set this yourself. It's updated by FS when the aircraft is on the ground.
The 030C is constantly updated while in the air. As soon as the plane touches down this value gets 'frozen'. So this value will be the last vertical speed as the wheels touched down. When the plane takes off again this offset gets updated again.
What you need to do is READ the OnGround flag until it's 1 (plane has touched down). Then you can read the 030C. This will now contain the Vertical Speed at touch down.
so something like this in your timer...
if (this.onGround.Value == 1) { double TouchdownRate = ((double)touchdown_rate.Value * 60D * 3.28084D / 256D); this.txttouchdown.Text = TouchdownRate.ToString(); } else { this.txttouchdown.Text = "Airborne"; }
Let me know if you need any more help.
Paul
-
ADF1 IDENTITY is at 303E.
Paul
-
I want to read the pitch and roll in visual basic 2010.
To use any offset you need to follow this procedure: (This example will use Pitch)
1. Look in the "FSUIPC4 Offsets Status.pdf" (from the SDK) for the offset address. A search for "Pitch" finds 0578.
2. Get the size from the size column (in this case 4 bytes).
3. Read the description to see if a data type is mentioned and if the data is signed. (There is no data type mentioned in this case so we assume some kind of integer type. We can also read that the data is signed).
4. From the table on page 7 of my UserGuide.pdf we read that an integer type of size 4 that is signed corresponds to the VB.NET type of 'Integer'. Thus we declare the offset:
Dim Pitch As Offset(Of Integer) = New Offset(Of Integer)(&H578)[/CODE]
5. After the Process() call, when we want to use the data, we use the conversion described in the "FSUIPC4 Offsets Status.pdf" document to get the data into human readable format.
[CODE]
Dim PitchDegrees As Double
PitchDegrees = Pitch.Value * 360.0# / (65536.0# * 65536.0#)[/CODE](The # signs are appended to the values to tell the compiler that these are to be Doubles and not Integers.)
You can now apply this process to any offset you want and know how to use it. Roll (also called Bank) is at 057C.
Paul
-
Please let us know if the reason we can only see the About and Loggin tab is because the add-on is not registered or not, or if there is an error somewhere. Thanks...
The other tabs are only available after you register.
Paul
-
For a motion platform the data you need will be accelerations relative to the body of the aircraft. How many pieces of data you take will depend on how complicated you want to make the algorithm to determine the position of the motion platform.
The most basic data I would use for 2 degrees of freedom (roll and pitch only) is:
3070 for the longitudinal accelerations (e.g. takeoff/braking)
3078 for the pitch accelerations
3080 for the roll accelerations
If you have 3 degrees of freedom (up/down as well) then you need to add:
3068 for the vertical accelerations
Paul
-
Firstly, programming with FSUIPC is quite advanced. It's not a good place to learn about programming. I would advise you learn a bit about Visual Basic programming in general before you try to use FSUIPC.
Everything you need to program with FSUIPC in Visual Basic 2010 is contained in two downloads:
1. The file called "FSUIPC4 Offsets Status.pdf" from FSUIPC SDK. This lists all the offsets available and gives important information about how to deal with the data from each offset.
2. My FSUIPC Client DLL package from here:
http://forum.simflig...net-version-20/
This contains the DLL, an extensive user guide that explains how to get data from FSUIPC, a reference manual and a sample project (in VB and C#). The sample project contains many examples of reading and writing data to/from FSUIPC and has lots of comments telling you what each line in doing.
I suggest reading the "getting started" section of the user guide and then run the sample project. Then look at the code in the sample project and see how it's done. Most of the code examples in the user guide are taken from the sample project.
If you need any more help with my DLL or getting data from FSUIPC feel free to ask. It's always helpful when asking questions to paste in any error messages and the code that's failing. Without these it's almost impossible to offer any help.
Paul
-
I tried the sample of FSUIPCDotNetClient2.0 but I got only errors.
I tried the VB6 and strings in FSUIPC (in the file FSUIPC_SDK) but I got the error "Statement is not valid in a namespace"
Hi,
The VB6 stuff won't work in .NET, that's why I wrote my .NET DLL from scratch.
What are the errors you are getting with the DLL? Have you tried building the project or running it? Until you build it at least once there will be errors reported by the code editor because the zip file doesn't include any binaries.
If you can't run the project then let me know what the errors are and I'll help you get it working.
Paul
-
The Problem is Visual Basic 2010 can´t open VB6 files. And so it is a little bit difficult. But I hope I find a way to convert the files.
I recommend that you use my FSUIPC Client DLL for .NET languages. It's much more suited to the way things are usually done in .NET. The package also includes a sample project in Visual Basic.
You can download it from here:
http://forum.simflight.com/topic/40989-fsuipc-client-dll-for-net-version-20/
Paul
-
1
-
-
I tried with ATR of Flight One, Wilco Airbus and iFly 737 NGX.
As Graham says, some paid aircraft do not use the underlying Flight Sim systems, they write their own. With these kind of aircraft it's not possible to use FSUIPC and therefore my DLL to access the data. You'll need to investigate how to access the lights for each paid aircraft individually. This could be through their own SDK or using L:VARS etc. There are many ways that can be used but each plane is different. With some planes it may not be possible at all.
It's probably best to ask in the support forums of makers of the aircraft.
Paul
-
Hi Dave,
What's going wrong is that you're declaring the offset variables every time the timer ticks in Poll_Timer_Tick.
This is bad because you're creating new instances of the offset requests every time the timer ticks. Even though your variables go out of scope after the method ends they are still registered with the FSUIPCClient DLL,
So each tick adds 4 new offsets to the list of offsets being requested by the DLL. After 30 seconds your program is actually requesting 400 offsets. After a while you'll exceed the total amount of data that can be handled by FSUIPC and you get the FSUIPC_ERR_SIZE exception.
When working with the DLL only declare (Dim) the offsets once during the lifetime of the application. The easiest way to do this is to place the declarations at the form or class level, rather than in the timer loop. Like this:
Dim airSpeed As Offset(Of Integer) = New FSUIPC.Offset(Of Integer)(&H2BC) Dim altAltitude As Offset(Of Integer) = New FSUIPC.Offset(Of Integer)(&H7D4) Dim altRate As Offset(Of Short) = New FSUIPC.Offset(Of Short)(&H7F2) Dim altHead As Offset(Of Short) = New FSUIPC.Offset(Of Short)(&H7CC) Private Sub Poll_Timer_Tick(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Poll_Timer.Tick FSUIPCConnection.Process() Dim altALT As Double = (altAltitude.Value / 65536D) * 3.28084D Dim altHDG As Double = (altHead.Value / 65536D) * 360D Me.TxtAPALT.Text = altALT.ToString("f0") Me.TxtAPRate.Text = altRate.Value.ToString("f0") Me.TxtAPHDG.Text = altHDG.ToString("f0") End Sub
I've just had a look at the manual and it doesn't really make this clear which is my fault. I'll have to amend it in future releases. Sorry to dash your expectations but it turns you are not an idiot after all. :smile:
Paul
-
I am unable to simulate more planes, i do not know what i am doing wrong.
Next offset i am choosing 0x1F80+sizeof(TCAS_DATA),&
Am i missing anything
Hi,
You need to write every plane to 0x1F80. You don't add sizeof(TCAS_DATA) every time. You can write multiple planes to 0x1F80 before calling Process(). Even though they all have the same offset they won't overwrite each other because offsets do not refer to real memory locations. They are just tokens that tell FSUIPC what to do with the data.
Paul
-
the value of 10 for P3D has been defined in the both the "FSUIPC for Programmers" and the "FSUIPC4 Offsets Status" documents for a while now.
...
As well as the documents mentioned above, the README.TXT included in the SDK says this:
...
Sorry if this is not clear enough. I'll change the .h file for C/C++ now.
That's fair enough Pete. It's very clear, I just didn't think to look anywhere other than the .h file for these values.
Now I know where this info is likely to be I'll be able to find it in the future.
Thanks,
Paul
-
Uhm in FSUIPC_SDK in readme ... tell Prepar3D it is 10 .... or i wrong ?!?
You're right.
Attached is a new DLL (version 2.3) with the new enum value for Prepar3d. It also has extra overloads on Open() to pass an integer.
You need to copy over your existing DLL and XML files. (Make backups first in case you need to go back).
Paul
-
i mean that when i call open API in c# i should pass it an Enum (FSX ESP ...) this enum is a number (9 for ESP) but for connect to prepar3d i should pass 10 in FSUIPC ... i can pass an integer to your OpenConnection API?
OK I understand.
How do you know Prepar3d is 10? If I look at the current FSUIPC SDK (February 2012) the FSUIPC_User.h file only goes up to ESP (9).
I can only keep my DLL in line with the current SDK. If you can point me to an official source that says Prepar3d = 10 then I'll happy to amend my DLL.
At the moment you can just pass 'Any' for the enum, or just don't pass any parameters into Open() and it will connect.
You cannot pass an integer at the moment, but I'll think about adding that.
Paul
-
One question how i can use FSUIPClient for connect with Prepar3d?
I don't think there would be anything special or different about connecting to FSUIPC running inside Pepar3d. It should be the same as connecting to FSUIPC running in FSX or FS9. The FSUIPC interface is the same.
If you've tried to connect to Prepar3d but cannot, then please describe the problem. Are there any error messages?
Paul
-
Nevertheless I always stumble over the speed vertical ( 0x02C8 ) and the height.
Here is the code to read and display the vertical speed and height. I've included the conversions for metres and feet.
Declare the offsets as follows:
private Offset<int> verticalSpeed = new Offset<int>(0x02C8); private Offset<long> altitude = new Offset<long>(0x0570);
Get the values (after the Process()) like this:
double verticalSpeed_MetresPerSecond = (double)verticalSpeed.Value / 256d; double verticalSpeed_FeetPerMinute = (double)verticalSpeed.Value * 60d * 3.28084d / 256d; double altitude_Metres = (double)altitude.Value / (65536d * 65536d); double altitude_Feet = (double)altitude.Value / (65536d * 65536d) * 3.28084d;
I do not arrive has to have a flow of dated fluids: my servos is jerky, would have you an idea?The first thing to look at would be the interval for 'timer1'. This sets how often the timer1_Tick() method runs. If you want it smooth you need something like 20 ticks per second. This is an interval of 50ms. So set the timer1.Interval property to 50.
If you used my example application as a starting point, the interval is set to 200 in the method openFSUIPC(). This is only 5 times per second so it will be jerky. This is the line you should change.
Paul
Global weather in FSX Dotnet client 2.0
in FSUIPC Support Pete Dowson Modules
Posted
You can (and should) reuse it. Just remember that if the connection is lost to call Close() before you try to Open() it again.
It remembers everything.
Its a singleton pattern (technically a Static class in .net terminology) so there can only be one instance. There are facilities to connect to multiple instances of flight sims using WideClient but that's quite an unusual requirement.
Usually you are just connected to one copy of flight sim at a time so it doesn't really make sense to have multiple connections. You can of course use the grouping to read and write different groups of offsets at different times.
Yes, that would be good. I'll add it to my list of things to do. Thanks for the suggestion.
Paul