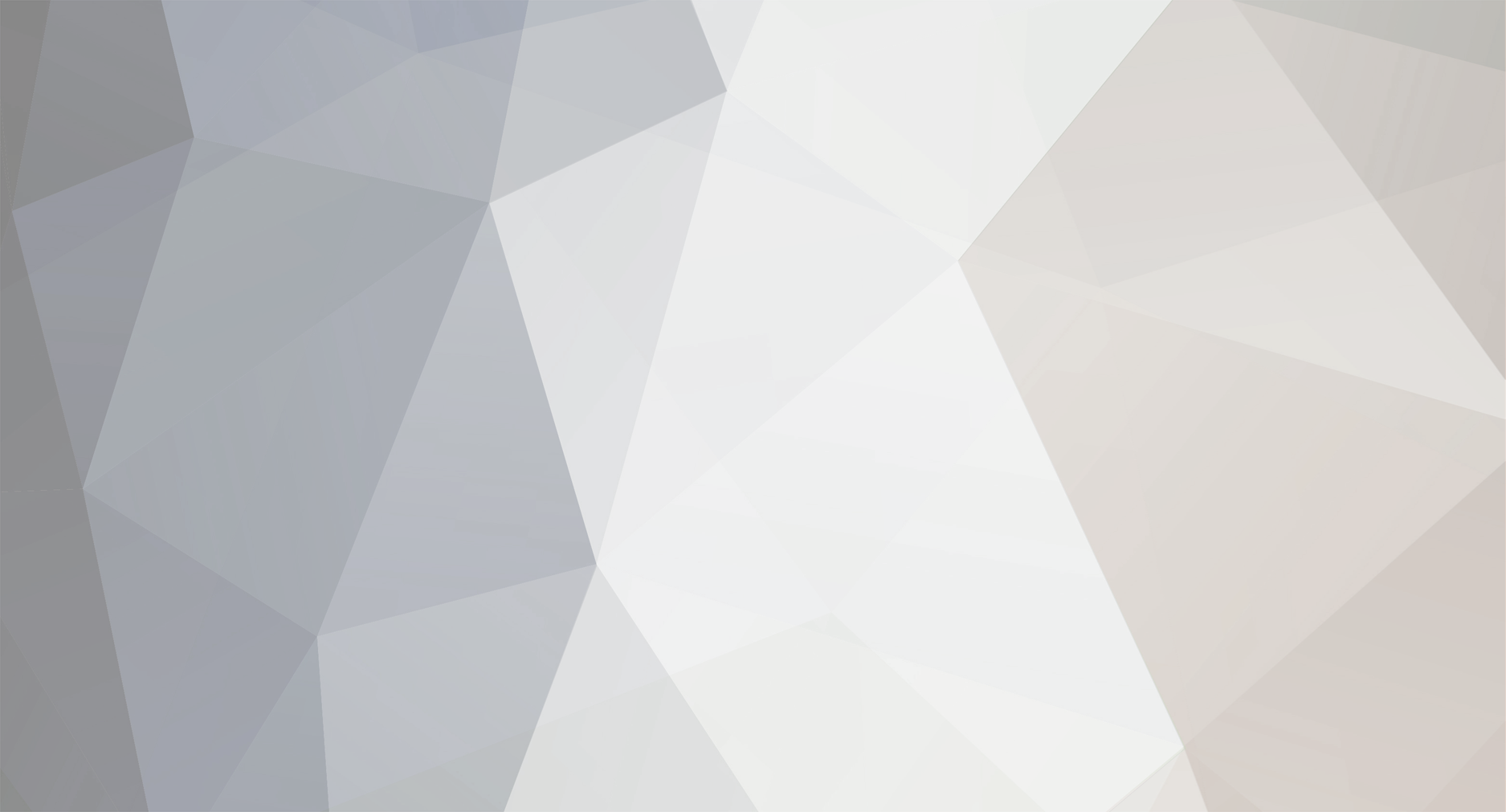
Paul Henty
Members-
Posts
1,724 -
Joined
-
Days Won
77
Content Type
Profiles
Forums
Events
Gallery
Downloads
Everything posted by Paul Henty
-
No that's not quite what I suggested. Firstly, can you not just run your current script on the wideclient machine? That would be the easiest way. If your current script must be run on the FSX machine for some reason then I'm suggesting that you make an additional, independent script on the wideclient machine that just sets the button colours. This script has nothing to do with your current script on the FSX machine, and there is no need for communication between the scripts. The wideclient script simply monitors the relevant offset or lvar using the event library (specifically event.offset() and/or event.Lvar()). When these values change you set the button colour accordingly. For example if you have a button for putting the gear up/down you could monitor offset 0x0BEC (nose landing gear position). When this value changes you can colour the button red when the gear is up, green when it's down and orange when it's in transit. Note that this has nothing to do with your existing script or responding to the button presses. It's simply monitoring the gear position in the simulator and sets the appropriate colour If you're not familiar with the Lua events library, it's explained in the "FSUIPC Lua Library.pdf" in it's own chapter. Search for "The Event Library". I'm not an expert in using Lua with FSUIPC but I from what I know this is a viable solution. Anyway, it's a suggestion for you if you want to try something before Pete gets back and gives you a definitive answer. Paul
-
The official FSUIPC lua documentation states very clearly that the ipc.setbtncol() function is for wideclient only. So this function doesn't exist in the FSUIPC lua library (=nil value), only the wideclient lua library. If your current lua script must be run on the FSX machine then you'll need another script on the wideclient machine that responds to changes in the state of the aircraft systems and colours the buttons accordingly. You could use events for this, monitoring changes in offsets (event.offset()) or changes in LVars (event.Lvar()) depending on how you get the data from your aircraft. Paul
-
>> latest FSUIPC and Make RWY versions installed.. You need to state the exact version numbers rather than just saying 'latest'. Paul
-
You can use the DecimalDegrees property then (as this is a pure number (double)) and use ToString("F6") to turn it into a string with 6 decimal places. Yes, bad technique, If you want to maintain the connection for the lifetime of the application then you should just open it once when the application starts. Usually you would do this on a timer until the open is successful. If the connection ever drops then you restart the timer to attempt reconnection. You have to maintain a connected flag yourself and track the Open() and subsequent Process() failures. Although FSUIPC uses the language of 'connections' being 'open' and 'closed' in reality there is nothing like a connection behind the scenes. The only way you know if the Flight Sim has been unloaded is when a Process() or Open() fails. You need to track those exceptions and maintain your own 'connected' flag. All of this is shown in the sample application that I provide with the DLL. No you don't need any such checks and there is no way anything gets 'balled up'. The only concern would be if you had multiple threads calling GetLatLonString() but there's no way that would happen on such a simple form as you have. Even then, the DLL is thread safe and would just deal with one thread at a time, blocking the others. You can check offset 0x3364. When it's 0 the sim is 'ready to fly'. It's the best available because it's the only official and complete list. It's the only source of information about offsets you should use. You have made a mistake in your new code: Dim ofsLatitude As Offset(Of Long) = New Offset(Of Long)(&H560) Dim ofsLongitude As Offset(Of Long) = New Offset(Of Long)(&H568) These offsets are now declared in your GetLatLongString method. I did not advise this. Offsets should only be declared once in the lifetime of your application.The easiest way to make sure this is the case is to declare them in at the form (or class) level, like you had them before. I've amended your code with the suggestions above. I've also added an example timer mechanism, 'open' connection tracking and the use of the ready to fly offset. You'll need to add a timer to your form called 'tmrConnection' and a label 'lblConnection' to show the status of the connection. Option Explicit On Imports FSUIPC Public Class frmACLocation Dim ofsLatitude As Offset(Of Long) = New Offset(Of Long)(&H560) Dim ofsLongitude As Offset(Of Long) = New Offset(Of Long)(&H568) Dim ofsReadyToFly As Offset(Of Byte) = New Offset(Of Byte)(&H3364) Dim bolConnected As Boolean = False Private Sub btnGetLatLong_Click(sender As Object, e As EventArgs) Handles btnGetLatLong.Click Try Dim sLatLon As String = GetLatLongString() If sLatLon <> "error" Then If MsgBox("a/c Lat/Lon: " & sLatLon & vbCrLf & "Open Chart?", MsgBoxStyle.YesNo) = MsgBoxResult.Yes Then Process.Start("http:\\skyvector.com/?ll=" & sLatLon & "&zoom=3") End If End If Catch ex As Exception MsgBox(ex.Message, , "btnGetLatLong_Click") End Try End Sub Function GetLatLongString() As String Dim mlat As FsLatitude Dim mlon As FsLongitude Dim sLat As String = "" Dim sLon As String = "" Try FSUIPCConnection.Process() If (ofsReadyToFly.Value = 0) Then mlat = New FsLatitude(ofsLatitude.Value) mlon = New FsLongitude(ofsLongitude.Value) sLat = mlat.DecimalDegrees.ToString("F6") sLon = mlon.DecimalDegrees.ToString("F6") Return sLat & "," & sLon Else MsgBox("Flight Sim is not ready to fly", , "GetLatLongString") Return "error" End If Catch ex As Exception 'Badness occurred - show the error message MsgBox(ex.Message, , "GetLatLongString") Me.tmrConnection.Start() 'start the timer again to reconnect Return "error" End Try End Function Private Sub frmACLocation_FormClosing(sender As Object, e As FormClosingEventArgs) Handles Me.FormClosing Try FSUIPCConnection.Close() Catch ex As Exception End Try End Sub Private Sub frmACLocation_Load(sender As Object, e As EventArgs) Handles MyBase.Load configureScreen() Me.tmrConnection.Interval = 1000 ' try connection every second Me.tmrConnection.Start() ' Start looking for flight sim End Sub Private Sub tmrConnection_Tick(sender As Object, e As EventArgs) Handles tmrConnection.Tick Me.bolConnected = False Try FSUIPCConnection.Open() ' Open sucessful Me.bolConnected = True Me.tmrConnection.Stop() ' Stop looking for a connection Catch ex As Exception ' Connection failed FSUIPCConnection.Close() ' Close ready for next retry Finally configureScreen() End Try End Sub Private Sub configureScreen() If Me.bolConnected Then Me.lblConnection.Text = "Connected to " & FSUIPCConnection.FlightSimVersionConnected.ToString() Me.lblConnection.ForeColor = Color.Green Me.btnGetLatLong.Enabled = True Else Me.lblConnection.Text = "Connecting to Flight Sim..." Me.lblConnection.ForeColor = Color.Red Me.btnGetLatLong.Enabled = False End If End Sub End Class Paul
-
Hi, You don't need to worry about garbage collection, the .NET framework will take care of everything for you. Your current 'finally' block is overkill and not required. You could improve things a little by declaring mlat, mlon and mlatlon in the method rather than at the class level as they only seem to be used in that method. They only take a up a few bytes however which is insignificant on modern PCs with gigabytes of memory. The only improvement I would advise is to call FSUIPCConnection.Close() at the end of your Catch block. If you don't do this then you might find you'll never be able to open a connection again if you ever get an exception (e.g. if FSX is not running at the time). To show the lat/lon in decimal format, use the ToString() overload that takes the formatting parameters. See the intellisense for details. (If you're not getting the intellisense make sure you have the FSUIPCClient.xml file in the same folder as your FSUIPCClient.dll.) I suggest the following would be what you want but it's quite flexible so you can tweak it to your exact requirements. mlatlon.ToString(False, "d", 6) Here's your code with the suggested changes: Option Explicit On Imports FSUIPC Public Class frmACLocation Dim ofsLatitude As Offset(Of Long) = New Offset(Of Long)(&H560) Dim ofsLongitude As Offset(Of Long) = New Offset(Of Long)(&H568) Private Sub btnGetLatLong_Click(sender As Object, e As EventArgs) Handles btnGetLatLong.Click Try MsgBox(GetLatLongString) Catch ex As Exception MsgBox(ex.Message, , "btnGetLatLong_Click") End Try End Sub Function GetLatLongString() As String Try FSUIPCConnection.Open() FSUIPCConnection.Process() Dim mlat As FsLatitude = New FsLatitude(ofsLatitude.Value) Dim mlon As FsLongitude = New FsLongitude(ofsLongitude.Value) Dim mlatlon As New FsLatLonPoint = New FsLatLonPoint(mlat, mlon) Return mlatlon.ToString(False, "d", 6) FSUIPCConnection.Close() Catch ex As Exception ' Badness occurred - show the error message MsgBox(ex.Message, , "OpenFSUIPC") FSUIPCConnection.Close() Return "error" End Try End Function End Class Paul
-
Using FSUIPS_SDK_C in Xcode
Paul Henty replied to pcssundahl's topic in FSUIPC Support Pete Dowson Modules
Hi, Unfortunately I know nothing about Python so I can't help you out with that. I suggest making a new topic with Python in the title to attract the right person to your thread. But I have to say I've never seen Python discussed here at all. I've just remembered that FSUIPC has the Lua language built in and that has networking libraries. There is some sample Lua code supplied with FSUIPC that transmits data between two Flight Sim pcs. I don't know if Lua would be suitable for your purposes but it's something to investigate. The Lua documentation and samples are in your Flight Sim 'modules\FSUIPC Documents' directory (folder). Paul. -
Using FSUIPS_SDK_C in Xcode
Paul Henty replied to pcssundahl's topic in FSUIPC Support Pete Dowson Modules
You cannot implement the FSUIPC interface on any operating system but Windows. Even if you got the SDK to compile on your XCode compiler it would be a waste of time as your app needs to run on the same machine as FSX or FS9 or WideClient.exe. That's just how it works. None of these programs run on IOS. If there are iOS apps out there that use FSUIPC then they must be using some bespoke bridging software. This would be installed on the Windows flightsim PC and would get information from FSUIPC and pass it along to the iOS client using their own networking protocols. The .NET Client would help you talk to FSUIPC on the Windows PC, but if you then wanted to send that data to an IOS device you'll need to learn about sending data over a network, not only from the Windows side of things but also the IOS side. You'll also need to learn a completely different language and framework for IOS as .NET doesn't run on IOS. To achieve what you want to do you'll be best sticking with C for both the Windows and XCode side of things. You'll need to know the following: 1. How to get data from FSUIPC on a Windows Machine. 2. How to write a server program on the windows machine to respond to network requests and send data to the client making the request. 3. How to write an IOS app in XCode 4. How to send a network request from the IOS app to the windows server program over the network and receive that data back. The FSUIPC SDK and this forum are for item 1. The other three areas can be found elsewhere on the internet. Paul -
Hi, You seem to be working with the payload stations rather than the fuel tanks. It's not well documented so I've written some examples of working with fuel. I think these will cover your requirements. Example 1: Setting all fuel tanks to 100% full. // Fill all fuel tank PayloadServices ps = FSUIPCConnection.PayloadServices; ps.RefreshData(); foreach(FsFuelTank fuelTank in ps.FuelTanks) { if (fuelTank.CapacityLitres > 0) // If the tank is not in use for this aircraft, capacity = 0 { fuelTank.LevelPercentage = 100; } } ps.WriteChanges(); Example 2: Setting individual tanks from text boxes on a form: // Add fuel levels from textboxes // Example covers only three main tanks // Capacity is tested to make sure the tank is in use for current aircraft PayloadServices ps = FSUIPCConnection.PayloadServices; ps.RefreshData(); if (ps.GetFuelTank(FSFuelTanks.Centre_Main).CapacityLitres > 0) { ps.GetFuelTank(FSFuelTanks.Centre_Main).LevelPercentage = double.Parse(this.txtCentreTankLevel.Text); } if (ps.GetFuelTank(FSFuelTanks.Left_Main).CapacityLitres > 0) { ps.GetFuelTank(FSFuelTanks.Left_Main).LevelPercentage = double.Parse(this.txtLeftTankLevel.Text); } if (ps.GetFuelTank(FSFuelTanks.Right_Main).CapacityLitres > 0) { ps.GetFuelTank(FSFuelTanks.Right_Main).LevelPercentage = double.Parse(this.txtRightTankLevel.Text); } ps.WriteChanges(); Example 3: Showing/Hiding textboxes according to tanks used by current aircraft // Show hide textboxes according to tank usage on current aircraft PayloadServices ps = FSUIPCConnection.PayloadServices; ps.RefreshData(); this.txtCentreTankLevel.Visible = ps.GetFuelTank(FSFuelTanks.Centre_Main).CapacityLitres > 0; this.txtLeftTankLevel.Visible = ps.GetFuelTank(FSFuelTanks.Left_Main).CapacityLitres > 0; this.txtRightTankLevel.Visible = ps.GetFuelTank(FSFuelTanks.Right_Main).CapacityLitres > 0; Paul
-
Well there isn't a single offset that makes the plane refuel is that's what you mean. You can set the level of each fuel tank in the aircraft. These offsets start at 0B7C, but it's much easier to set fuel using the payload services in my DLL. Please see this post for details: http://forum.simflight.com/topic/74848-fsuipc-client-dll-for-net-version-24/?p=457925 Using the payload services makes it easy to set the fuel by % level, weight (lbs or kgs) or volume (litres or gallons). Paul
-
I think you want the 'simulation rate' at 0C1A. Lookup this offset in "FSUIPC4 Offset Status.pdf" as there are some limitations writing to this offset for FSX. Reading is fine though. You need to declare it as 'ushort' and you need to divide the .value by 256 to get the correct value. Paul
-
Are you running a very old version of FSUIPC4? If you're not using 4.929, please update (see the downloads sub-forum) and try again. Paul
-
You have not answered this question yet: What is the line that is throwing this error? Is it the FSUIPCConnection.Open() or one of the Process() calls? Also when you say "In log file nothing!", do you mean you can't see anything interesting or there is no text at all in the log file? I still think you should paste the contents of FSUIPC4.log here. Paul
-
Well if other FSUIPC programs are working then I don't know what else to suggest. The whole point about FSUIPC is that there is no difference between connecting to FSUIPC3 or FSUIPC4. What is the line that is throwing this error? Is it the FSUIPCConnection.Open() or one of the Process() calls? Is there anything shown in the FSUIPC4 log file (in the FSX Modules folder)? Maybe post the contents of the log file here; it might help. Paul
-
Hi, Things you can try: 1. If you are running FSX 'as administrator' you need to run your client program 'as administrator' as well. If you're not running FSX 'as administrator' then make sure you don't run your client program 'as administrator'. 2. If your FSUIPC4 is registered make sure the date on the PC is set correctly. 3. Test your FSUIPC4 installation by running a different FSUIPC program (e.g. FSInterrogate that comes with the FSUIPC SDK, or TrafficLook available in the downloads sub-forum). If other FSUIPC programs do not connect then something is wrong with your FSUIPC4 installation. Paul
-
The way this would be done would be to have the web application server (e.g. php, python, asp.net or whatever) call into a DLL that will talk to FSUIPC. This could either be a COM DLL or a .NET DLL depending on which is supported by the application server you are using. I don't have any experience with Apache or php or python, so if you wanted to use these with Apache you'd need to know how to use them yourself. These could call into my FSUIPC Client DLL for .NET and I could help you with that side of things, or even create a COM wrapper if the .NET route didn't work. I do have experience with Microsoft's web technology (IIS and ASP.NET) so I could give you much more help with those. You can get them with the free version of "Visual Studio Express 2013 for Web". This would be more suited to using my .NET DLL and you could write your code in C#. Either way it's a big learning curve for you if you're not already familiar with these technologies. You would need to learn the basics first before attempting to interface with FSUIPC. You'll likely find lots of good tutorials on YouTube. That's all I can suggest. Someone else may have some other ideas. Paul
-
Can you give some more details about what you're proposing: What kind of webserver technology are you using? Is the server always going to be localhost or might the server be on the internet? Is this meant to control a copy of FS on the webserver or on the same machine as the client browser? Without knowing the above it's difficult to give any clear advice about this. Paul
-
How to add option to FS9 menu bar
Paul Henty replied to Mohamed Sayed's topic in FSUIPC Client DLL for .NET
Hi Mohamed, This question is probably best asked in the main support forum above this one. This sub-forum is just for my .NET DLL and your question will only be seen by a few people here. I'm fairly sure that making an FS module DLL isn't possible in VB6. If your VB program is a standalone .exe, you can use facilities in FSUIPC to add your own menu item and respond to them. See the document called "FSUIPC for Programmers.pdf" in the FSUIPC SDK. The relevant section is called "MODULES MENU access for Applications". Paul -
Problems with aircraft type (C#)
Paul Henty replied to Even92LN's topic in FSUIPC Support Pete Dowson Modules
There's your problem. That isn't what TrimEnd() does. It only removes the specified character if it's the last character(s) of the string. To remove everything after the \0 you need a bit more code... string tmp = System.Text.Encoding.ASCII.GetString(type); int pos = tmp.IndexOf('\0'); if (pos >= 0) { tmp = tmp.Remove(pos); } Paul -
George, I posted the exact code you need to get this working. I showed you the offsets you need. I showed you how to convert the offset values into the correct units. I told you that you need to subtract the ground height from the altitude. I even wrote the code for the subtraction. I'm sorry that you find all of this inconvenient. I'm not sure what else you expect me to do. I don't have the skill to guess what variable names and label names you would prefer. I'm just not that good a programmer. Maybe you are trolling me, in which case you've had lots of fun wasting my time. If you are sincere there you really need to learn to program before asking me for any more help. If you cannot take my working examples and put them into your code then I am wasting my time. There are many excellent C# courses available on YouTube for free. I cannot lock this thread but I will not be answering any more of your questions. [LOCKED IT FOR YOU! Pete] Paul
-
Hi George, Offset 0020 is the height of the ground (above sea level) directly under the aircraft. So to get the altitude of the aircraft above the ground you subtract this from the altitude of the aircraft: private Offset<long> altitude = new Offset<long>(0x0570); private Offset<int> groundHeight = new Offset<int>(0x0020); //Altitude in meters double altitude_Metres = (double)altitude.Value / (65536d * 65536d); //---------------------------------------------------------------------------------// //Ground height in meters double groundHeight_Metres = (double)groundHeight.Value / (256d); //---------------------------------------------------------------------------------// //Altitude above ground in meters double altitudeAboveGround_Metres = altitude_Metres - groundHeight_Metres; //---------------------------------------------------------------------------------// //Altitude above ground in feet double altitudeAboveGround_Feet = altitudeAboveGround_Metres * 3.28084d; this.lblAltitudeAboveGround.Text = altitudeAboveGround_Feet.ToString("F0"); Paul.
-
There is no such offset. You would need to keep track of this yourself. Every few seconds you should get the current position (lat/lon) and measure the distance from the previous position. Then add this to a total. I suggest adding another timer to the form and doing this every 10 seconds. The more often you track the position the more accurate the distance will be. You can use the FsLonLatPoint class to measure the distance between two points in nm or km. E.g. distanceFlown += currentPoint.DistanceFromInNauticalMiles(previousPoint) Paul
-
Getting assigned parking for AI when taxi in
Paul Henty replied to Garfield_X's topic in FSUIPC Client DLL for .NET
Sorry about that. There was a bug with the write only offsets. Fix attached. Paul FSUIPCClient2.6_NET2.zip FSUIPCClient2.6_NET4.zip