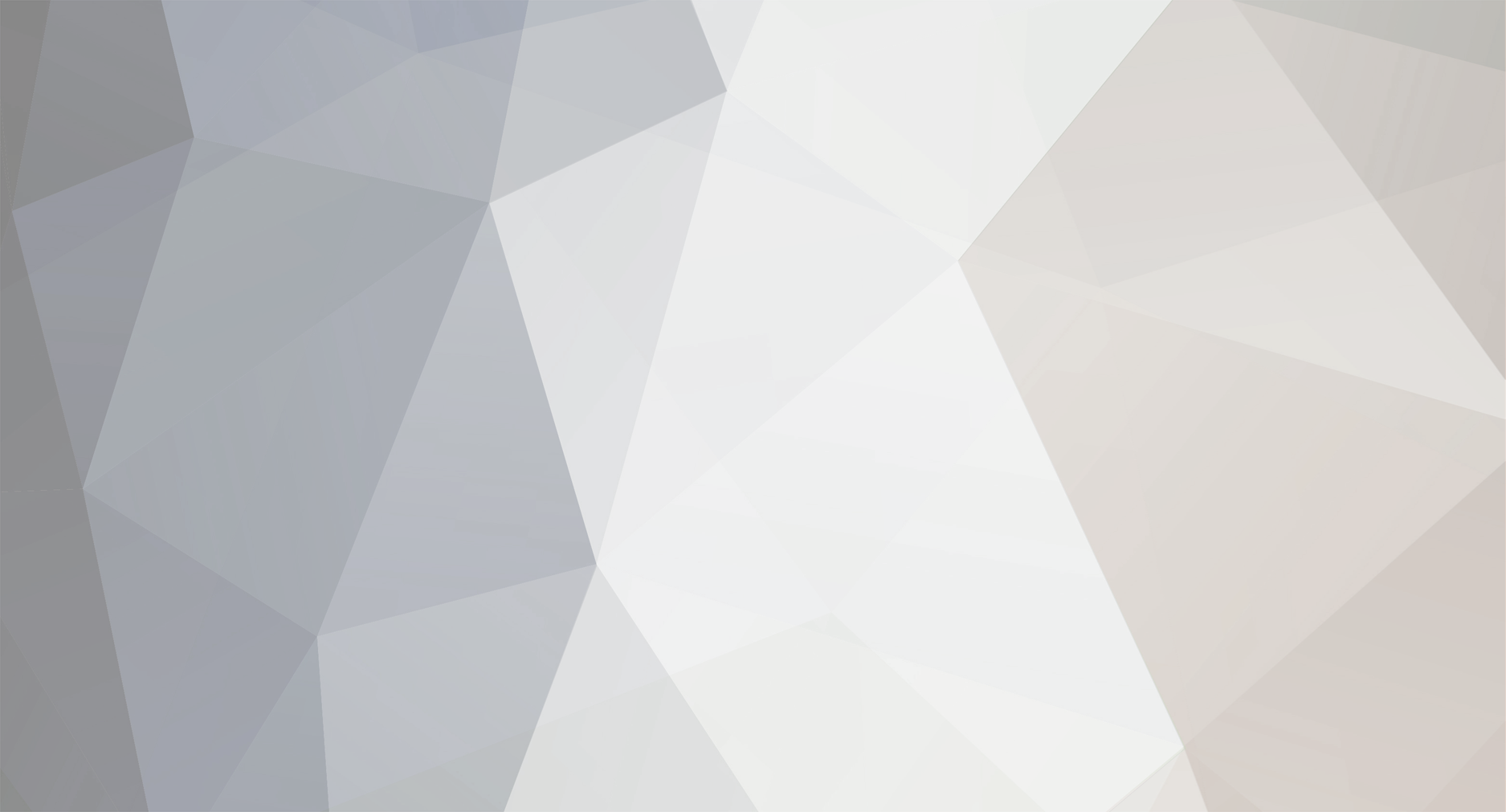
Paul Henty
Members-
Posts
1,652 -
Joined
-
Days Won
74
Content Type
Profiles
Forums
Events
Gallery
Downloads
Everything posted by Paul Henty
-
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
The code looks good to me. The only mistake I can see is that turbRpm (0x0896) is only 2 bytes and should therefore be declared as 'short' not 'int'. private Offset<short> turbRpm = new Offset<short>(0x0896); I'm not sure if you had a problem you want help with. If you do, please be more specific about what the problem is. Regards, Paul -
Global weather in FSX Dotnet client 2.0
Paul Henty replied to André Gonçalves's topic in FSUIPC Support Pete Dowson Modules
It's not easy in C# because my DLL doesn't support reading and writing structures. I've also never tried using the NWI myself, but this might work... You need to convert your structure to a byte array before assigning it to the offset. Here is a method that will do that: You'll need to add the following using statement: using System.Runtime.InteropServices private byte [] StructureToByteArray(object obj) { int len = Marshal.SizeOf(obj); byte [] arr = new byte[len]; IntPtr ptr = Marshal.AllocHGlobal(len); Marshal.StructureToPtr(obj, ptr, true); Marshal.Copy(ptr, arr, 0, len); Marshal.FreeHGlobal(ptr); return arr; } Now you can use this to give the offset the byte array, rather than the structure itself: NewWeather weather = new NewWeather(); weather.uCommand = 3; NWICommand.Value = StructureToByteArray(weather); // pressure.Value = 1024; FSUIPCConnection.Process(); I don't know if this will work but it's certainly a step in the right direction. If you want to read from the NWI, you'll need to convert the byte array back into a structure using this method: private void ByteArrayToStructure(byte [] bytearray, ref object obj) { int len = Marshal.SizeOf(obj); IntPtr i = Marshal.AllocHGlobal(len); Marshal.Copy(bytearray,0, i,len); obj = Marshal.PtrToStructure(i, obj.GetType()); Marshal.FreeHGlobal(i); } Paul -
[Help] FSUIPC Connection
Paul Henty replied to Kaan Kuscu's topic in FSUIPC Support Pete Dowson Modules
Do you mean Visual Basic by Microsoft? If so, it's fairly simple. Let me know which version (VB6? VB.NET 2012?) and I'll tell you what you need and where to find it. If you mean a different language called Vbasic, there is no FSUIPC SDK for it. You'd have to write one yourself by translating the C SDK, Paul -
Yes, that's all correct. A list of all the offsets (code numbers) can be found in the FSUIPC SDK. For FSUIPC4 the document is called 'FSUIPC4 Offsets Status.pdf'. This document tells you three main things about each offset: 1. The offset code (address) e.g. 02BC 2. The Size of the offfset in bytes and the type of data stored in the offset - This tells you what data type to Dim your offset as. Example: 4 byte integer = (As Integer), 2 Byte = (As Short). 3. How the value is stored so you can convert it to real-world values. Example: (Indicated Air Speed, as knots * 128). This means to get back to knots you need to divide by 128. - (airSpeed.Value / 128D) There is a useful table on page 7 of my UserGuide.pdf (see the Docs folder in the Zip) which tells you what .NET types to use for each size/type of FSUIPC offset. Paul
-
HI Joaogl, I've reproduced the error you are getting on the form designer. You can fix it by building or running the project, then close the form designer and reopen it. This isn't really to do with FSUIPC or my DLL. It's just VB / .NET programming. It's not really possible to give VB lessons in this forum. You can find this kind of thing elsewhere on the internet or in books. But to answer your question just convert to a string using a fixed point format with 0 decimal places: Dim mySpeed as Double = 50.5 myLabel.Text = mySpeed.ToString("F0") [/CODE] This will probably round the number for you. If you don't want rounding then use the Floor function. [CODE] Dim mySpeed as Double = 50.5 myLabel.Text = Math.Floor(mySpeed).ToString() [/CODE] Paul
-
Hi, Have you tried to build the project or have you just gone into the form designer. Building it first might help. If it won't build, let me know if you can see the DoubleBufferPanel class. If should be at the bottom of the Form1.vb code file. It looks like this: Public Class DoubleBufferPanel Inherits Panel Public Sub New() ' Set the value of the double-buffering style bits to true. Me.SetStyle(ControlStyles.DoubleBuffer Or ControlStyles.UserPaint Or ControlStyles.AllPaintingInWmPaint, True) Me.UpdateStyles() End Sub End Class [/CODE] Paul
-
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
I'm pretty sure there is no way to get flight numbers for sleeping planes. They just don't have flight numbers assigned to them by Flight Sim. Certainly FSUIPC is not providing the information so I can only assume it doesn't exist. Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
Hi Achilles, That's probably because the ground planes are 'sleeping'. They only have flight numbers assigned when they are in an active state like 'Taxiing out' or 'ready for takeoff'. I've tried it here with FSUIPC4 and the active ground planes do have flight numbers. The sleeping ones (most of them) do not. Incidentally, it's probably better to set the INI options before refreshing the AI data: FSUIPCConnection.AITrafficServices.OverrideGroundTrafficINISettings(ATCIdentifier.AirlineAndFlightNumber,True, 0, 0) FSUIPCConnection.AITrafficServices.OverrideAirborneTrafficINISettings(ATCIdentifier.AirlineAndFlightNumber, RangeInNM:=0) FSUIPCConnection.AITrafficServices.RefreshAITrafficInformation() [/CODE] If you still think there's a problem (e.g. you can't see flight numbers on active ground AI) let me know and I'll look into it some more. Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
Hi, There's nothing wrong with the code that I can see. I've tried it here using "02BC" (Airspeed) in the textbox and it worked fine. It would depend on what you're typing into the textbox and what kind of data is stored at that offset. You can obviously only use offsets of 4 bytes that are integers with the code you posted. This is not related to your problem, but you need to be careful when creating offsets when the program is running. When you 'Dim' a new offset it's registered with the FSUIPCConnection class which holds a reference to that Offset instance it. If that offset then goes out of scope in your program it will still be registered with the FSUIPCConnection class. Therefore it is still taking up memory and will still be read every time you do Process(). As your program is at the moment, if you use this dynamic feature 50 times you will have all 50 offsets still in memory and all 50 being read each Process(). To clean up offsets you don't want any more you must either: 1. Disconnect them by calling MyOffset.Disconnect() or 2. Delete the group they are in (If you put them in a group). e.g. FSUIPCConnection.DeleteGroup("MyTemporaryOffsets") Here is your code again (works here for a few offsets I tried - e.g. 02BC (Airspeed)) but with the disconnect in: Dim Newoffset = "&H" & TextBox1.Text Dim readoffset As Offset(Of Integer) = New FSUIPC.Offset(Of Integer)(Newoffset) FSUIPCConnection.Process() MsgBox(readoffset.Value) readoffset.Disconnect() [/CODE] If you still have problems let me know what offsets don't work. Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
I don't know of any, sorry. Certainly my DLL isn't supplying such information and I don't remember reading anything about that in the FSUIPC documentation. Maybe Pete or someone else might be able to help you if you post in the main support forum. Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
Hi Achilles, All the locations used in my DLL are an instance of the FsLatLonPoint class. This has properties for the Longitude and the Latitude which are instances of FsLongitude and FsLatitude. These classes have various properties for obtaining the lon/lat in various ways. It's all explained on the Intellisense and in the user guide. If you're not seeing the intellisense then make sure you have the FSUIPCClient.XML file in the folder as the FSUIPCClient.DLL. To answer your specific question about the lon/lat of AI traffic you can use the following to get the lon and lat as a numeric value of degrees: Dim aiPlaneLat As Double = myAIPlane.Location.Latitude.DecimalDegrees Dim aiPlaneLon As Double = myAIPlane.Location.Longitude.DecimalDegrees [/CODE] Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
You need to use offsets 3380 and 32FA. Details of these can be found in the FSUIPC documentation. Here is some example code: Declaration of the required offsets: Public messageText As Offset(Of String) = New Offset(Of String)("textstrip", &H3380, 128, True) Public messageControl As Offset(Of Short) = New Offset(Of Short)("textstrip", &H32FA, True) [/CODE] Note that 3380 requires the offset length (128) as it's a string type. Also they are both declared as WriteOnly because there is no point reading what's in these offsets. I have also put them in thier own offset group called "textstrip". This is so we can send messages without processing all the other offsets. The code to write the message: [CODE] messageText.Value = "XXX has connected with your FSX" messageControl.Value = 5 FSUIPCConnection.Process("textstrip") [/CODE] Note that here we only process the "textstrip" group. This will make the message appear on the screen for 5 seconds. See the FSUIPC documentation for the different options avilable for the control offset (32FA). Paul -
FSUIPC.net dll use with Module
Paul Henty replied to Alex White's topic in FSUIPC Support Pete Dowson Modules
The hex specifier in VB is &H. So you need this: Public Gear As Offset(Of Integer) = New FSUIPC.Offset(Of Integer)(&HBF0) ' gear Please take some time to review the UserGuide that comes with my DLL and the VB sample application. Everything is explained and shown in great detail. Paul -
FSUIPC.net dll use with Module
Paul Henty replied to Alex White's topic in FSUIPC Support Pete Dowson Modules
Of course. The positions of the landing gear are at offsets 0BEC, 0BF0 and 0BF4. Information about these offsets and all other offsets are found in the documentation from the FSUIPC SDK. Either "FSUIPC4 Offsets Status.pdf" for FSX or "FSUIPC for Programmers.pdf" for FS9 and earlier. Paul -
FSUIPC.net dll use with Module
Paul Henty replied to Alex White's topic in FSUIPC Support Pete Dowson Modules
Anyway I think I see the problem: FSUIPCConnection.Process("") If you don't want to process a particular group just use: FSUIPCConnection.Process() The error you are getting probably says that the group "" does not exist. If that's not it then show me the message from the exception that's being thrown. Paul -
Trouble with 0x88C offset in C#
Paul Henty replied to rafaelcoronel's topic in FSUIPC Support Pete Dowson Modules
The most likely cause of your problem is that throttle_1 is declared and 'int' (4 bytes) and not 'short' 2 bytes. Therefore, at the same time you're writing to 0x088C you are also trampling over 0x88E which is the 'prop lever'. Could this possibly be the collective in helicopters? Changing the collective on a helicopter would certainly account for a change in N1. Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
Hi Ron, My snippit of code assumes there is a . in the frequency string because that's how it normally is. However, if your string doesn't have a . in it then you can just change it to fit what you have. FSUIPC requires 121.55 to be in the format 2155. In my example the second line of code transforms 121.55 into 2155 buy concatenating the second, third, fith and sixth together: Dim com2FS As String = newCom2Frequency.Substring(1, 2) & newCom2Frequency.Substring(4, 2) So if you are starting with 12155 all you need to do is take the second digit onwards: Dim com2FS As String = newCom2Frequency.Substring(1) So the whole example would be: Dim newCom2Frequency As String = "11945" ' 119.45 as read from arduino ' get the freqeuncy into FS format (no leading 1 = 1945) Dim com2FS As String = newCom2Frequency.Substring(1) ' set the value to the com2 offset after converting to a short as a hex string com2Offset.Value = Short.Parse(com2FS, Globalization.NumberStyles.AllowHexSpecifier) If you use the code above you don't need to. But incase you need it for display purposes just use the Substring() method: Dim stringFromArduino as String = "12155" Dim stringWithDecimal as String = stringFromArduino.Substring(0,3) & "." & stringFromArduino.Substring(3) Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
Hi Ron, You need to take the BCD string and convert it to a Short as if it was a hex string. Here is a snippit of code that shows how: I think the last line is what you're looking for... Dim newCom2Frequency As String = "119.45" ' Set to 119.45 ' get the freqeuncy into FS format (no . and no leading 1 = 1945) Dim com2FS As String = newCom2Frequency.Substring(1, 2) & newCom2Frequency.Substring(4, 2) ' set the value to the com2 offset after converting to a short as a hex string com2Offset.Value = Short.Parse(com2FS, Globalization.NumberStyles.AllowHexSpecifier) Paul -
If you are using my DLL then the easiest way is to declare the offset as a 'BitArray' type. The sample application included with the DLL has an example of using a BitArray offset for the lights. Basically, you need to declare the offset as follows: Dim enginesOnFire As Offset(Of BitArray) = New FSUIPC.Offset(Of BitArray)(&H3366, 1) [/CODE] Note the size is 1 because the offset is 1 byte. Then after processing you can check if each bit is set by using an array index. This is 0 based so to test is bit 0 is set you use: [CODE] If enginesOnFire.Value(0) = True then ' Engine 1 on Fire! end if [/CODE] Engine 2 would be enginesOnFire.Value(1) Engine 3 would be enginesOnFire.Value(2) Engine 4 would be enginesOnFire.Value(3) You can also set and reset these bits by setting the value to True or False repectively e.g. [CODE] enginesOnFire.Value(2) = True [/CODE] although the documentation says this probably doesn't start a fire in the simulation, just sets the warning light. Paul
-
Has offset 0x3308 (FS version) been updated?
Paul Henty replied to lordofwings's topic in FSUIPC Support Pete Dowson Modules
My DLL will also tell you with the FlightSimVersionConnected property: if (FSUIPCConnection.FlightSimVersionConnected == FlightSim.FSX) { // Do stuff } [/CODE] Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
Hi, You use the full size. The dll knows about 0 terminations etc. -
Using C# SDK and Offset 3380
Paul Henty replied to Raysot's topic in FSUIPC Support Pete Dowson Modules
The DLL supports reading and writing of strings directly so just declare 3380 as a string type: public Offset<string> messageWrite = new Offset<string>(0x3380, 128); [/CODE] The control offset at 0x32FA is defined in the documentation as 2 bytes. You should therefore decalre this as a short, not an int (which is 4 bytes). [code] public Offset<short> messageDuration = new Offset<short>(0x32FA); The full code will something like this: public Offset<string> messageWrite = new Offset<string>(0x3380, 128); public Offset<short> messageDuration = new Offset<short>(0x32FA); string Message = "my message test"; this.messageWrite.Value = Message; this.messageDuration.Value = 2; FSUIPCConnection.Process(); Paul -
FSUIPC Client DLL for .NET - Version 2.0
Paul Henty replied to Paul Henty's topic in FSUIPC Client DLL for .NET
There are examples in the UserGuide.pdf that comes with the DLL. There is also an example in the sample application. Both are in C# and VB. Have you seen these? Paul