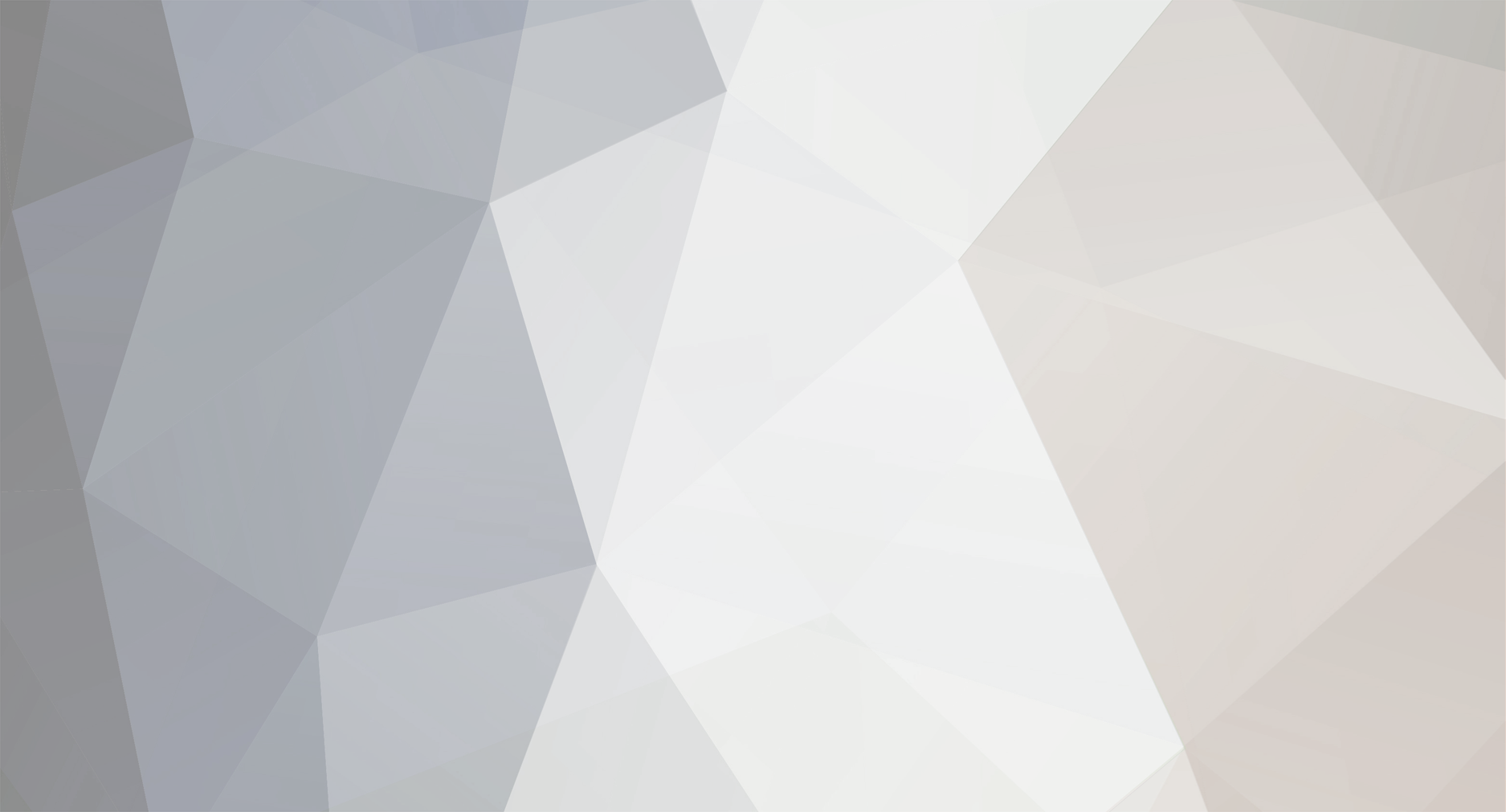
Paul Henty
Members-
Posts
1,728 -
Joined
-
Days Won
78
Content Type
Profiles
Forums
Events
Gallery
Downloads
Everything posted by Paul Henty
-
Hi Lukas, It's not enough to run the .exe located in the FSX folder, you also need to set the 'start' folder for your new process to the FSX folder. How you do that will be specific to your programming language and method of running an external program. There will be some parameter or property that you can set. If you can't find out how to do this I would suggest mentioning the programming language you are using or posting the code that doesn't work. Someone who knows that language will then be able to help further. Pete will pick up your other question on his return. Paul
-
Hi Manfred, The METAR strings are returned directly from the SimConnect interface (via FSUIPC). These contain extended data used by SimConnect in addition to the standard METAR codes. The extensions tend to start with &. &D920NG means the (D)epth (height) of the wind layer is 920 metres, the turbulence is (N)one, and wind shear is (G)radual. The &A is for winds above the surface layer and specifies the altitude in metres (in your example 1539m), The official specification of the SimConnect METAR string can be found here: http://msdn.microsoft.com/en-gb/library/cc526983.aspx#Metar_Data_Format If you want a clean, standard METAR string then you can either build one from the SimConnect METAR, or create your own from the information in the FsWeather object e.g.: FsWeather weather = ws.GetWeatherAtLocation(IACO); Paul
-
Converting nm to FS Format
Paul Henty replied to xxallrounderxx's topic in FSUIPC Support Pete Dowson Modules
The runway heading you used (161) seems to be the Magnetic heading. You need to use True headings when working with Lon/Lat positions. The True heading of this runway is about 164. If you don't have the true headings for the runways you can derive them from the magnetic headings by adding the magnetic variation. This can be found is offset 02A0, but only gives the value for the current position of the player. Paul -
Converting nm to FS Format
Paul Henty replied to xxallrounderxx's topic in FSUIPC Support Pete Dowson Modules
Pete's away at the moment so I'll tell you how I did this in my .NET Client DLL for FSUIPC. It has a helper class for calculating the very thing you describe. I assume you're not using .NET and so can't use these facilities, so here's what's 'under the hood': What I suggest is not converting the NM distances into FS Units, but rather use them to calculate new Lon/Lat positions, then convert these to FS Units in the normal way. Converting Lon/Lat to FS Units for 0560 and 0568 is well documented and fairly easy. Trying to convert NM distances into these units is not documented and more difficult. Adding/subtracting NM distances to Lon/Lat coordinates is easy for Latitude and a bit more cumbersome for Longitude. The following calculations all assume the Lon/Lat is in pure degrees and fractions of a degree (e.g. you're working with a value of 50.5 degrees representing 50 Degrees 30 Minutes) Latitude: 1 Nautical Mile = 1/60th of 1 degree of Latitude, or 1 Degree of Latitude = 60 NM. Therefore the number of degrees (or fraction of degrees) to add for distance d in NM = d / 60. e.g. Adding 30NM to latitude 50.454: 50.454 + (30 / 60) = 50.954 Longitude: A bit more tricky because the length of 1 degree of longitude varies depending on the latitude because the circles of longitude are smaller towards the pole than at the equator. The first step is to figure out the length of a degree of longitude at the latitude of the point. This is given by: cosine(latitude) * circumference of FS equator / 360 The circumference of the FS Equator is 40,075km = 21,638.7689 NM So at 49.882 degrees latitude, one degree of longitude (in FS at least) is: cos(49.882) * 21638.7689 / 360 = 38.73 NM (Assume the cos function above takes degrees to keep this simple. Most programming languages have trig functions that take radians). The next step is to calculate the number of degrees of longitude to add for the given distance (same as latitude but using the value from step 1 instead of the fixed 60 value) e.g. Adding 30mn to 2.33 degrees longitude at latitude 49.882: 2.33 + (30 / 38.73) = 3.10 Once you have the new Lon/Lat you can just do the normal conversion to FS units to write to the offsets you mentioned. Paul Nothing in this post should be used for real-world navigation :smile: -
Everything is in the FsWeather structure. The intellisense will tell you everything you need to know after you press the '.' key. To get you started, here is a sample of stepping though each wind layer and getting the speed and direction: FsWeather weather = ws.GetWeatherAtLocation("EGLL"); foreach (FsWindLayer wl in weather.WindLayers) { // get information from the wind layer e.g. Double direction = wl.Direction; Double speed = wl.SpeedKnots; } Paul
-
Yes, see offsets 3380 and 32FA. Make sure offset 3380 is declared before 32FA. FSUIPC get this from SimConnect which uses a special format for FSX. If you want a more standard one you have two choices: 1. Create a new one by parsing the one above and pulling out the information you want. 2. Get back a full weather structure with: FsWeather weather = ws.GetWeatherAtLocation("EGLL"); You'll then need to construct your METAR string by reading all the information in the weather structure. Paul
-
Hi, By far the easiest way is to use Version 3 of my DLL. I haven't released it because I haven't written the documentation yet, and I've had a few users testing it. I've attached the latest to this post. It seems to be pretty stable now. Just overwrite your current DLL and XML files with the ones included in the zip. There are different version depending on what version of the .NET framework you are targeting (2 or 4). To get the METAR for an ICAO use the following code: // 1. Get a reference to the weather services to save typing... // NOTE: Connection must already be open. WeatherServices ws = FSUIPCConnection.WeatherServices; // 2. Get METAR string at London, Heathrow string METAR = ws.GetMetarAtLocation("EGLL"); You don't need to call Process() when using the weather facilities, you get the results immediately. The DLL has full Intellisense documentation, but if you need any more help let me know... Paul FSUIPCClient3.0_BETA.zip
-
Embed FSUIPCClient.dll in .EXE
Paul Henty replied to vgmarimon's topic in FSUIPC Client DLL for .NET
Not with Visual Studio. You can download a tool called ILMerge that will merge the .exe and the dlls into a single .exe after they are compiled by VS. It's a command line utility but it's quite simple to use. You can get it here: http://www.microsoft.com/en-us/download/details.aspx?id=17630 Here is an example of the command to merge my example project .exe with the FSUIPCClient.dll. This is run from the folder with the two files in, e.g. the bin/release folder, so 'cd' there first. "C:\program files (x86)\microsoft\ilmerge\ilmerge.exe" FSUIPCCLientExample_CSharp.exe FSUIPCClient.dll /out:ExampleMerged.exe /target:winexe /targetplatform:v4 /log:mergelog.log /ndebug After the ilmerge.exe command, list the name of the main .exe followed by all the dlls you want to merge (I just have one in this example). Set the /out: parameter to the name you want your single .exe to be. Then add the other options, but make sure you set the target platform correctly, i.e. if you're targeting version 2 of the .NET runtime then you would need /targetplatform:v2. In my example I was targeting version 4.0. Paul -
In the zip file that you downloaded there is a docs folder. In there is a file called userguide.pdf. On page 7 there is a table explaining how to choose the variable type from the information given in Pete's document "FSUIPC4 Offset Status.pdf". Paul
-
Hi Ido, Declare the offset like this: private Offset<string> nearestICAO = new Offset<string>(0x0658, 4); Then in your code, after the process(), read the value like this: FSUIPCConnection.Process(); MessageBox.Show("Nearest airport is " + this.nearestICAO.Value); Paul
-
Yes, the simplest way is to use each byte (each offset number in the block) to store a 1 or 0 value and read these from .net. You could make it more memory efficient by using individual bits, so each byte will hold the state of 8 lights. This is a little more complicated as you'll need to deal with the lua to set and reset each bit individually i.e. ipc.clearbitsUB(offset, mask) and ipc.setbitsUB(offset, mask). If you use my DLL you can declare the offset using the BitArray type to make this easier on the .net side. Paul
-
You have all of that area of memory to use (64 bytes). The offset addresses you use within this block will be determined by what kind of data you are writing there, specifically the length in bytes of each value. If you wanted to write 64 values and each was 1 byte long then you would have all offsets (hex numbers) available. However, let's say you're writing an integer value that is 2 bytes long (e.g. 'short' in most .net languages). The first value would be written to 0x66C0, but it would take up 2 bytes so 0x66C1 would be taken by the second byte. The next available offset would be 0x66C2. (If you wrote anything to 0x66C1 you'd overwrite part of the first value). What you have to do is map out your own offsets within the block based on the length (in bytes) of each value you are writing. Paul
-
Download 2.4 from the sticky at the top of this sub-forum. Paul
-
Help with Offset 0x30C0 in FSX
Paul Henty replied to Michael Kostelcev's topic in FSUIPC Support Pete Dowson Modules
It's almost impossible to see what's wrong without seeing your code. Specifically we need to see where you are reading the offset (or the Offset<> declaration if you are using my DLL). According to the documentation 0x30C0 is 8 bytes and is in double floating point format. You must make sure you read 8 bytes and that it's being converted to a 'double' type in c#. If you are using my DLL you just declare the offset type as double. From the results you've posted it seems like you are only reading 4 bytes and treating the returned value as an integer. Paul -
Hi Paul, None of the .csv files contain info about the airport beyond the ICAO code. The Runways.txt file contain lots of information about the airports (including runway and comms info) but it's not in a very friendly format for parsing by code. It's possible, but I think it's more intended for humans to read. Your only other option (and the one I used) is runways.xml which has some good information about the airport (lon/lat, name, city etc). It also has runway info. You'll need to write some code to read the XML file and then push this data into your SQL database. Depending on what programming language you are using there could be some built-in or free libraries to parse XML files into an easily navigable class structure. Failing that you'll need to write your own text parser based on the rules of XML. Dealing with the XML will be much easier than trying to work out the format of a third-party's binary file, especially if you have access to ready-made libraries that handle XML reading. Paul
-
0x311A COM1 standby frequency 0x311C COM2 standby frequency 0x311E NAV1 standby frequency 0x3120 NAV2 standby frequency They are in the offsets status document. Paul
-
Hi Joe, The code you posted is just reading offset B800, but it's more complicated than that. There is a weather reading process you need to go through first to tell FSUIPC what station or lon/lat you want the METAR for. This is all described in the "NewWeather ReadMe.txt" document supplied with the FSUIPC SDK. Specifically the section called "CC00-CFFF (Area 3): Weather reading area". This should be read in conjunction with "NewWeather.h" which is a C header file that contains the data structures. I'm not sure how you're going to deal with writing C data structures in VB6 though. You may need to build byte arrays instead but I don't know VB6 that well. Paul
-
Hi JayCee, I think the simplest explanation for this is that the FSHostClient program isn't transmitting the local com frequency to the server. It's probably not something they thought of doing. If that's the case then there's no way FSUIPC (and therefore my DLL) could pick it up. I suggest getting confirmation from Russell about this first. Paul
- 4 replies
-
- Multiplayer
- Frequency
-
(and 1 more)
Tagged with:
-
Incorrect data given by FSUIPC
Paul Henty replied to gdscei's topic in FSUIPC Support Pete Dowson Modules
Just a small suggestion; you might want to look at using the "event.Lvar" function rather than the infinite while loop. I believe it's far better from a performance point to view. Paul -
Incorrect data given by FSUIPC
Paul Henty replied to gdscei's topic in FSUIPC Support Pete Dowson Modules
You are right, there is no offset or any feature in FSUIPC to transfer data to or from lua. There are spare offsets you can use in FSUIPC, I think called user offsets or free offsets in the docs. Sorry for being vague but I am away at the moment with no access to the docs. You can have your lua program copy the LVAR data into these offsets and read them from your program in the normal way. If your program is being released to the public then it is advisable to ask Pete for some allocated offsets for this purpose instead of using the free block. Another way would be writing the data to a local file in lua and then reading it from your program. Or you can open a local tcpip socket connection using the libraries in lua and .net and have the two program's communicating over that connection. I think those are your options. Paul -
Confused about the innerworking of the DLL
Paul Henty replied to FsWebIO's topic in FSUIPC Client DLL for .NET
Hi, I think the problem is being caused because you are creating multiple offset instances for the same address. For example if you want to access 4 bits from the same offset you end up with four entries in your FsVarList collection, each having the same offset address but different bit numbers. For each one of these you create a new Offset but they all have the same address. The problem is that when writing to FSUIPC, all bits of the offset are written at the same time. So say you want to write 1 in bits 0 and 1: Your first instruction to FSUIPC would be to write the value 1 to offset x. Then you use the second copy of the offset to write the value 2. This second copy does not know about the first one so it cannot send the correct value of 3. When you call process after each set, all the offsets that were not written get updated from FSUIPC. So after you write 1 that second copy of the offset gets updated to 1. Then you set bit 2 on this offset so the new value is 3 which is correct. To fix this you should only have one Offset instantiated for each address. Then you will be able to call process() after you set everything which is more efficient. I suggest keeping a dictionary of offsets keyed on the address. Before creating a new offset check the dictionary to see if there is already one there for that address and use that if there is. If not create one and store it in the dictionary for other FsVar objects to use. You want each FsVar object that uses the same address to be using the same instance of the FSUIPC Offset. If you need any more help with this let me know. Paul -
You make a mistake when copying the code. For setting the bit on you need to OR the bit value not AND it: FS_lights.Value = FS_lights.Value Or (2 ^ 3) ' Expected: only taxi light (3) should change (light ON) Paul
-
Hi Dan, FSUIPC has no facilities to write to a bit directly. The BitArray is used by the dll to make things easier. Normally you'd need to treat 0D0C offset as a short and perform bitwise operations to set the correct bit. e.g. to set bit x you would need to do this: (This is 0 based, so the value of x for the least significant bit is 0 (as specified in the FSUIPC docs)) FS_lights.Value = FS_lights.Value Or (2 ^ x) To reset the bit you do this: FS_lights.Value = FS_lights.Value And (Not 2 ^ x) To get a bit value you do this: Dim bitValue As Boolean bitValue = (FS_lights.Value And (2 ^ x)) > 0 This might help you because I presume you have some way of handling the different sizes of integer at the moment (1,2 and 4 bytes). So you can use the same method to declare the offsets (byte, short, integer), you just can't use the nice bitarray syntax, you must use the above maths. Alternatively you could just ask the user to give you the length of the offset along with the offset address when they want to use a bit array. Let me know if need any more help with this. Paul
-
I can't suggest anything else. The only reasons I know that the key would be invalid are: 1. The system date is wrong. 2. You're not using the current installer (4.936) 3. Your Name is not entered exactly as it is on your simMarket key page. Use copy and paste. 4. Your Email address is not entered exactly 5. The Key is not entered exactly 6. You've bought a key for WideFS6 (not 7) by mistake. Pete gets back on Tuesday if no one else has any ideas. Paul
-
Also, make sure the date on your PC is set correctly. Keys have a start date and won't work if your PC's date is set in the past.